Ruby on Rails Q & A
What are the Rails security best practices?
Rails has always emphasized convention over configuration, and this principle extends to its security practices. However, as with any framework, developers should be vigilant about potential vulnerabilities. Here are some security best practices for Rails applications:
- SQL Injection: Always use ActiveRecord’s query methods, which automatically handle data sanitization, instead of manually constructing SQL strings. For instance, prefer `User.where(email: params[:email])` over `User.where(“email = ‘#{params[:email]}'”)`.
- Cross-site Scripting (XSS): Rails automatically escapes content rendered in views to prevent XSS. Always use the provided view helpers, like `link_to`, and be cautious when using `html_safe` or `raw`, as these methods can introduce vulnerabilities if misused.
- Cross-site Request Forgery (CSRF): Rails includes CSRF protection out-of-the-box. Ensure it’s enabled by including the `protect_from_forgery` directive in your `ApplicationController`. This provides a token that’s validated for POST, PUT, DELETE, and PATCH requests.
- Session Hijacking: Use HTTPS to encrypt data between the client and server, making it harder for attackers to eavesdrop on sessions. Also, set the `secure` flag on cookies to ensure they’re only transmitted over secure connections.
- Mass Assignment: Use `strong parameters` to whitelist attributes that can be mass-assigned. This prevents attackers from updating sensitive model attributes. Example: `params.require(:user).permit(:name, :email)`.
- Redirection and Files: Always validate redirection URLs and don’t use user input directly for redirection or file rendering. An attacker could use this for phishing attacks or to expose internal files.
- Dependencies: Regularly audit your gem dependencies for vulnerabilities. Tools like `bundler-audit` can be invaluable in checking your `Gemfile.lock` against known vulnerabilities.
- Password Storage: Use a strong, slow hash function like `bcrypt` for password storage. The `has_secure_password` feature in Rails uses `bcrypt` by default, which provides a good balance of security and performance.
- Clickjacking: Employ the `Content Security Policy` (CSP) header to specify which sources of content are valid, reducing risks of clickjacking attacks.
- Logging: Be careful not to log sensitive information, especially in production. Passwords, API keys, and personal user data should be kept out of logs.
While Rails provides a strong foundation for secure web development, developers must remain informed and cautious. Regularly consulting the Rails security guide and staying updated on best practices is essential.
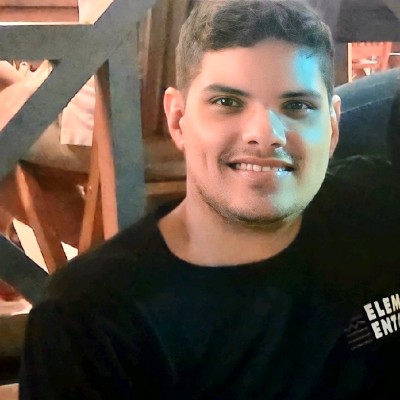
Previously at
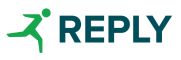
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.