Ruby on Rails Tutorial: Understanding Rake and Task Automation
In the world of web development, efficiency is the name of the game. Developers are always on the lookout for tools and techniques that can help streamline their workflows, save time, and reduce errors. One such indispensable tool in the Ruby on Rails framework is Rake. In this tutorial, we’ll dive deep into Rake and explore how it can automate repetitive tasks and make your Ruby on Rails development experience smoother.
Table of Contents
1. Introduction to Rake
1.1. What is Rake?
Rake is a build automation tool written in Ruby, primarily used in Ruby on Rails applications. It’s similar to Makefiles in Unix systems but is designed specifically for Ruby projects. Rake is a command-line tool that allows you to define and run tasks to automate various parts of your development process.
1.2. Why Use Rake?
Rake is a powerful ally for Ruby on Rails developers for several reasons:
- Task Automation: Rake simplifies repetitive and complex tasks, making it easier to manage your application.
- Consistency: Tasks are defined in code, ensuring that everyone on your team performs them consistently.
- Extensibility: You can create custom tasks to fit your project’s unique needs.
- Integration: Rake seamlessly integrates with Rails and other Ruby gems.
2. Getting Started with Rake
2.1. Installation
Rake comes pre-installed with Ruby, so there’s no need to install it separately. You can check if Rake is available on your system by running the following command:
bash rake --version
2.2. Basic Rake Tasks
Let’s start with some basic Rake tasks. In your Rails application directory, you can run the default Rake task with:
bash rake
This will list all available Rake tasks for your project. By default, Rails generates some essential tasks for database management, testing, and more.
To run a specific task, use the following command:
bash rake <task_name>
For instance, to migrate your database, use:
bash rake db:migrate
3. Writing Your Own Rake Tasks
3.1. The Rakefile
To create custom Rake tasks, you’ll need to work with the Rakefile. This file, usually located in your Rails application’s root directory, is where you define and organize your tasks. If your application doesn’t have a Rakefile, you can create one.
3.2. Defining Tasks
Defining a task in Rake is straightforward. You use the task method and provide a symbol as the task name, followed by a block of code that represents the task’s functionality. Here’s a simple example:
ruby task :greet do puts "Hello, Rake!" end
You can run this task using rake greet. It will output “Hello, Rake!” to the console.
3.3. Running Tasks
Rake tasks can also take arguments and options. For instance, suppose you want to pass a name to your greet task:
ruby task :greet, [:name] do |t, args| puts "Hello, #{args[:name] || 'Rake'}!" end
Now, you can run rake greet[“Alice”], and it will greet Alice. If you omit the name, it defaults to “Rake.”
4. Common Use Cases for Rake
4.1. Database Management
One of the most common use cases for Rake in Ruby on Rails is database management. Rails provides several built-in tasks to help you migrate, seed, and reset your database.
To create and migrate the database:
bash rake db:create rake db:migrate
To populate the database with seed data:
bash rake db:seed
To reset the database:
bash rake db:reset
These tasks help you keep your database schema up-to-date and ensure that your development and production databases are in sync.
4.2. Asset Compilation
In addition to database tasks, Rake can be used for asset compilation. When working with assets like JavaScript and CSS files, you can use Rake to precompile and manage them efficiently.
To precompile assets for production:
bash rake assets:precompile
This task generates minified and concatenated versions of your assets, which can significantly improve your application’s performance in production.
4.3. Testing Automation
Automating tests is another area where Rake shines. Rails developers commonly use Rake tasks to run tests, generate code coverage reports, and manage testing-related tasks.
To run your test suite:
bash rake test
To generate a code coverage report:
bash rake coverage
By creating custom Rake tasks, you can extend and enhance your testing automation to suit your project’s specific needs.
5. Advanced Rake Techniques
5.1. Namespaces
Namespaces help organize your Rake tasks into logical groups. For example, if you have multiple database-related tasks, you can group them under a db namespace:
ruby namespace :db do desc "Migrate the database" task :migrate do # ... end desc "Seed the database" task :seed do # ... end end
This allows you to run tasks like rake db:migrate and rake db:seed, keeping your Rake tasks organized and more readable.
5.2. Dependencies
You can specify task dependencies in Rake. This means that one task can depend on the successful completion of another task. For instance, you might want to run database migrations before seeding the database:
ruby task :seed => :migrate do # This task will run after the :migrate task end
Now, when you run rake seed, Rake will first execute the migrate task and then proceed with seed.
5.3. Task Arguments
Tasks can accept arguments, making them more versatile. For example, you can create a task to generate a report based on a specific date:
ruby task :generate_report, [:date] do |t, args| report_date = args[:date] || Date.today # Generate the report based on the specified date end
You can then run this task with rake generate_report[2023-09-26] to generate a report for a specific date.
6. Rake in Real Projects
Example 1: Database Backup Task
Let’s consider a real-world example. You want to create a Rake task to backup your production database regularly. Here’s how you might define such a task:
ruby task :backup_database do timestamp = Time.now.strftime("%Y%m%d%H%M%S") backup_filename = "backup_#{timestamp}.sql" `pg_dump -U your_db_user your_production_db > #{backup_filename}` end
You can run this task with rake backup_database, and it will create a backup of your production database with a timestamp in the filename.
Example 2: Automated Testing Suite
In a large Rails project, you might have a suite of Rake tasks for various testing scenarios. Here’s an example of how you can structure your testing tasks:
ruby namespace :test do task :unit do # Run unit tests end task :integration do # Run integration tests end task :coverage => [:unit, :integration] do # Generate code coverage report end end
With this setup, you can run all unit tests with rake test:unit, all integration tests with rake test:integration, and generate a code coverage report for both with rake test:coverage.
7. Best Practices and Tips
7.1. Organizing Your Rake Tasks
As your project grows, you may accumulate numerous Rake tasks. To maintain code readability and organization, consider placing tasks related to a specific area (e.g., database, testing) into their respective namespaces. Additionally, document your tasks with desc statements to provide clear descriptions for each task.
7.2. Error Handling
When writing Rake tasks, be sure to implement error handling to gracefully handle failures. You can use begin and rescue blocks to capture and handle exceptions, ensuring that tasks do not leave your application in an inconsistent state.
7.3. Documentation
Documenting your Rake tasks is essential, especially when working with a team. Include comments explaining the purpose and usage of each task in your Rakefile. This helps team members understand the tasks and use them effectively.
Conclusion
In the world of Ruby on Rails development, Rake is a powerful tool that can significantly improve your workflow by automating repetitive tasks and enhancing project organization. Whether you’re managing databases, compiling assets, or automating testing, Rake provides the flexibility and extensibility needed to make your development process smoother and more efficient.
By mastering Rake and incorporating it into your Rails projects, you’ll not only save time but also ensure consistency and reliability in your development tasks. So, don’t hesitate to explore Rake’s capabilities and start automating your Ruby on Rails projects today. Happy coding!
Table of Contents
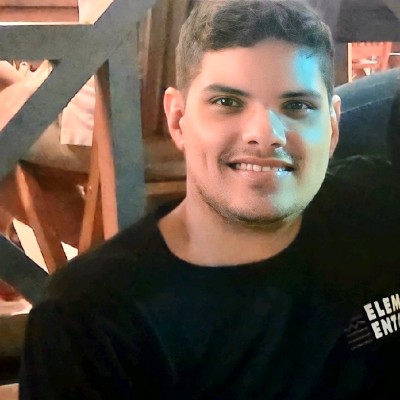
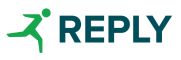