How to use nested forms in Rails?
In Rails, nested forms allow you to create or edit multiple model objects with a single form submission. This is particularly useful when you have parent-child relationships, where you want to manage child objects directly from the parent’s form. A common example is creating an `Invoice` model with multiple `LineItem` entries in a single form.
To implement nested forms:
- Model Configuration:
First, you’ll want to set up the parent model (`Invoice`) to accept nested attributes for its associated model (`LineItem`):
```ruby class Invoice < ApplicationRecord has_many :line_items accepts_nested_attributes_for :line_items, allow_destroy: true, reject_if: :all_blank end ```
The `accepts_nested_attributes_for` method provides the foundation for handling the attributes of associated models. The `allow_destroy` option lets you remove a child model via the form, while `reject_if` prevents saving blank records.
- Form Configuration:
In the parent model’s form (`Invoice`), use the `fields_for` method to define the nested fields for the associated model (`LineItem`):
```erb <%= form_with(model: @invoice) do |f| %> <!-- Invoice fields here --> <%= f.fields_for :line_items do |line_item_form| %> <%= line_item_form.label :description %> <%= line_item_form.text_field :description %> <%= line_item_form.label :amount %> <%= line_item_form.number_field :amount %> <% end %> <%= f.submit %> <% end %> ```
- Controller Configuration:
Make sure the controller permits the nested attributes:
```ruby def invoice_params params.require(:invoice).permit(:your_invoice_attributes_here, line_items_attributes: [:id, :description, :amount, :_destroy]) end ```
The `:id` and `:_destroy` attributes are essential to handle the updating and deletion of child records.
- Adding & Removing Nested Forms Dynamically:
To enhance the user experience, you might want to dynamically add or remove nested form fields using JavaScript. This involves writing JS to clone and remove form field templates, updating the nested attributes’ index, and handling their display in the view.
Nested forms in Rails offer a streamlined way to handle complex, hierarchical data structures. Proper setup ensures you can create, edit, and delete associated records seamlessly within a single form. Ensure you have validations in place to catch any unexpected or invalid inputs, keeping your data integrity intact.
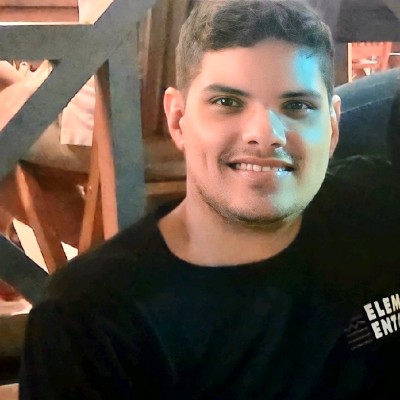
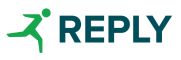