Ruby on Rails Tutorial: Understanding Routing and RESTful Architecture
Ruby on Rails (RoR) is a powerful and widely used web development framework known for its simplicity and convention-over-configuration philosophy. A core component of building web applications with RoR is understanding routing and the principles of RESTful architecture. In this comprehensive tutorial, we’ll delve into the world of routing and RESTful architecture in Ruby on Rails, exploring key concepts, best practices, and code samples to help you build robust and maintainable web applications.
Table of Contents
1. Introduction to Routing
1.1. What Is Routing?
In the context of web development, routing refers to the process of determining how an incoming HTTP request should be handled by the application. It serves as a map that connects URLs to specific actions or controllers within your Ruby on Rails application.
Routing defines the relationship between URLs and the code that should execute when a user visits a particular URL. For example, when a user goes to “https://example.com/articles,” routing helps determine which Ruby on Rails controller and action should handle this request.
1.2. The Importance of Routing
Effective routing is essential for several reasons:
- Clean and Intuitive URLs: Routing allows you to create clean, human-readable URLs for your web application. This enhances the user experience and improves SEO.
- Logical Code Structure: Routing helps organize your code by mapping URLs to specific controller actions. This separation of concerns makes your codebase more maintainable and scalable.
- RESTful Architecture: Routing plays a crucial role in implementing RESTful architecture, which is a standard approach to building web APIs and applications. RESTful architecture simplifies complex operations and promotes best practices.
Now that we understand the basics of routing, let’s dive deeper into the concept of RESTful architecture.
2. Understanding RESTful Architecture
2.1. What Is REST?
REST, which stands for Representational State Transfer, is a set of architectural principles and constraints for designing networked applications. It was introduced by Roy Fielding in his doctoral dissertation in 2000 and has since become a widely adopted approach for building web services and APIs.
RESTful architecture is characterized by several key principles:
- Statelessness: Each HTTP request from a client to a server must contain all the information needed to understand and process the request. The server should not rely on any previous requests.
- Client-Server Architecture: REST separates the client (the user interface or user agent) from the server (the application that stores and processes data). This separation allows for better scalability and flexibility.
- Uniform Interface: RESTful APIs have a consistent and uniform set of rules for interacting with resources. These rules include using standard HTTP methods (GET, POST, PUT, DELETE) and using URLs to identify resources.
- Resource-Based: In REST, everything is treated as a resource, and resources are identified by URLs. Resources can represent objects, data, or entities in the application.
- CRUD Operations: RESTful architecture often maps CRUD (Create, Read, Update, Delete) operations to HTTP methods. For example, a POST request may create a new resource, a GET request may retrieve a resource, a PUT request may update a resource, and a DELETE request may delete a resource.
2.2. The Six RESTful Actions
In RESTful architecture, there are six primary actions that can be performed on a resource:
- Index (GET): Retrieve a list of all resources.
- Show (GET): Retrieve a single resource by its unique identifier.
- New (GET): Display a form to create a new resource.
- Create (POST): Create a new resource based on the data submitted in a form.
- Edit (GET): Display a form to edit an existing resource.
- Update (PUT/PATCH): Update an existing resource based on the data submitted in a form.
- Destroy (DELETE): Delete an existing resource.
These actions form the basis of RESTful routing in Ruby on Rails. When you define routes for your application, you map URLs to these actions, allowing your controllers to respond accordingly.
3. Configuring Routes in Ruby on Rails
3.1. The routes.rb File
In Ruby on Rails, route configuration is typically done in the config/routes.rb file. This file serves as the central hub for defining how incoming requests should be routed to controllers and actions.
Let’s take a look at a basic routes.rb file:
ruby Rails.application.routes.draw do # Define routes here end
3.2. Basic Route Configuration
To define a basic route in Ruby on Rails, you can use the get method within the draw block. Here’s an example:
ruby Rails.application.routes.draw do get '/welcome', to: 'pages#home' end
In this example, when a user visits the URL /welcome, the PagesController’s home action will be executed. This is a simple and common way to configure routes in RoR.
3.3. Named Routes
Named routes are a way to give a route a specific name, making it easier to reference in your application. You can create named routes using the as option. For instance:
ruby Rails.application.routes.draw do get '/about', to: 'pages#about', as: 'about_page' end
Now, you can reference this route as about_page_path or about_page_url in your views and controllers.
