What are Rails validations and how to use them?
Rails validations are essential tools provided by the ActiveRecord ORM to ensure that only valid data gets saved into the database. They’re a set of conditions specified in your models that the data must meet before being persisted. Here’s a breakdown:
- Why Use Validations?: Validations are crucial for maintaining data integrity, enhancing security, and improving the user experience. By catching and responding to invalid data immediately, you can prevent potential database errors, protect from malicious input, and give feedback to the user.
- Common Validations: Rails provides a suite of built-in validations. Here are a few examples:
– `presence`: ensures that a value is not empty.
```ruby validates :name, presence: true ```
– `uniqueness`: ensures a value doesn’t already exist in the database.
```ruby validates :email, uniqueness: true ```
– `format`: ensures a value matches a provided regular expression.
```ruby validates :email, format: { with: URI::MailTo::EMAIL_REGEXP } ```
– `length`: validates the length of a value.
```ruby validates :password, length: { minimum: 8 } ```
- Custom Validations: Beyond built-in helpers, you can define your custom validation methods:
```ruby validate :custom_criteria def custom_criteria errors.add(:base, "Error message") if some_condition_is_not_met end ```
- Using Validations: Once validations are set up in a model, Rails will automatically check them whenever you try to save or update an object. If any validation fails, the action will halt, and the record won’t be saved.
- Handling Validation Errors: After a failed validation, you can access the specific errors through the `.errors` method on the instance:
```ruby user = User.new(email: "invalid") user.valid? # returns false user.errors.messages # returns a hash of the errors ```
- Displaying Errors: In views, you can inform users of validation errors. For example, using the `form_for` helper, any validation error messages would automatically be wrapped around the erroneous form fields.
Validations in Rails offer an efficient, declarative way to ensure the integrity and consistency of your data. They act as safeguards, minimizing the risk of persisting erroneous, incomplete, or malicious data, and provide mechanisms to relay informative feedback to the end user, creating a more seamless and responsive application experience.
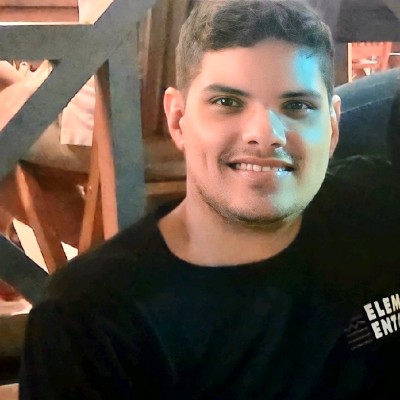
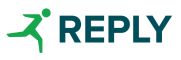