What are routes and how to use them in Ruby on Rails?
In the Ruby on Rails framework, routes determine how incoming requests to a web application are dispatched to a specific controller action. Essentially, they define the URL patterns that the application should respond to and how. Here’s a breakdown of Rails routes and their utilization:
- Basics of Routes: Every Rails app has a file named `routes.rb` located in the `config` directory. This file contains all the routing declarations for the application. The router interprets the URL and dispatches the request to the corresponding controller action.
- Defining Routes: In `routes.rb`, you can specify routes in various ways:
– Simple Route: Connects a URL to a specific controller action.
```ruby get 'welcome/index', to: 'welcome#index' ```
This means that a GET request to `/welcome/index` will be directed to the `index` action of the `Welcome` controller.
– Resourceful Route: Automatically generates multiple standard routes for a given resource.
```ruby resources :articles ```
This single line creates several routes for CRUD operations on `articles`, such as index, show, new, edit, create, update, and destroy.
- Route Parameters: Routes can also capture parameters from the URL. For instance:
```ruby get 'articles/:id', to: 'articles#show' ```
In this case, `:id` is a dynamic segment, capturing a section of the URL to be used as a parameter. If a user accesses `/articles/5`, the `show` action of the `Articles` controller will be invoked with `params[:id]` set to `5`.
- Named Routes: These provide a way to generate a URL or path. For example:
```ruby get 'welcome/index', to: 'welcome#index', as: 'welcome' ```
Now, `welcome_path` will return `/welcome/index`, and you can use it in views and controllers.
- Root Route: It’s common to define a root route, which specifies the default page the app loads when accessing the base URL.
```ruby root 'welcome#index' ```
Routes are the bridge between URLs and the logic defined in your controllers. They are crucial for the user experience, helping shape the structure and navigation of your Rails application. When defining routes, always strive for clarity and consistency, ensuring that your URLs are intuitive and reflective of your application’s structure.
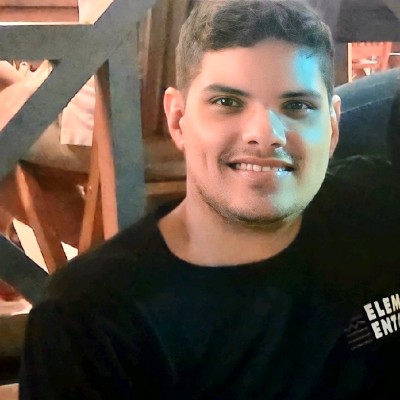
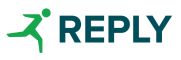