How to Use Ruby Functions for Speech Recognition and Synthesis
In today’s world, speech recognition and synthesis are becoming increasingly important in various applications, from voice assistants to automated customer support systems. Ruby, a dynamic and versatile programming language, offers powerful tools and libraries for handling these tasks. In this blog, we will dive into the fascinating world of speech recognition and synthesis using Ruby functions. We’ll explore the fundamentals, practical examples, and code samples to help you get started.
Table of Contents
1. Understanding Speech Recognition
1.1. What Is Speech Recognition?
Speech recognition, also known as speech-to-text or automatic speech recognition (ASR), is the process of converting spoken language into written text. It enables machines to understand and interpret human speech, making it a crucial component in voice-controlled applications and transcription services.
1.2. Using the pocketsphinx-ruby Gem for Speech Recognition
Ruby provides a variety of gems and libraries for speech recognition. One of the popular choices is the pocketsphinx-ruby gem, which is a Ruby wrapper for the CMU PocketSphinx speech recognition engine.
Here’s how you can use the pocketsphinx-ruby gem to recognize speech:
ruby # Install the gem gem install pocketsphinx-ruby # Create a recognizer require 'pocketsphinx-ruby' recognizer = Pocketsphinx::LiveSpeechRecognizer.new # Start recognizing recognizer.recognize do |speech| puts "You said: #{speech}" end
In this code snippet, we first install the pocketsphinx-ruby gem, create a speech recognizer, and then start recognizing speech in real-time. The recognize method captures spoken words and prints them to the console.
2. Practical Application: Building a Voice Assistant
2.1. Building a Simple Voice Assistant
Now that we have a basic understanding of speech recognition in Ruby, let’s take it a step further by building a simple voice assistant. Our voice assistant will respond to specific commands and provide predefined responses.
ruby require 'pocketsphinx-ruby' # Create a recognizer recognizer = Pocketsphinx::LiveSpeechRecognizer.new # Define command-response mappings commands = { 'hello' => 'Hello there!', 'what is the weather like today' => 'I am sorry, I do not have access to weather information.', 'tell me a joke' => 'Why did the programmer go broke? Because he used up all his cache!', 'exit' => 'Goodbye!' } # Start recognizing recognizer.recognize do |speech| command = speech.downcase response = commands[command] puts response || "I didn't understand that." break if command == 'exit' end
In this code snippet, we create a simple voice assistant that responds to commands like “hello,” “tell me a joke,” and “exit.” The assistant listens for commands, matches them to predefined responses, and executes the corresponding action.
3. Exploring Speech Synthesis
3.1. What Is Speech Synthesis?
Speech synthesis, also known as text-to-speech (TTS), is the process of converting text into spoken language. It allows computers to generate human-like speech, which is valuable in applications such as screen readers, voice assistants, and audio content creation.
3.2. Using the espeak Gem for Speech Synthesis
Ruby provides several options for text-to-speech synthesis, including the espeak gem. This gem allows you to generate speech from text with ease.
Here’s how you can use the espeak gem for speech synthesis:
ruby # Install the gem gem install espeak-ruby # Create a speech synthesizer require 'espeak' synthesizer = ESpeak::Speech.new("Hello, Ruby speech synthesis!") # Generate speech synthesizer.speak
In this code snippet, we first install the espeak gem, create a speech synthesizer, and then generate speech from the provided text. The speak method plays the generated speech.
4. Practical Application: Creating an Audio Book
4.1. Converting Text to Speech for an Audio Book
Let’s put our knowledge of speech synthesis to practical use by creating a simple Ruby script that converts a text file into an audio book. We’ll use the espeak gem to accomplish this.
ruby require 'espeak' # Define the text to be converted text = File.read('book.txt') # Create a speech synthesizer synthesizer = ESpeak::Speech.new(text) # Generate speech and save it as an audio file synthesizer.save('book_audio.wav')
In this example, we read the content of a text file, create a speech synthesizer, and generate speech from the text. We then save the generated speech as an audio file in WAV format. You can modify this script to convert entire books or articles into audio.
5. Combining Speech Recognition and Synthesis
5.1. Building a Conversational Ruby Application
Now that we’ve covered speech recognition and synthesis separately, let’s explore a more complex scenario: building a conversational Ruby application. This application will recognize spoken commands and respond using text-to-speech synthesis.
ruby require 'pocketsphinx-ruby' require 'espeak' # Create a recognizer recognizer = Pocketsphinx::LiveSpeechRecognizer.new # Create a speech synthesizer synthesizer = ESpeak::Speech.new # Start recognizing recognizer.recognize do |speech| command = speech.downcase response = case command when 'hello' 'Hello there!' when 'what is the weather like today' 'I am sorry, I do not have access to weather information.' when 'tell me a joke' 'Why did the programmer go broke? Because he used up all his cache!' when 'exit' 'Goodbye!' else "I didn't understand that." end puts "You said: #{command}" puts "Response: #{response}" # Generate and play the response synthesizer.text = response synthesizer.save('response_audio.wav') `aplay response_audio.wav` break if command == 'exit' end
In this more advanced example, we combine speech recognition and synthesis to create a conversational Ruby application. The application listens for spoken commands, generates text-based responses, converts these responses into speech, and plays them back to the user.
Conclusion
Speech recognition and synthesis are exciting areas of technology with numerous practical applications. Ruby, with its rich ecosystem of gems and libraries, provides a robust platform for implementing these features in your projects. Whether you’re building voice assistants, accessibility tools, or creative applications like audio books, Ruby has you covered. Start exploring the possibilities today and give your applications a voice of their own.
In this blog post, we’ve covered the fundamentals of speech recognition and synthesis in Ruby, provided code samples, and demonstrated practical applications. Armed with this knowledge, you can embark on your journey to create innovative and interactive voice-powered applications using Ruby. Happy coding!
Table of Contents
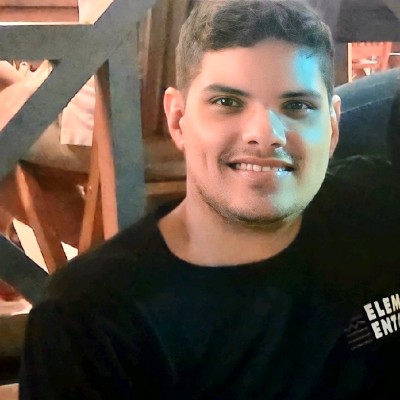
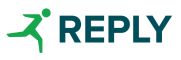