What are Rails concerns and when should I use them?
Rails concerns, introduced with Rails 4, are a way to make your models, controllers, and other classes more modular by extracting reusable pieces of functionality into mixable modules. They’re part of Rails’ approach to adhere to the DRY (Don’t Repeat Yourself) principle, aiding in organizing and decluttering large models or controllers. Let’s delve into the specifics:
- Purpose and Structure: A concern is essentially a module that extends `ActiveSupport::Concern`. It allows you to encapsulate a specific functionality that you then can mix into any model or controller. This is especially useful when you find that certain behaviors or methods are being used across multiple classes.
- Location: Concerns are typically stored in the `app/models/concerns` or `app/controllers/concerns` directories, depending on their intended usage.
- Usage: To create a concern, you might have something like:
```ruby module Commentable extend ActiveSupport::Concern included do has_many :comments, as: :commentable end def recent_comments comments.order('created_at DESC').limit(10) end end ```
To then use this concern in a model, you’d simply include it:
```ruby class Post < ApplicationRecord include Commentable end ```
- When to Use Them: While concerns can be powerful, they should be used judiciously. Here are some guidelines:
– Reusability: When a piece of functionality is clearly reusable across multiple models or controllers.
– Decomposition: When a model or controller becomes too bulky or violates the Single Responsibility Principle. Using concerns can help decompose it into more focused segments.
– Avoid Overuse: It’s crucial not to slice models or controllers too thinly, as having too many concerns can make the code harder to follow.
- Criticism: Some developers feel that concerns can sometimes be overused, leading to obscured logic and harder-to-follow codebases. It’s essential to strike a balance, ensuring that concerns are used to genuinely enhance modularity and clarity.
Rails concerns provide a structured way to modularize and share code between models, controllers, or both. When used judiciously, they can be instrumental in creating clean, maintainable, and DRY Rails applications. However, as with all tools, they’re most effective when used with discretion and a clear understanding of their benefits and potential pitfalls.
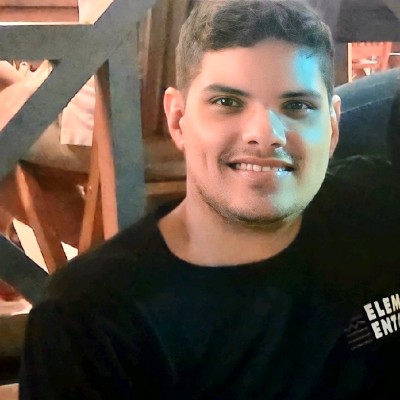
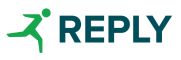