What are Rails scopes?
Rails scopes are a powerful feature provided by the ActiveRecord framework to create reusable and chainable SQL queries. Essentially, they offer a method to define commonly used queries, allowing cleaner and more maintainable code.
A scope is defined in the model and can be used just like any class method. The primary advantage of using scopes over class methods is their ability to chain together seamlessly, making complex queries more readable.
Here’s a simple example to illustrate: Imagine you have a `Product` model, and you frequently need to filter products that are active and priced above a certain threshold. Instead of writing these queries repeatedly, you can define scopes for them:
```ruby class Product < ApplicationRecord scope :active, -> { where(active: true) } scope :priced_above, ->(amount) { where("price > ?", amount) } end ```
With these scopes in place, querying becomes more intuitive:
```ruby Product.active.priced_above(100) ```
This would return all active products with a price greater than 100.
A few key points about scopes:
- Chainable: As demonstrated, scopes can be chained together. If one scope doesn’t return any records, subsequent scopes won’t break the chain—they simply return an empty result set.
- Lambdas: Scopes use lambda functions to ensure that the code inside the scope is executed every time it’s called, allowing dynamic parameters.
- Default Scopes: While Rails does offer the ability to define a `default_scope`, it’s advisable to use this feature cautiously. A default scope applies to all queries on the model, which can lead to unexpected results if not managed properly.
Scopes in Rails are a way to keep your database queries organized, readable, and maintainable. They encapsulate complex query logic and offer a fluid API to interact with the data layer of your Rails application.
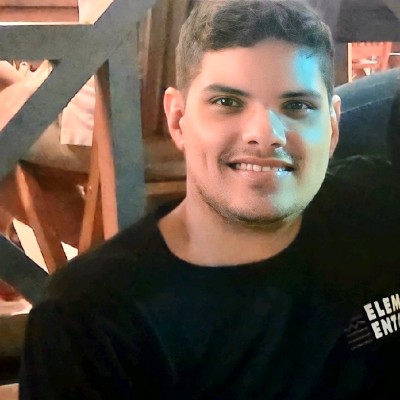
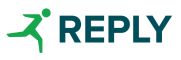