How to use ActionCable in Rails?
ActionCable is a framework in Rails that seamlessly integrates WebSockets into your application, allowing for real-time features like chat, notifications, and live updates. By using WebSockets, Rails can push content to the connected users as it becomes available, ensuring a more interactive and dynamic user experience. Let’s walk through the basics of integrating ActionCable:
- Setup: If you generated your Rails application with Rails 5 or later, ActionCable should already be set up. If not, you’ll need to add `gem ‘actioncable’` to your Gemfile and run `bundle install`.
- Channels: In ActionCable, communication is organized around channels. A channel is like a controller, but for WebSocket communication. You can generate a new channel using:
```bash rails generate channel MyChannel ```
- Server-Side Logic: Inside your generated channel (e.g., `app/channels/my_channel.rb`), you’ll define methods that handle different WebSocket events:
```ruby class MyChannel < ApplicationCable::Channel def subscribed stream_from "my_channel" end def receive(data) # Handle data sent from the client-side end end ```
- Client-Side Logic: Corresponding JavaScript code will be generated in `app/javascript/channels/my_channel.js`. This is where you can define client-side behavior for when data is received from the server:
```javascript consumer.subscriptions.create("MyChannel", { received(data) { // Handle data received from the server } }); ```
- Broadcasting: To send data from the server to clients, you can use broadcasting. From anywhere in your Rails app, you can broadcast to a channel:
```ruby ActionCable.server.broadcast('my_channel', message: 'Hello World!') ```
- Deployment Considerations: For deploying apps using ActionCable, you’d typically need a server setup that supports WebSocket, like Puma. Additionally, if you’re deploying on platforms like Heroku, you might need to use an add-on like Redis to manage the WebSocket connections.
ActionCable offers a built-in solution in Rails to leverage WebSockets, making real-time features more accessible for developers. By following the conventions and patterns set out by the framework, you can quickly add dynamic, real-time elements to enhance your application’s user experience. However, always ensure you understand the scalability and deployment implications when working with real-time features.
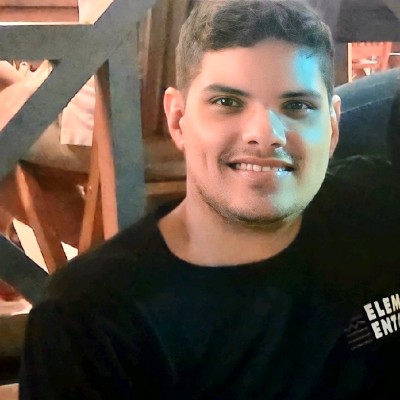
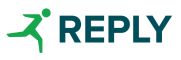