How to use ActionMailer in Rails?
ActionMailer in Rails provides a framework to send emails from your application using mailer classes and views. Here’s how you can set it up and use it effectively:
- Setting Up ActionMailer:
Start by configuring your email delivery method in the `config/environments/development.rb` or `config/environments/production.rb`:
```ruby config.action_mailer.delivery_method = :smtp config.action_mailer.smtp_settings = { address: 'smtp.mailserver.com', port: 587, user_name: 'your_username', password: 'your_password', authentication: 'plain', enable_starttls_auto: true } ```
- Generating Mailer:
Use the rails generator to create a new mailer, for instance, a UserMailer:
``` rails generate mailer UserMailer ```
This will generate a `user_mailer.rb` file in `app/mailers` and a `user_mailer` directory inside `app/views` for your mailer views.
- Creating Mailer Action:
In the generated mailer class (`user_mailer.rb`), define a method for each email you wish to send. For instance:
```ruby class UserMailer < ApplicationMailer default from: 'notifications@example.com' def welcome_email(user) @user = user mail(to: @user.email, subject: 'Welcome to My Awesome Site') end end ```
- Mailer Views:
For each mailer action, you can create corresponding views for the email’s body. For the `welcome_email` action, create `welcome_email.html.erb` (for HTML emails) and/or `welcome_email.text.erb` (for plain text emails) inside `app/views/user_mailer`.
- Sending the Email:
Once your mailer action and views are ready, you can send the email from anywhere in your Rails application with:
```ruby UserMailer.welcome_email(@user).deliver_now ```
- Testing:
ActionMailer provides ways to test emails without actually sending them. You can check email attributes, like subject or receiver, and even the content.
ActionMailer in Rails offers a structured way to manage and send emails from your application. With its integration of mailer actions and views, it makes sending rich, dynamic emails as straightforward as rendering an HTML page.
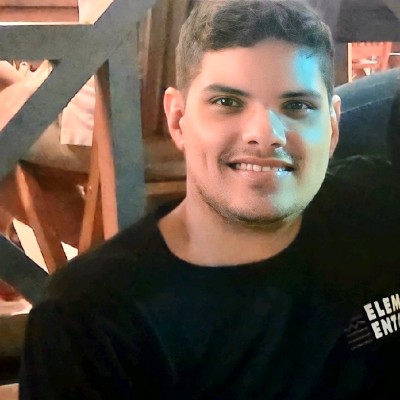
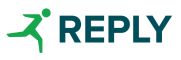