How to Use Ruby Functions for Scheduling and Cron Jobs
In the world of software development, automation is key to improving efficiency and reducing manual effort. One powerful way to automate repetitive tasks is by using cron jobs, and when it comes to handling cron jobs in Ruby, you have a range of tools and libraries at your disposal. In this comprehensive guide, we’ll delve into the world of scheduling and cron jobs in Ruby, exploring how you can harness the power of Ruby functions to automate your tasks effectively.
Table of Contents
1. Introduction to Cron Jobs
1.1. Understanding Cron Syntax
Cron jobs are time-based job schedulers in Unix-like operating systems. They follow a specific syntax for scheduling tasks at predefined intervals. The cron syntax consists of five fields:
- Minute (0 – 59)
- Hour (0 – 23)
- Day of the Month (1 – 31)
- Month (1 – 12)
- Day of the Week (0 – 7) (Sunday is both 0 and 7)
For example, if you want to schedule a job to run every day at 2:30 PM, the cron syntax would be:
30 14 * * *
1.2. Common Use Cases
Cron jobs are versatile and find applications in various scenarios, including:
- Data Backup: Automate regular backups of databases and files.
- Data Cleanup: Remove temporary files or outdated records.
- Email Notifications: Send daily reports or notifications.
- Periodic Tasks: Execute tasks like generating reports, updating caches, or running maintenance scripts.
Now that we understand the basics of cron jobs, let’s explore how Ruby can simplify the process.
2. Using the ‘rufus-scheduler’ Gem
Rufus-scheduler is a popular gem for scheduling jobs in Ruby. It provides a straightforward API for scheduling tasks and recurring jobs.
2.1. Installation
To get started, you’ll need to install the ‘rufus-scheduler’ gem. Add it to your Gemfile:
ruby gem 'rufus-scheduler'
And then run:
bash bundle install
2.2. Basic Scheduling
Here’s a basic example of scheduling a job to run every day at a specific time:
ruby require 'rufus-scheduler' scheduler = Rufus::Scheduler.new scheduler.at '14:30' do # Your task code here puts "Scheduled task executed at #{Time.now}" end
In this example, we create a scheduler instance and use the at method to schedule a task at 2:30 PM. You can replace the puts statement with your task logic.
2.3. Recurring Jobs
Rufus-scheduler also supports recurring jobs. For instance, to run a job every hour, you can do the following:
ruby scheduler.every '1h' do # Your hourly task code here puts "Hourly task executed at #{Time.now}" end
2.4. Error Handling
It’s essential to handle errors in your scheduled tasks. Rufus-scheduler allows you to add error handling easily:
ruby scheduler.at '14:30' do begin # Your task code here puts "Scheduled task executed at #{Time.now}" rescue StandardError => e puts "Error in scheduled task: #{e.message}" end end
By wrapping your task code in a begin and rescue block, you can capture and log any exceptions that may occur during execution.
3. Leveraging the ‘whenever’ Gem
The ‘whenever’ gem simplifies the process of working with cron jobs in Ruby. It provides a higher-level DSL (Domain-Specific Language) for creating and managing cron jobs.
3.1. Installing ‘whenever’
To start using ‘whenever,’ you need to install the gem:
bash gem install whenever
3.2. Creating Cron Jobs with ‘whenever’
‘Whenever’ allows you to define cron jobs using a clear and readable syntax in your Ruby codebase. First, generate a ‘schedule.rb’ file with the following command:
bash wheneverize .
Now, you can define your cron jobs in ‘config/schedule.rb’:
ruby every 1.day, at: '2:30 pm' do runner 'YourModel.your_method' end
In this example, we schedule a job to run every day at 2:30 PM, invoking a method your_method in the model YourModel.
3.3. Customizing Schedules
‘Whenever’ provides a wide range of options for customizing schedules. You can use the :at option to specify the exact time, :every to set the frequency, and much more. For example, to run a job every hour, you can do the following:
ruby every 1.hour do runner 'YourModel.hourly_method' end
The ‘whenever’ gem simplifies the process of managing cron jobs and makes your code more readable and maintainable.
