How to Use Ruby Functions for URL Encoding and Decoding
In the world of web development, URLs play a crucial role in defining the structure of web pages and facilitating data exchange between the client and server. However, URLs may contain special characters, spaces, and non-ASCII characters, making them prone to errors and security vulnerabilities. To handle such situations, URL encoding and decoding become indispensable tasks. Fortunately, Ruby offers built-in functions to effortlessly encode and decode URLs, ensuring proper data transmission and enhancing web application security.
Table of Contents
In this blog post, we’ll delve into the concept of URL encoding and decoding and explore how Ruby functions can be employed to carry out these operations effectively. We’ll cover essential concepts, best practices, and practical examples, so you can confidently implement URL encoding and decoding in your Ruby projects.
1. Introduction to URL Encoding and Decoding
URLs are used to identify resources on the internet and are composed of various components, such as the protocol, domain, path, query parameters, and fragments. However, URLs can only contain a limited set of characters, excluding special characters and non-ASCII characters, which can lead to conflicts and errors when transmitting data via URLs.
URL encoding is the process of converting reserved and unsafe characters into a URL-safe format, typically using percent-encoding (also known as URL encoding). Conversely, URL decoding involves translating encoded characters back to their original form.
2. The Importance of URL Encoding and Decoding
Proper URL encoding is crucial for preserving data integrity during data transmission. By encoding URLs, you ensure that special characters and non-ASCII characters are correctly represented and interpreted by web servers and browsers. Additionally, URL encoding helps in avoiding conflicts with reserved characters, preventing unexpected behavior in your web applications.
On the other hand, URL decoding is essential when handling incoming data from URLs. It allows you to extract parameters and values correctly, ensuring accurate processing of user input and avoiding potential security vulnerabilities.
3. URL Encoding in Ruby
3.1. Using the URI.encode Method
In Ruby, the URI module provides the encode method, which can be used to perform URL encoding. Let’s take a look at a simple example:
ruby require 'uri' # URL encode a string original_string = 'Hello, Ruby URL Encoding!' encoded_string = URI.encode(original_string) puts encoded_string
Output:
perl Hello%2C%20Ruby%20URL%20Encoding%21
In this example, the URI.encode method encodes spaces as %20 and the comma as %2C. These encoded characters represent the URL-safe format of their respective special characters.
3.2. Handling Special Characters
During URL encoding, certain characters like ?, =, &, and / are considered safe and not encoded. However, in some cases, you may need to encode these characters manually to avoid conflicts in your URLs:
ruby require 'uri' # URL encode with custom safe characters original_string = 'https://example.com?search=ruby&category=programming' encoded_string = URI.encode(original_string, '&=') puts encoded_string
Output:
perl https://example.com%3Fsearch%3Druby%26category%3Dprogramming
In this example, the & and = characters are manually encoded as %3F and %26, respectively.
3.3. Encoding Parameters in URLs
URLs often contain query parameters that need to be encoded correctly. In Ruby, you can use the URI.encode_www_form method to encode multiple query parameters:
ruby require 'uri' # URL encode query parameters params = { search: 'ruby programming', page: 1 } encoded_query = URI.encode_www_form(params) url = "https://example.com/search?#{encoded_query}" puts url
Output:
sql https://example.com/search?search=ruby+programming&page=1
The URI.encode_www_form method handles spaces by encoding them as +, making the URL more readable.
4. URL Decoding in Ruby
4.1. The URI.decode Method
Similarly, Ruby provides the decode method in the URI module to perform URL decoding. Let’s decode the previously encoded URL:
ruby require 'uri' # URL decode a string encoded_string = 'Hello%2C%20Ruby%20URL%20Encoding%21' decoded_string = URI.decode(encoded_string) puts decoded_string
Output:
mathematica Hello, Ruby URL Encoding!
The URI.decode method decodes the percent-encoded characters back to their original form.
