Ruby on Rails Tutorial: Understanding Rails Middleware
Ruby on Rails, often simply referred to as Rails, is a popular web application framework known for its simplicity and convention-over-configuration approach. It provides developers with a robust toolkit for building web applications quickly and efficiently. One of the key components that make Rails so powerful is its middleware.
Table of Contents
In this tutorial, we’ll take a deep dive into Rails middleware, explaining what it is, how it works, and why it’s essential for developing web applications. We’ll explore various middleware components and demonstrate their practical use with code samples and examples. By the end of this tutorial, you’ll have a solid understanding of how to leverage Rails middleware to enhance your web applications.
1. What Is Middleware?
Middleware, in the context of web development, is a software layer that sits between the web server and your application. Its primary purpose is to process and manipulate requests and responses as they flow through the application stack. In the case of Ruby on Rails, middleware components are an integral part of the request-response lifecycle.
Middleware provides a way to perform tasks such as authentication, logging, caching, and more, without cluttering your application’s core logic. It promotes the separation of concerns and modular design, making your codebase more maintainable and extensible.
2. The Rails Middleware Stack
Rails has a well-defined middleware stack that is executed for every incoming HTTP request. This stack consists of various middleware components, each responsible for specific tasks. Let’s take a closer look at some of the essential middleware in the Rails stack:
2.1. Rack Middleware
At the core of Rails middleware is Rack middleware. Rack is a web server interface used in Ruby web applications, and it defines a standard interface for handling HTTP requests and responses. Rails builds on top of Rack, making it the foundation for its middleware architecture.
Rack middleware is responsible for processing requests and responses at the lowest level. It sits between the web server (e.g., Puma, Unicorn, or WEBrick) and the Rails application. Each middleware component in the stack has the opportunity to inspect, modify, or terminate the request-response cycle.
Here’s an example of a simple Rack middleware:
ruby class CustomMiddleware def initialize(app) @app = app end def call(env) # Do something before the request reaches the application response = @app.call(env) # Do something after the request has been processed by the application response end end
In this example, CustomMiddleware intercepts the request and response, allowing you to add custom logic both before and after the Rails application processes the request.
2.2. Action Dispatch Middleware
Action Dispatch middleware is specific to Rails and provides higher-level functionality for handling web requests. It includes middleware for tasks like parameter parsing, session management, and routing. Some commonly used Action Dispatch middleware components include:
2.2.1. ParamsParser
ParamsParser middleware parses incoming request parameters and makes them available to your controllers. It supports various data formats, such as JSON and XML, making it easy to work with different request payloads.
2.2.2. Session
The Session middleware handles session management. It allows you to store and retrieve session data, making it possible to maintain user state across multiple requests.
2.2.3. Router
The Router middleware is responsible for routing incoming requests to the appropriate controller actions based on the URL and HTTP verb. It plays a crucial role in the Rails routing system.
2.3. Custom Middleware
Apart from the built-in middleware components, you can create custom middleware tailored to your application’s specific needs. Custom middleware can perform tasks like authentication, authorization, and logging. Let’s create a simple custom middleware for logging requests:
ruby class RequestLoggerMiddleware def initialize(app) @app = app end def call(env) request = Rack::Request.new(env) Rails.logger.info("Received #{request.request_method} request for #{request.url}") @app.call(env) end end
In this example, RequestLoggerMiddleware logs incoming requests, providing valuable information for debugging and monitoring.
3. Using Middleware in Rails
Now that we’ve covered the basics of middleware in Rails let’s see how to use them in a Rails application.
3.1. Configuring Middleware
Rails allows you to configure middleware in the config/application.rb file. The config.middleware method lets you add, remove, or reorder middleware components in the stack. Here’s an example of how to add the RequestLoggerMiddleware we created earlier:
ruby config.middleware.use RequestLoggerMiddleware
You can also use insert_before and insert_after to specify where your custom middleware should be placed in the stack relative to existing middleware.
3.2. Example Use Case: Authentication
One common use case for custom middleware in Rails is implementing authentication. Let’s create a simple authentication middleware that checks if a user is logged in and redirects them to the login page if not:
ruby class AuthenticationMiddleware def initialize(app) @app = app end def call(env) if user_not_authenticated?(env) redirect_to_login_page else @app.call(env) end end private def user_not_authenticated?(env) # Check if the user is not authenticated based on session or tokens # Return true if not authenticated, false otherwise end def redirect_to_login_page [302, { 'Location' => '/login' }, []] end end
In this example, AuthenticationMiddleware checks if the user is authenticated based on session data or tokens. If not, it redirects them to the login page.
3.3. Middleware Ordering Matters
The order of middleware in the stack matters, as they are executed in the sequence they are defined. For example, if you have a logging middleware that logs requests and a caching middleware that serves cached responses, you would want the logging middleware to come before the caching middleware.
ruby config.middleware.insert_before ActionDispatch::Static, RequestLoggerMiddleware
In this case, we’re inserting the RequestLoggerMiddleware before the ActionDispatch::Static middleware, ensuring that requests are logged before potentially serving cached responses.
3.4. Disabling Middleware
In some cases, you may need to disable or skip certain middleware for specific routes or actions. Rails provides a way to do this using skip or only in the config/application.rb file. For example, to skip the AuthenticationMiddleware for the /public route:
ruby config.middleware.skip AuthenticationMiddleware, only: '/public'
This configuration ensures that the authentication check is bypassed for requests to the /public route.
Conclusion
Rails middleware is a powerful mechanism that enhances the functionality and extensibility of your web applications. Understanding how middleware works and how to leverage it can significantly improve the maintainability and organization of your code.
In this tutorial, we’ve explored the fundamentals of Rails middleware, including Rack middleware and Action Dispatch middleware. We’ve also created custom middleware for logging and authentication and learned how to configure, order, and skip middleware in a Rails application.
As you continue to develop Rails applications, consider the role that middleware can play in simplifying complex tasks.
Table of Contents
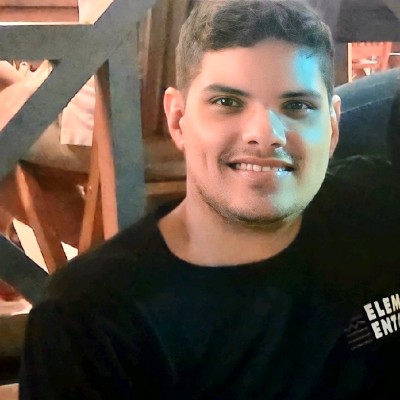
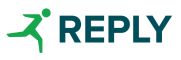