4. Scheduling Tasks with System Cron
In addition to using Ruby gems like ‘rufus-scheduler’ and ‘whenever,’ you can also schedule tasks using the system’s built-in cron service. Ruby can interact with the system cron by executing shell commands.
4.1. The ‘system’ Command
The simplest way to create a cron job from Ruby is by using the system command. For instance, to schedule a Ruby script to run every day at 3:45 PM, you can do the following:
ruby # Schedule a Ruby script with system cron system('whenever -w') # Update the 'whenever' cron jobs (optional) system('crontab -l | { cat; echo "45 15 * * * /usr/bin/ruby /path/to/your_script.rb"; } | crontab -')
The first system command updates the ‘whenever’ cron jobs if you are using the ‘whenever’ gem, and the second command appends the new cron job to the existing list.
4.2. Managing Cron Jobs with Ruby
You can also use Ruby to manage and manipulate cron jobs directly. The ‘whenever’ gem, for example, provides a Ruby API for interacting with the system’s cron.
Here’s an example of how to add a cron job using ‘whenever’ Ruby API:
ruby require 'whenever' # Initialize 'whenever' with your schedule file every_day_job = Whenever::Job.new( :every => 1.day, :at => '3:45 pm', :command => '/usr/bin/ruby /path/to/your_script.rb' ) # Add the job to the schedule Whenever.cron.unshift every_day_job Whenever.write_crontab
This code defines a daily job and adds it to the ‘whenever’ schedule.
4.3. Security Considerations
When using system cron, be cautious about security. Ensure that the Ruby scripts you execute are secure and do not expose vulnerabilities. Additionally, limit access to your cron configuration files to authorized users only.
5. Advanced Techniques
5.1. Handling Long-Running Tasks
Some tasks scheduled with cron may take a significant amount of time to complete. To prevent overlapping instances of the same task, you can use file locks or process checks in your Ruby code.
Here’s an example of using a file lock with Ruby:
ruby # Ensure only one instance of the task is running at a time lockfile = '/tmp/my_cron_task.lock' if File.exist?(lockfile) puts 'Task is already running.' else File.open(lockfile, 'w') do |file| file.puts Process.pid end # Your task code here File.delete(lockfile) end
5.2. Logging and Monitoring
To track the execution of your scheduled tasks, implement proper logging and monitoring. Ruby provides robust logging libraries like ‘Logger’ that you can use to record task execution details.
ruby require 'logger' log = Logger.new('cron.log') begin # Your task code here log.info('Task executed successfully.') rescue StandardError => e log.error("Error in task: #{e.message}") end
With logging in place, you can easily review the task’s history and troubleshoot any issues that arise.
5.3. Testing Cron Jobs
Testing cron jobs can be challenging since they are time-based and often run in a production environment. However, you can simulate cron job execution in your development environment by creating wrapper scripts or using tools like ‘Timecop’ to manipulate time.
Additionally, you can use automated testing frameworks to test the functionality of the tasks that your cron jobs execute.
Conclusion
Using Ruby functions for scheduling and managing cron jobs is a powerful way to automate recurring tasks and improve your workflow. Whether you prefer using gems like ‘rufus-scheduler’ and ‘whenever’ or interacting directly with the system’s cron service, Ruby provides flexibility and control over your scheduled tasks.
Remember to handle errors gracefully, implement logging and monitoring, and consider security best practices when working with cron jobs in Ruby. With these techniques in your toolkit, you can automate tasks efficiently and free up time for more critical aspects of your development work.
Table of Contents
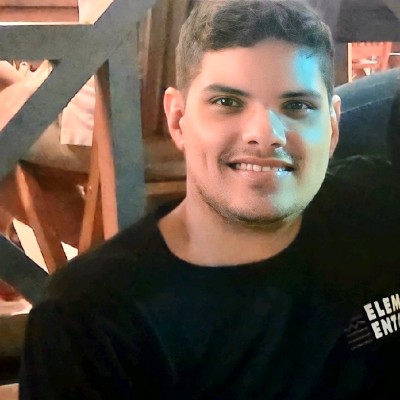
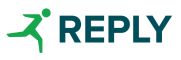