How to use virtual attributes in Rails?
In Rails, models typically map directly to database tables, and their attributes correspond to columns in the table. However, sometimes you may want to add attributes to a model that don’t have corresponding columns in the database. These are known as “virtual attributes.”
Virtual attributes in Rails are attributes that are not persisted to the database but behave much like regular attributes. They can be useful for various scenarios, like consolidating or transforming data before saving, or interacting with form fields that don’t map directly to the database.
To implement a virtual attribute:
- Define Accessor Methods: Use `attr_accessor` to create getter and setter methods for your virtual attribute. For example, for a virtual attribute named `full_name`:
```ruby attr_accessor :full_name ```
- Manipulating Data: Often, virtual attributes serve as a way to manipulate data. Suppose you have `first_name` and `last_name` in the database but want a `full_name` attribute for forms. You could define the getter and setter as:
```ruby def full_name [first_name, last_name].join(' ') end def full_name=(name) split = name.split(' ', 2) self.first_name = split.first self.last_name = split.last end ```
- Validations: Virtual attributes can also be validated just like regular attributes. For instance, if you wish to validate the presence of `full_name`:
```ruby validates :full_name, presence: true ```
- Usage in Forms: Virtual attributes can be used in forms just like regular attributes. If you’ve defined `full_name` as a virtual attribute, you can use `f.text_field :full_name` in your form.
- Limitation: Remember, virtual attributes are not saved to the database. So if you set a virtual attribute and later retrieve the same record from the database, the virtual attribute will be `nil` or whatever default value you’ve set in its getter method.
Virtual attributes in Rails provide a powerful way to enhance models with non-persistent attributes. They allow for more flexible data manipulation and can simplify interactions with forms. However, it’s crucial to remember their transient nature and handle them appropriately in your application logic.
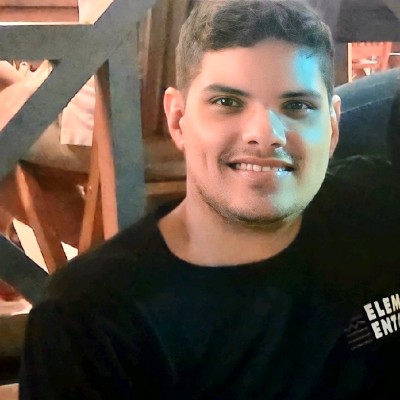
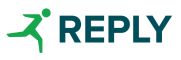