Ruby on Rails Tutorial: Understanding Rails Generators
Ruby on Rails, often referred to simply as Rails, is a powerful web development framework that follows the Model-View-Controller (MVC) architecture. One of the key features that makes Rails a developer’s favorite is its ability to automate many aspects of application development. And at the heart of this automation lie Rails generators.
Table of Contents
In this tutorial, we will dive deep into Rails generators, exploring what they are, how they work, and how you can harness their potential to streamline your development process. Whether you’re a beginner or an experienced Rails developer, understanding Rails generators is essential for building applications more efficiently.
1. What Are Rails Generators?
Rails generators are command-line tools that automate the creation of various components in a Ruby on Rails application. These components can include models, controllers, views, migrations, and more. Instead of writing code from scratch, you can use generators to generate boilerplate code and speed up your development process significantly.
2. Why Use Rails Generators?
Before we delve into the practical aspects of using Rails generators, let’s understand why they are so valuable in the world of web development.
- Efficiency: Rails generators save you time by generating the basic code structure for different parts of your application. This means you can focus on implementing your application’s specific logic rather than writing repetitive code.
- Consistency: Generators help maintain a consistent coding style and structure throughout your application. This is crucial for teamwork and when you revisit your codebase after some time.
- Reduced Errors: Since generators generate boilerplate code, there is less chance of introducing errors due to manual coding. This leads to a more stable and reliable application.
- Convention Over Configuration: Rails follows the principle of “Convention over Configuration.” This means that it makes assumptions about how things should be done, reducing the need for explicit configuration. Generators follow this principle, adhering to Rails conventions.
Now, let’s dive into some common Rails generators and see how they can be used effectively.
3. Common Rails Generators
3.1. Generating a Model
A model in Rails represents the data structure of your application. You can use the generate model command to create a model along with its corresponding database migration file. Here’s an example:
ruby rails generate model Article title:string body:text
In this example, we are generating a model called “Article” with two attributes: “title” and “body.” Rails will create the necessary files for this model, including the migration file for creating the “articles” table in the database.
3.2. Generating a Controller
Controllers handle the logic for processing requests and rendering views. You can generate a controller using the generate controller command:
ruby rails generate controller Articles
This command generates a controller named “Articles” along with associated view files and a test file. You can also specify actions to generate within the controller.
3.3. Generating a Scaffold
A scaffold generator is a powerful tool that generates a complete set of MVC components for a resource, including a model, controller, views, and routes. It’s a quick way to generate a fully functional CRUD (Create, Read, Update, Delete) interface for a resource. Here’s how you can use it:
ruby rails generate scaffold Product name:string price:decimal
This command generates a “Product” model, a “Products” controller, views for all CRUD actions, and sets up the corresponding routes.
3.4. Generating a Migration
Migrations in Rails are used to manage changes to your database schema. You can use the generate migration command to create a new migration file:
ruby rails generate migration AddDescriptionToProducts description:text
This command generates a migration file that adds a “description” column to the “products” table.
3.5. Generating Resources
The generate resource command is a convenient way to generate both a model and a controller for a resource:
ruby rails generate resource Comment body:text
This command generates a “Comment” model and a “Comments” controller, which are linked together as a resource.
4. Customizing Rails Generators
While Rails generators provide a lot of automation out of the box, you can also customize their behavior to suit your specific requirements. Rails allows you to create your own custom generators and modify existing ones.
4.1. Custom Generator Templates
You can create your own custom generator templates to enforce your team’s coding standards and practices. To do this, you need to define a custom generator template and then use it when generating new components. Here’s a high-level overview of the process:
- Create a directory for your custom templates, such as lib/templates.
- Organize the templates by generator type. For example, you can have a lib/templates/scaffold directory for scaffold templates.
- Create a custom template file with the same name as the generator you want to customize. For instance, if you want to customize the scaffold generator, create a file named scaffold_controller.rb in the appropriate directory.
- Customize the template to match your requirements.
When you generate a scaffold using the rails generate scaffold command, Rails will use your custom template instead of the default one.
4.2. Modifying Generator Behavior
You can also modify the behavior of existing generators by adding your own custom logic. To do this, you can create a custom Rails generator class and override the methods you want to customize. Here’s a basic example:
ruby # lib/generators/my_custom_generator/my_custom_generator_generator.rb require 'rails/generators/resource_helpers' module MyCustomGenerator class MyCustomGeneratorGenerator < Rails::Generators::NamedBase include Rails::Generators::ResourceHelpers def create_controller_file # Custom logic for generating controller end def create_view_files # Custom logic for generating views end end end
In this example, we’ve created a custom generator for generating controllers and views. By overriding the create_controller_file and create_view_files methods, you can add your own logic to these generator tasks.
5. Running Rails Generators
To run a Rails generator, you use the rails generate command, followed by the generator name and any necessary arguments and options. For example:
ruby rails generate model User name:string email:string
This command generates a User model with two attributes: name and email. Rails will create the migration file and model file for you.
Each generator has its own set of options and arguments, so be sure to consult the official Rails documentation for detailed information on each generator’s usage.
6. Cleaning Up Generated Code
While Rails generators are incredibly useful, they can also generate a lot of code that you may not need. It’s important to review and clean up the generated code regularly to ensure your application remains maintainable.
Here are some tips for cleaning up generated code:
- Delete Unused Files: If you generate a resource but don’t need all the default views or actions, feel free to delete the files you don’t need.
- Modify Generated Code: You can modify the generated code to better suit your application’s requirements. Just be cautious not to deviate too far from Rails conventions.
- Keep Migrations Organized: As your application evolves, you may generate and run multiple migrations. It’s a good practice to keep migrations organized and avoid redundancy.
Conclusion
Rails generators are powerful tools that can significantly boost your productivity as a Ruby on Rails developer. They automate the creation of various components, saving you time, ensuring consistency, and reducing errors. By customizing generators and understanding their behavior, you can tailor them to fit your specific project requirements.
As you continue to explore Ruby on Rails, make sure to leverage generators to streamline your development workflow. Whether you’re building a small personal project or a large-scale application, Rails generators will be your trusted companions on the journey to web development success. Happy coding!
Table of Contents
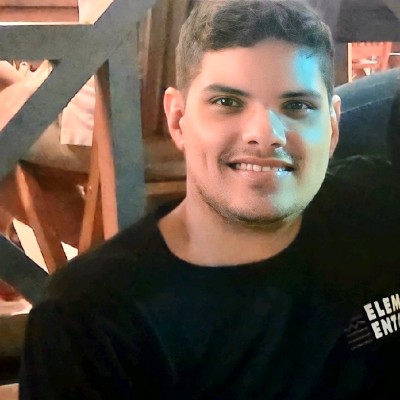
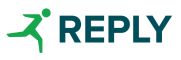