How to Use Ruby Functions for Video Streaming and Playback
In today’s digital age, video content has become an integral part of our lives. From streaming platforms to social media, videos are everywhere. As a developer, you might find yourself in need of tools to manipulate and serve videos efficiently. This is where Ruby, a versatile and dynamic programming language, comes into play. In this blog, we will explore how to use Ruby functions for video streaming and playback, unlocking the potential to create feature-rich applications and platforms.
Table of Contents
1. Understanding the Power of Ruby
Ruby is renowned for its elegance and simplicity in writing code. Its extensive library ecosystem and a thriving community make it a popular choice for web development, automation, and more. When it comes to video streaming and playback, Ruby offers a variety of gems (libraries) that can simplify the process. Before diving into the practical aspects, let’s take a moment to understand why Ruby is an excellent choice for this task:
1.1. Rich Ecosystem
Ruby boasts a vast ecosystem of gems, some specifically designed for video processing and streaming. These gems provide powerful functions and simplify complex tasks.
1.2. Easy to Learn
Ruby’s clean and intuitive syntax makes it accessible to developers of all skill levels. If you’re new to Ruby, don’t worry; you’ll quickly grasp the basics needed for video manipulation.
1.3. Cross-Platform Compatibility
Ruby is a cross-platform language, which means you can develop video applications that work seamlessly on various operating systems.
Now that we appreciate why Ruby is an ideal choice for video streaming and playback, let’s dive into the practical aspects.
2. Setting Up Your Environment
Before you can harness Ruby’s capabilities for video manipulation, you need to set up your development environment. Ensure you have Ruby installed on your system, and then follow these steps:
Step 1: Install Required Gems
Open your terminal and use the gem command to install the necessary gems for video streaming and manipulation. Two popular gems you’ll need are ffmpeg and streamio-ffmpeg. The ffmpeg gem provides an interface to the FFmpeg multimedia framework, while streamio-ffmpeg simplifies working with multimedia files.
ruby gem install ffmpeg streamio-ffmpeg
Step 2: Create a New Ruby Project
Now, create a new Ruby project folder and navigate to it in your terminal:
bash mkdir video_streaming_project cd video_streaming_project
Step 3: Start Coding
You’re all set to start coding for video streaming and playback. Let’s explore some common tasks and Ruby functions for this purpose.
3. Basic Video Playback
One of the fundamental tasks in video streaming is playing a video. Let’s begin with a simple example of how to use Ruby to play a video file.
ruby require 'streamio-ffmpeg' input_file = 'input.mp4' # Replace with your video file movie = FFMPEG::Movie.new(input_file) movie.transcode('output.mp4') # Output file puts 'Video playback complete.'
In the code above, we use the streamio-ffmpeg gem to open a video file (input.mp4) and transcode it to another format (output.mp4). This basic example demonstrates how Ruby can be used to manipulate video files efficiently.
4. Video Streaming on the Web
Now, let’s explore how to stream videos on the web using Ruby. Streaming video content is crucial for delivering a seamless user experience. We’ll use the popular Sinatra framework to create a simple web application for video streaming.
Step 1: Install Sinatra
Install Sinatra by running the following command:
ruby gem install sinatra
Step 2: Create a Sinatra Application
Create a new Ruby file, e.g., app.rb, and add the following code:
ruby require 'sinatra' get '/video' do content_type 'video/mp4' send_file 'output.mp4' end # Start the Sinatra web server set :bind, '0.0.0.0' set :port, 4567
In this code, we create a simple Sinatra application that serves a video file (output.mp4) with the MIME type video/mp4. When you access the /video endpoint, the video will be streamed to the browser.
Step 3: Start the Web Server
Run the Sinatra application using the following command:
ruby ruby app.rb
You can now access the video stream in your web browser by visiting http://localhost:4567/video.
This example demonstrates how easy it is to set up video streaming on the web using Ruby and Sinatra.
5. Adding Video Controls
To enhance the user experience, you can add video controls to allow users to play, pause, and adjust the video’s timeline. HTML5 provides native video controls, and you can generate the necessary HTML code using Ruby.
ruby require 'sinatra' get '/video' do content_type 'video/mp4' send_file 'output.mp4' end get '/player' do %( <!DOCTYPE html> <html> <head> <title>Video Player</title> </head> <body> <video width="640" height="360" controls> <source src="/video" type="video/mp4"> Your browser does not support the video tag. </video> </body> </html> ) end # Start the Sinatra web server set :bind, '0.0.0.0' set :port, 4567
In this code, we create a new /player endpoint that generates an HTML page with an embedded video player. The controls attribute adds native video controls to the player.
Now, when you visit http://localhost:4567/player, you’ll see the video with playback controls.
6. Advanced Video Editing
Ruby also allows you to perform advanced video editing tasks, such as trimming, adding watermarks, and merging videos. Let’s explore how to trim a video using the streamio-ffmpeg gem.
ruby require 'streamio-ffmpeg' input_file = 'input.mp4' output_file = 'trimmed.mp4' movie = FFMPEG::Movie.new(input_file) # Trim the video from 10 seconds to 30 seconds movie.transcode(output_file, { seek_time: 10, duration: 20 }) puts 'Video trimming complete.'
In this code, we open an input video file (input.mp4) and trim it from the 10-second mark to the 30-second mark, creating a new output file (trimmed.mp4). This is just one example of the advanced video editing capabilities Ruby offers.
Conclusion
Ruby’s versatility and simplicity make it an excellent choice for video streaming and playback. In this blog, we explored how to set up your environment, perform basic video playback, create a web application for video streaming, add video controls, and even delve into advanced video editing tasks. With the power of Ruby and its extensive ecosystem of gems, you have the tools you need to create feature-rich video applications and platforms.
Whether you’re building your own video streaming service, enhancing your website with multimedia content, or developing video editing software, Ruby’s capabilities are sure to simplify the process and deliver exceptional results. So, go ahead and leverage Ruby’s functions to take your video projects to the next level.
Table of Contents
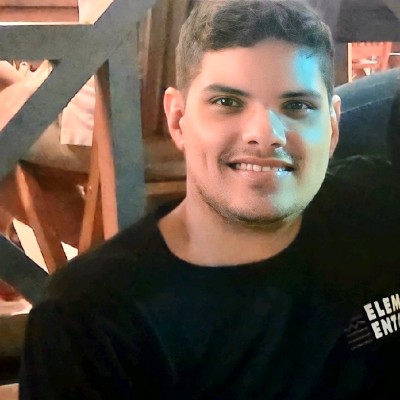
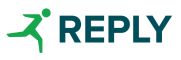