What are `presenters` in Rails?
In the world of Rails, the primary goal is to maintain clean and manageable code. One of the challenges developers face is bloated models and controllers, especially when dealing with complex view logic. This is where the concept of `presenters` comes into play.
Presenters in Rails are a design pattern used to extract view-specific logic from models and controllers. Think of them as an intermediary layer between the model, which holds our data, and the view, which displays it. When you find yourself adding methods to a model purely for presentation purposes or cluttering views with complex logic, it might be time to consider a presenter.
For instance, if you have a `User` model and want to display the user’s full name in a view, instead of adding a `full_name` method to the model, you could use a presenter. The presenter would take an instance of the `User` model and have its own `full_name` method.
Here’s a basic example:
```ruby class UserPresenter def initialize(user) @user = user end def full_name "#{@user.first_name} #{@user.last_name}" end end ```
In your view, you would then create an instance of the `UserPresenter` and call the `full_name` method.
By using presenters, you adhere to the Single Responsibility Principle. Your models remain focused on business logic and data integrity, controllers on HTTP-specific tasks, and presenters handle the intricacies of presentation. It keeps views cleaner and more focused on layout and structure.
There are gems like `Draper` that provide additional functionality and make using presenters in Rails even smoother. However, the essence of the pattern is to simplify and segregate responsibilities, ensuring a maintainable and clean codebase.
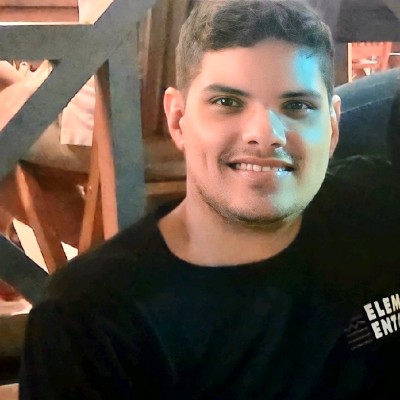
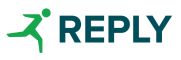