Ruby on Rails Tutorial: Understanding Routing and Controllers
Ruby on Rails is a powerful web development framework known for its simplicity, convention-over-configuration approach, and rapid development capabilities. One of the key components of Rails is its routing system and controllers, which play a crucial role in handling HTTP requests and directing them to the appropriate actions within an application.
In this tutorial, we’ll explore the fundamental concepts behind routing and controllers in Ruby on Rails. We’ll delve into the process of defining routes, understanding RESTful routing, and working with controllers to handle requests and deliver responses. By the end, you’ll have a solid understanding of how routing and controllers work together to build dynamic and interactive web applications.
What is Routing?
Routing is the process of mapping URLs (Uniform Resource Locators) to specific actions within a web application. In Ruby on Rails, the routing system determines which controller and action should be invoked based on the incoming request’s URL and HTTP verb (GET, POST, PUT, DELETE, etc.).
By defining routes, we establish the connection between URLs and the corresponding controller actions, enabling our application to respond to client requests appropriately.
The Basics of Routes
1. Defining Routes
In Ruby on Rails, routes are defined in the config/routes.rb file. This file serves as the central hub for all route definitions within your application. The routes file uses a DSL (Domain-Specific Language) that provides a concise and expressive way to define routes.
Here’s an example of a simple route definition:
ruby # config/routes.rb Rails.application.routes.draw do get 'welcome/index' root 'welcome#index' end
In this example, we define two routes: one for the URL welcome/index, and another for the root URL. The get method maps the specified URL to the appropriate controller action.
2. RESTful Routing
RESTful routing is a convention in Ruby on Rails that encourages designing applications around a set of well-defined, standardized actions. These actions map to the basic CRUD operations: Create, Read, Update, and Delete.
Here’s an example of RESTful routing:
ruby # config/routes.rb Rails.application.routes.draw do resources :articles end
By using the resources method, Rails automatically generates a set of routes for handling CRUD operations on the articles resource. These routes include index, new, create, show, edit, update, and destroy.
3. Resourceful Routing
Resourceful routing allows you to define custom routes for a specific resource while keeping the RESTful nature intact. It provides flexibility to add additional routes specific to a resource.
Here’s an example of resourceful routing:
ruby # config/routes.rb Rails.application.routes.draw do resources :articles do get 'search', on: :collection patch 'publish', on: :member end end
In this example, we define two additional routes for the articles resource. The get ‘search’ route is defined on the collection, meaning it operates on the entire collection of articles. The patch ‘publish’ route, on the other hand, is defined on a member, operating on a specific article.
Working with Controllers
1. Controller Basics
Controllers in Ruby on Rails act as intermediaries between the models and views. They handle incoming requests, interact with the corresponding model layer, and render appropriate responses using views.
To generate a controller, you can use the following command:
shell rails generate controller ControllerName
2. Action Methods
Action methods within a controller represent the individual actions that can be performed on a resource. These methods are responsible for executing the logic associated with a particular request and preparing the data to be displayed in the corresponding view.
Here’s an example of an action method:
ruby # app/controllers/articles_controller.rb class ArticlesController < ApplicationController def index @articles = Article.all end end
In this example, the index action retrieves all articles from the database and assigns them to an instance variable @articles, which can be accessed in the corresponding view.
3. Filters and Callbacks
Rails provides filters and callbacks that allow you to execute code before, after, or around specific actions within a controller. These callbacks provide a way to add additional behavior, such as authentication, authorization, and data manipulation.
Here’s an example of a before_action filter:
ruby # app/controllers/articles_controller.rb class ArticlesController < ApplicationController before_action :authenticate_user def index @articles = Article.all end private def authenticate_user # Logic to authenticate the user end end
In this example, the before_action filter is used to authenticate the user before executing the index action.
Building a Simple Application
1. Setting Up the Project
To get started, make sure you have Ruby and Rails installed on your system. You can install them by following the instructions provided in the official Ruby on Rails documentation.
Once you have Rails installed, create a new Rails application by running the following command:
shell rails new blog
2. Creating Routes
In the config/routes.rb file, define the routes for your application. For example:
ruby # config/routes.rb Rails.application.routes.draw do root 'articles#index' resources :articles End
In this example, we set the root route to the index action of the articles controller and define the RESTful routes for the articles resource.
3. Generating a Controller
Generate a controller for the articles resource using the following command:
shell rails generate controller Articles
This command generates a controller file (articles_controller.rb) and related view files.
4. Handling Requests and Rendering Views
In the generated articles_controller.rb file, add the necessary actions to handle requests and render views. For example:
ruby # app/controllers/articles_controller.rb class ArticlesController < ApplicationController def index @articles = Article.all end def show @article = Article.find(params[:id]) end def new @article = Article.new end def create @article = Article.new(article_params) if @article.save redirect_to @article else render 'new' end end private def article_params params.require(:article).permit(:title, :content) end end
In this example, we define the index, show, new, and create actions. The index action retrieves all articles, the show action finds a specific article by its ID, the new action initializes a new article object, and the create action saves a new article to the database.
Advanced Routing Techniques
1. Named Routes
Named routes provide a way to assign custom names to routes, allowing for easier navigation within your application and cleaner code. To define a named route, use the as option.
Here’s an example:
ruby # config/routes.rb Rails.application.routes.draw do get 'about', to: 'pages#about', as: 'about_us' end
In this example, the about route is assigned the name about_us. This allows you to use about_us_path or about_us_url in your views and controllers to generate URLs
2. Route Constraints
Route constraints allow you to add conditions to your routes based on specific requirements. These constraints can be regular expressions, custom classes, or lambdas.
Here’s an example of using a regular expression constraint:
ruby # config/routes.rb Rails.application.routes.draw do get 'articles/:id', to: 'articles#show', constraints: { id: /\d+/ } end
In this example, the route will only match if the id parameter is a numeric value.
3. Nested Resources
Nested resources allow you to express relationships between different resources in your application. By nesting resources, you can represent more complex associations and generate URLs that reflect the hierarchical structure.
Here’s an example:
ruby # config/routes.rb Rails.application.routes.draw do resources :articles do resources :comments end end
In this example, the comments resource is nested within the articles resource. This generates routes such as /articles/:article_id/comments, enabling you to create, read, update, and delete comments associated with a specific article.
Conclusion
In this tutorial, we’ve explored the ins and outs of routing and controllers in Ruby on Rails. We’ve covered the basics of defining routes, RESTful routing, and resourceful routing. We’ve also discussed how controllers handle requests, execute action methods, and employ filters and callbacks.
By mastering routing and controllers, you’ll have the foundation needed to build robust and dynamic web applications using Ruby on Rails. So go ahead, dive in, and start building amazing applications with confidence!
Table of Contents
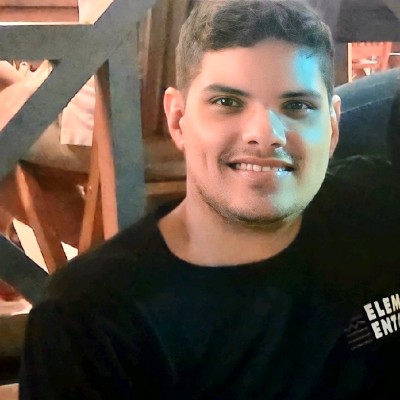
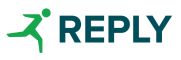