3.4. Dynamic Segments
In many cases, your URLs will include dynamic segments, such as an article’s ID or a username. You can define dynamic segments in your routes using colon syntax. Here’s an example:
ruby Rails.application.routes.draw do get '/articles/:id', to: 'articles#show', as: 'article' end
With this configuration, visiting a URL like /articles/1 will call the show action of the ArticlesController, and the params[:id] will be set to 1.
4. RESTful Routing in Ruby on Rails
4.1. Resourceful Routes
Ruby on Rails simplifies the process of defining RESTful routes with the resources method. The resources method allows you to declare routes for a resource in a single line. Here’s how you can define routes for an Article resource:
ruby Rails.application.routes.draw do resources :articles end
This single line of code generates all the routes needed for a RESTful resource, including index, show, new, create, edit, update, and destroy routes.
4.2. Using Resources
By declaring resourceful routes, Ruby on Rails adheres to RESTful conventions. For example, the GET /articles route maps to the index action, and the GET /articles/1 route maps to the show action.
To see the generated routes for a resource, you can run the rails routes command in your terminal. It will display a list of all the routes generated by the resources method.
4.3. Custom Routes
While resourceful routes are convenient, there are cases where you need to define custom routes to handle specific actions. You can do this by adding custom route definitions after the resources declaration. For example:
ruby Rails.application.routes.draw do resources :articles get '/articles/:id/publish', to: 'articles#publish', as: 'publish_article' end
In this example, a custom route GET /articles/1/publish is defined to handle the publish action of the ArticlesController. You can reference this route as publish_article_path or publish_article_url.
5. Code Samples and Best Practices
5.1. Sample Routes
Let’s take a closer look at how resourceful routes map to controller actions:
- Index (GET /articles): Lists all articles – ArticlesController#index
- Show (GET /articles/1): Displays a specific article – ArticlesController#show
- New (GET /articles/new): Displays a form to create a new article – ArticlesController#new
- Create (POST /articles): Creates a new article – ArticlesController#create
- Edit (GET /articles/1/edit): Displays a form to edit an article – ArticlesController#edit
- Update (PUT/PATCH /articles/1): Updates an article – ArticlesController#update
- Destroy (DELETE /articles/1): Deletes an article – ArticlesController#destroy
5.2. RESTful Controller Actions
When defining controller actions for your resources, it’s essential to follow RESTful conventions. Here’s a typical controller structure for managing articles:
ruby class ArticlesController < ApplicationController def index # List all articles end def show # Display a specific article end def new # Display a form to create a new article end def create # Create a new article end def edit # Display a form to edit an article end def update # Update an article end def destroy # Delete an article end end
Following this structure makes your code more organized and easier to maintain. Each action corresponds to one of the six RESTful actions.
5.3. Nesting Resources
In more complex applications, you might need to nest resources. Nesting allows you to represent the hierarchical relationship between different resources. For example, if you have an Article resource that belongs to a User, you can nest the routes as follows:
ruby resources :users do resources :articles end
This nesting creates routes like /users/1/articles and /users/1/articles/2, where the first URL lists all articles belonging to a user, and the second URL displays a specific article for that user.
Conclusion
Mastering routing and RESTful architecture in Ruby on Rails is essential for building scalable, maintainable, and user-friendly web applications. By understanding the principles of routing and adhering to RESTful conventions, you can streamline your development process and create applications that are intuitive for both users and developers.
In this tutorial, we’ve covered the fundamentals of routing, explored RESTful architecture, and learned how to configure routes in Ruby on Rails. We’ve also discussed best practices for defining controller actions and introduced the concept of nesting resources. Armed with this knowledge, you’re well on your way to becoming a proficient Ruby on Rails developer.
Remember that practice makes perfect, so continue building and refining your Rails applications, and don’t hesitate to consult the official Rails documentation and community resources for further guidance. Happy coding!
Table of Contents
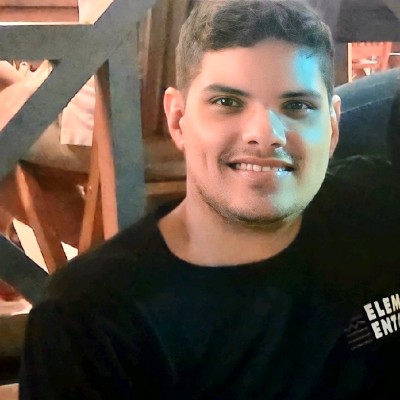
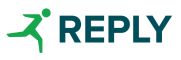