How to Use Ruby Functions for Social Media Integration
In today’s digital world, social media integration is vital for businesses and individuals alike to connect with their audience and expand their reach. Ruby, a versatile programming language, offers an array of powerful functions that can simplify the process of integrating social media platforms into your applications. In this blog, we will explore how to effectively use Ruby functions for social media integration, along with code samples and practical tips to get you started.
Table of Contents
1. Understanding Social Media Integration:
Social media integration is the process of incorporating social media features and functionalities into your web or mobile applications. It enables users to interact with social media platforms, such as Twitter, Facebook, Instagram, etc., from within your app, fostering better engagement and user experience.
2. Installing Required Gems:
To begin integrating social media into your Ruby application, you’ll need to install the necessary gems. Gems are packages that extend Ruby’s functionality and provide pre-built modules for social media integration. Some essential gems for social media integration are twitter, koala, and omniauth.
ruby # Gemfile source 'https://rubygems.org' gem 'twitter' gem 'koala' gem 'omniauth' # Add other gems required for your project
To install the gems, run the following command:
bash $ bundle install
3. Authentication with Social Media APIs:
Before you can access social media data or post content on behalf of users, you must authenticate your application with the respective social media APIs. Let’s see how to authenticate with the Twitter API and Facebook Graph API.
3.1. Twitter API Integration:
To use the Twitter API, create a Twitter Developer account and obtain the necessary API keys and tokens.
ruby # twitter_auth.rb require 'twitter' # Set your Twitter API credentials client = Twitter::REST::Client.new do |config| config.consumer_key = 'YOUR_CONSUMER_KEY' config.consumer_secret = 'YOUR_CONSUMER_SECRET' config.access_token = 'YOUR_ACCESS_TOKEN' config.access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET' end # Now you can use the 'client' object to interact with the Twitter API
3.2. Facebook Graph API Integration:
For the Facebook Graph API, you need to create a Facebook Developer account and acquire an access token.
ruby # facebook_auth.rb require 'koala' # Set your Facebook access token access_token = 'YOUR_ACCESS_TOKEN' # Initialize the Koala API client graph = Koala::Facebook::API.new(access_token) # Now you can use the 'graph' object to interact with the Facebook Graph API
4. Posting to Social Media:
Once authenticated, you can start posting content to social media platforms programmatically.
4.1. Tweeting with Ruby:
To post tweets using Ruby, the twitter gem simplifies the process:
ruby # tweet.rb require 'twitter' # Authenticate with Twitter (use 'twitter_auth.rb' from the previous section) # ... # Post a tweet tweet_text = 'Hello, Twitter! This is my first tweet using Ruby!' client.update(tweet_text) puts 'Tweet posted successfully!'
4.2. Posting to Facebook with Ruby:
With the koala gem, you can easily publish posts on Facebook:
ruby # post_to_facebook.rb require 'koala' # Authenticate with Facebook (use 'facebook_auth.rb' from the previous section) # ... # Post to Facebook post_text = 'Check out this awesome blog on social media integration with Ruby!' graph.put_wall_post(post_text) puts 'Post published on Facebook!'
5. Retrieving Social Media Data:
Besides posting, you can also fetch data from social media platforms using Ruby functions.
5.1. Fetching Tweets:
Using the Twitter gem, you can retrieve tweets based on various parameters:
ruby # fetch_tweets.rb require 'twitter' # Authenticate with Twitter (use 'twitter_auth.rb' from the previous section) # ... # Fetch tweets tweets = client.search('Ruby programming', result_type: 'recent', lang: 'en', count: 5) tweets.each do |tweet| puts "@#{tweet.user.screen_name}: #{tweet.text}" end
5.2. Retrieving Facebook Posts:
With the Koala gem, you can access posts from your Facebook page or profile:
ruby # fetch_facebook_posts.rb require 'koala' # Authenticate with Facebook (use 'facebook_auth.rb' from the previous section) # ... # Fetch Facebook posts posts = graph.get_connections('me', 'posts', { limit: 5 }) posts.each do |post| puts post['message'] end
6. Scheduling Social Media Posts:
Scheduling social media posts is a great way to maintain a consistent online presence. Let’s explore how to schedule posts using the whenever gem and by building a scheduler with Ruby.
