Ruby on Rails Tutorial: Understanding Rails Console and Debugging
Ruby on Rails is a versatile and powerful web application framework that has gained immense popularity over the years. While building web applications with Rails, developers often encounter bugs and issues that require debugging. This is where the Rails console comes to the rescue. In this tutorial, we will explore the Rails console and delve into effective debugging techniques to help you become a proficient Rails developer.
Table of Contents
1. What is the Rails Console?
1.1 Why Use the Rails Console?
The Rails console, often referred to as the rails console or simply console, is a command-line tool that provides an interactive environment for working with your Rails application. It allows you to interact with your application’s models, database, and various components in real-time.
So, why should you use the Rails console? Here are a few compelling reasons:
- Debugging: The console is a powerful debugging tool. It enables you to inspect the state of your application, test code snippets, and diagnose issues quickly.
- Data Manipulation: You can create, read, update, and delete records in your database directly from the console. This is incredibly useful for data-related tasks.
- Scripting: It can be used to write scripts that perform various tasks within your application, making it a versatile automation tool.
1.2 Accessing the Rails Console
To access the Rails console, open your terminal and navigate to your Rails application’s root directory. Then, simply type:
ruby rails console
or its shorthand:
ruby rails c
You’ll be greeted with an interactive shell where you can start executing commands and testing your application.
2. Basic Rails Console Commands
Now that you’re inside the Rails console, let’s explore some fundamental commands and techniques that will help you understand how it works.
2.1 Inspecting Variables
The Rails console allows you to inspect variables, objects, and the state of your application. For instance, to see the contents of a variable my_variable, you can simply type:
ruby my_variable
You can also use the puts or p methods to print the value of a variable or object:
ruby puts my_variable p my_object
This is particularly useful when you’re trying to understand the values of variables in your application’s code.
2.2 Running Queries
One of the most common use cases of the Rails console is running database queries. You can interact with your application’s models and query the database directly.
To retrieve all records from a specific model, use the all method:
ruby ModelName.all
For instance, if you have a User model:
ruby User.all
To find a specific record by its primary key (usually id), use the find method:
ruby ModelName.find(id)
For example:
ruby User.find(1)
2.3 Model Manipulation
The Rails console also allows you to create, update, and delete records in your database through your application’s models.
To create a new record, you can use the create method:
ruby ModelName.create(attribute1: value1, attribute2: value2)
For instance:
ruby User.create(name: 'John Doe', email: 'john@example.com')
To update a record, find it and then use the update method:
ruby user = User.find(1) user.update(name: 'Updated Name')
To delete a record, find it and use the destroy method:
ruby user = User.find(1) user.destroy
These basic console commands provide you with the groundwork for debugging and manipulating your Rails application.
3. Debugging Techniques with Rails Console
Debugging is an essential part of software development. The Rails console can be a valuable tool for diagnosing and fixing issues in your application. Let’s explore some debugging techniques.
3.1 Printing Debug Statements
One of the simplest debugging techniques is adding puts statements to your code to print information about the program’s execution. You can do the same in the Rails console to inspect variable values, control flow, and more.
For example, suppose you suspect an issue in a controller action:
ruby def some_action # ... puts "Debug point A" # ... puts "Debug point B" # ... end
You can call this action in the Rails console to see the debug messages:
ruby controller = SomeController.new controller.some_action
This helps you pinpoint where an issue might be occurring and what values your variables hold at different stages of execution.
3.2 Analyzing Database Queries
Debugging often involves identifying problems with database queries. The Rails console can help you analyze and optimize queries.
To see the SQL query generated by a specific ActiveRecord query, you can use the to_sql method:
ruby User.where(name: 'John Doe').to_sql
This will display the SQL query, which you can then use to analyze the database performance and correctness.
3.3 Fixing Associations
When dealing with ActiveRecord associations, you may encounter issues with data retrieval or manipulation. The console is a great place to test and fix these problems.
For instance, if you have a User model with a has_many association to Posts, you can use the console to access and manipulate posts related to a specific user:
ruby user = User.find(1) user.posts user.posts.create(title: 'New Post', content: 'Lorem ipsum')
This allows you to ensure that associations are working as expected and to troubleshoot any issues that arise.
3.4 Running Custom Methods
Sometimes, you may want to test custom methods or scripts within your Rails application. The Rails console is an ideal environment for this.
For example, if you have a custom method in your User model:
ruby class User < ApplicationRecord def custom_method # Custom logic here end end
You can test it in the console like so:
ruby user = User.find(1) user.custom_method
This helps you verify that your custom code behaves as intended and allows you to debug it effectively.
4. Advanced Debugging with Pry
While the Rails console is powerful on its own, you can supercharge your debugging experience with Pry, a powerful runtime developer console for Ruby. Pry provides enhanced features like code introspection, advanced navigation, and more.
4.1 Installing Pry
To use Pry in your Rails application, you need to add it to your Gemfile:
ruby gem 'pry'
Then, run:
ruby bundle install
4.2 Using Pry in Rails Console
After installing Pry, you can start using it in your Rails console sessions by invoking the binding.pry method:
ruby def some_method # ... binding.pry # ... end
When the code execution reaches the binding.pry line, your console will enter a Pry session, allowing you to interactively explore the code, evaluate expressions, and inspect variables.
Pry provides a more dynamic and interactive debugging experience, making it a valuable addition to your Rails debugging toolkit.
Conclusion
In this Ruby on Rails tutorial, we’ve explored the Rails console and learned essential debugging techniques to help you become a more proficient Rails developer. We covered the basics of the console, including variable inspection, running queries, and model manipulation. Additionally, we delved into advanced debugging using Pry, a powerful tool for debugging and exploration.
Mastering the Rails console and debugging techniques is crucial for building robust and maintainable Rails applications. With practice and experience, you’ll become adept at identifying and fixing issues, making you a more efficient and effective Rails developer. So, dive into your Rails projects with confidence, armed with the knowledge and tools to conquer any bug that comes your way. Happy coding!
Table of Contents
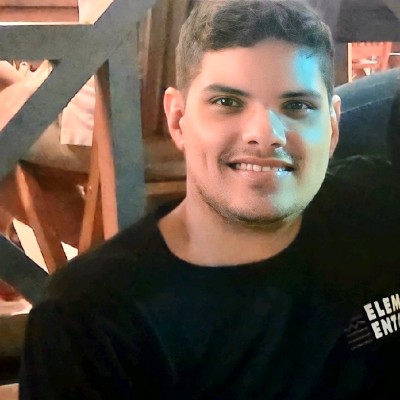
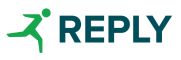