4.2. Decoding URL Components
When dealing with URLs, you may need to decode individual components separately. For example, to decode only the query parameters from a URL, you can use the URI.decode_www_form method:
ruby require 'uri' # URL decode query parameters encoded_query = 'search=ruby+programming&page=1' decoded_params = URI.decode_www_form(encoded_query) puts decoded_params.to_h
Output:
arduino {"search"=>"ruby programming", "page"=>"1"}
The URI.decode_www_form method decodes the + character back to spaces, giving us the original query parameters.
5. Best Practices for URL Encoding and Decoding
5.1. Identifying When to Use Encoding or Decoding
Properly identify situations where you need to perform URL encoding or decoding. When constructing URLs with dynamic data, encode the parameters to ensure correct transmission. Conversely, when handling incoming URLs and extracting parameters, use URL decoding to process the data correctly.
5.2. Handling Errors and Exceptions
When dealing with user-generated URLs or external data, always handle potential errors and exceptions. Invalid or improperly encoded URLs can lead to unexpected behavior or security vulnerabilities. Implement proper error handling and validation to ensure the data is safe and consistent.
5.3. Securing Your URL Parameters
Be cautious when using URL parameters to prevent security threats like SQL injection and cross-site scripting (XSS). Sanitize and validate user input to minimize risks and ensure a secure application.
6. Practical Use Cases
6.1. Building Query Strings with URL Encoding
URL encoding is commonly used when constructing query strings for API calls or search requests. Let’s see an example of encoding query parameters for a hypothetical search API:
ruby require 'uri' # URL encode query parameters for API request query_params = { keyword: 'ruby programming', page: 1 } encoded_query = URI.encode_www_form(query_params) api_endpoint = "https://api.example.com/search?#{encoded_query}" puts api_endpoint
Output:
arduino https://api.example.com/search?keyword=ruby+programming&page=1
The encoded query parameters are safely added to the API endpoint, ensuring correct data transmission.
6.2. Handling Dynamic URLs
In web applications, you may encounter scenarios where URLs are dynamically generated based on user input or database content. URL encoding helps ensure that such URLs are correctly encoded, avoiding any potential issues:
ruby require 'uri' # URL encode dynamic content in URL user_input = 'Hello, Ruby URL Encoding!' encoded_content = URI.encode(user_input) dynamic_url = "https://example.com/#{encoded_content}" puts dynamic_url
Output:
perl https://example.com/Hello%2C%20Ruby%20URL%20Encoding%21
By URL encoding the dynamic content, we ensure that any special characters in the user input are safely represented in the URL.
7. Tips for URL Sanitization and Validation
Always validate user input to prevent malicious data from being transmitted via URLs.
Sanitize user-generated URLs to avoid issues caused by improperly encoded characters or reserved characters.
Use URL encoding for transmitting sensitive information via URLs to ensure data privacy and security.
Conclusion
URL encoding and decoding are essential techniques in web development that help in ensuring proper data transmission and enhancing web application security. In this blog post, we explored the concepts of URL encoding and decoding and learned how to utilize Ruby functions to perform these operations efficiently. By employing the methods provided by the URI module, you can confidently handle URLs, query parameters, and dynamic content in your Ruby projects, creating robust and secure web applications.
Incorporate the knowledge gained from this guide into your development process, and you’ll be well-equipped to build web applications that handle URLs with precision and reliability. Happy coding!
Table of Contents
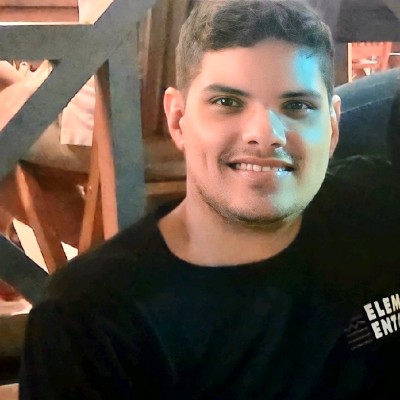
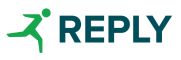