6.1. Using the whenever Gem:
The whenever gem allows you to set up a cron job to execute Ruby scripts at specific intervals:
ruby # Gemfile source 'https://rubygems.org' gem 'whenever' # …
6.2. Define the schedule in the schedule.rb file:
ruby # schedule.rb every 1.day, at: '10:00 am' do rake 'social_media:post_tweet' end every 3.days, at: '3:00 pm' do rake 'social_media:post_to_facebook' end
6.3. Run the following command to update the cron job:
bash $ whenever --update-crontab
6.4. Building a Scheduler with Ruby:
Alternatively, you can create a custom Ruby script to schedule social media posts:
ruby # social_media_scheduler.rb require 'rufus-scheduler' require_relative 'tweet' require_relative 'post_to_facebook' scheduler = Rufus::Scheduler.new # Schedule tweets every day at 10:00 am scheduler.cron('0 10 * * *') do Tweet.post_tweet('This is a scheduled tweet using Ruby!') end # Schedule Facebook posts every 3 days at 3:00 pm scheduler.cron('0 15 */3 * *') do PostToFacebook.post('Scheduled post on Facebook!') end # Start the scheduler scheduler.join
7. Analyzing Social Media Data:
Analyzing social media data can provide valuable insights. Two common analysis techniques are sentiment analysis and social media insights.
7.1. Sentiment Analysis:
Sentiment analysis helps determine the sentiment (positive, negative, neutral) behind social media posts. Using the sentimental gem, you can perform basic sentiment analysis in Ruby:
ruby # Gemfile source 'https://rubygems.org' gem 'sentimental' # …
7.2. Perform sentiment analysis on a tweet:
ruby # sentiment_analysis.rb require 'sentimental' # Load the default sentiment analysis lexicon Sentimental.load_defaults # Analyze a tweet's sentiment analyzer = Sentimental.new tweet_text = 'I love Ruby! It is an amazing language.' score = analyzer.score(tweet_text) if score > 0 puts 'The tweet has a positive sentiment!' elsif score < 0 puts 'The tweet has a negative sentiment!' else puts 'The tweet is neutral.' end
7.3. Social Media Insights:
To gain deeper insights into your social media performance, you can use third-party analytics tools or APIs provided by the respective platforms.
8. Best Practices for Social Media Integration:
As you integrate social media into your applications, keep these best practices in mind:
- Securely manage API keys and tokens.
- Implement error handling to gracefully handle API issues.
- Respect user privacy and data protection regulations.
- Monitor API usage to stay within rate limits.
- Regularly update and maintain your integration to adapt to API changes.
Conclusion
In this blog, we explored the power of Ruby functions for social media integration. By leveraging the twitter and koala gems, we learned how to authenticate with social media APIs, post content, retrieve data, and even schedule social media posts. Moreover, we touched upon sentiment analysis and social media insights to enhance our understanding of user interactions. As you delve deeper into social media integration, remember to stay updated with the latest API changes and explore further possibilities to make your social media presence truly engaging and effective. Happy coding!
Table of Contents
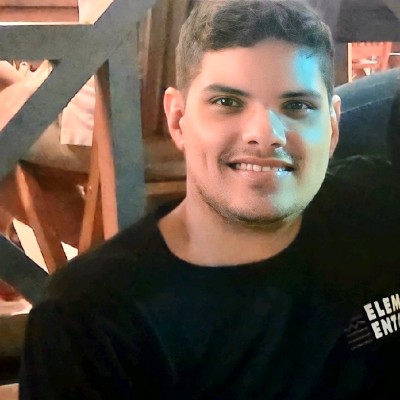
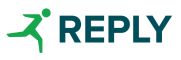