How to create relationships between Rails models?
In Rails, relationships between models, often reflecting underlying database relations, are vital for modeling real-world scenarios. Using the ActiveRecord ORM, Rails provides a concise and expressive syntax to establish these connections:
- One-to-Many Relationship: This is the most common relationship, where one record in a table can be related to multiple records in another table. For instance, if one `User` can have many `Posts`:
– In the `User` model:
```ruby has_many :posts ```
– In the `Post` model:
```ruby belongs_to :user ```
- Many-to-Many Relationship: Sometimes, you need a relation where records in Table A can have multiple associations in Table B and vice-versa. Rails achieves this using a join table. Let’s say `Students` and `Courses`:
– First, you’ll have a join model, perhaps `Enrollment`.
– In the `Student` model:
```ruby has_many :enrollments has_many :courses, through: :enrollments ```
– In the `Course` model:
```ruby has_many :enrollments has_many :students, through: :enrollments ```
- One-to-One Relationship: This is where one record in a table directly relates to one record in another table. For instance, if a `User` has one `Profile`:
– In the `User` model:
```ruby has_one :profile ```
– In the `Profile` model:
```ruby belongs_to :user ```
- Polymorphic Associations: These allow a model to belong to multiple other types of models. Imagine comments that can belong to posts, images, or videos. You’d use a `commentable` interface for this:
– In the `Comment` model:
```ruby belongs_to :commentable, polymorphic: true ```
– Then in models like `Post`, `Image`, or `Video`:
```ruby has_many :comments, as: :commentable ```
Rails’ ActiveRecord provides an elegant way to represent and manipulate database relationships in an object-oriented manner. This abstraction not only keeps your code DRY (Don’t Repeat Yourself) but also ensures consistency and clarity in how data models interact. As you design your Rails application, understanding these relationships is fundamental to capturing the essence and nuances of the problem you’re addressing.
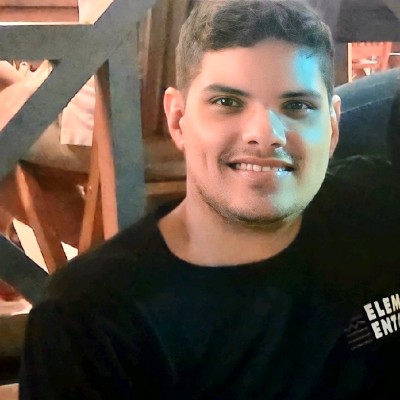
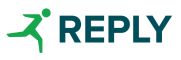