Working with Views and Templates on Ruby on Rails
Ruby on Rails is a robust web application framework written in Ruby. It is built to simplify common programming tasks, allowing developers to focus on writing high-quality code. In this tutorial, we’ll dive into the Rails view layer and templates.
Understanding Rails Views and Templates
In the Model-View-Controller (MVC) architecture, the view is responsible for generating the user interface, usually based on data provided by the controller. Views in Rails are typically written in ERB (Embedded Ruby), a templating language that lets you embed Ruby code in your HTML.
Creating a Basic View
Let’s start with a simple example. Imagine we have a “posts” controller, and we want to create a view for the “show” action.
- In your app, navigate to the “app/views/posts” directory. Create a new file named “show.html.erb“. This is where we’ll write the code for our view.
- Here’s some basic code for our “show” view:
<h1><%= @post.title %></h1> <p><%= @post.body %></p>
This simple HTML document includes two ERB tags. The `<%= %>` syntax tells Rails to evaluate the Ruby code inside and include the result in the final HTML. In this case, the title and body of the `@post` object.
Working with Partials
Partials are reusable snippets of code in your views. They can help you keep your code DRY (Don’t Repeat Yourself), which is a fundamental concept in software development.
To create a partial:
1. In your “app/views/posts” directory, create a new file named “_post.html.erb”. The underscore denotes that this is a partial.
2. Inside the “_post.html.erb” partial, write some code that you want to reuse. For example:
<div class="post"> <h2><%= post.title %></h2> <p><%= post.body %></p> </div>
To use this partial in your “show” view, you can use the `render` method, like this:
<h1>Post</h1> <%= render 'post', post: @post %>
Layouts and Yield
Layouts are a way to specify a common structure for multiple views. For example, you might want all your pages to have the same header and footer.
In your “app/views/layouts” directory, you can find the “application.html.erb” file. This is the default layout for your Rails app.
Here’s what a basic layout might look like:
<!DOCTYPE html> <html> <head> <title>MyApp</title> <%= csrf_meta_tags %> <%= csp_meta_tag %> <%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %> <%= javascript_pack_tag 'application', 'data-turbolinks-track': 'reload' %> </head> <body> <header> <!-- Header code here --> </header> <%= yield %> <footer> <!-- Footer code here --> </footer> </body> </html>
The `<%= yield %>` statement tells Rails where to insert the content for each view. Each view file will be inserted at the location of the `yield` in the layout file.
Advanced Topics
Rails views and templates also have advanced features that can make your code even more powerful and flexible.
Helpers
Helpers are methods that you can use in your views to keep your view code clean. Rails includes many built-in helper methods,
and you can also define your own.
For example, here’s how you can use the `link_to` helper to create a link:
<%= link_to 'View Post', post_path(@post) %>
Localization
Rails has excellent support for localization (l10n). You can easily create multiple versions of your views for different languages.
In your “config/locales” directory, you can create YAML files for each language you want to support. For example, you might have “en.yml” for English and “es.yml” for Spanish.
```yaml # en.yml en: hello: "Hello world" # es.yml es: hello: "Hola mundo"
Then, in your views, you can use the `t` (translate) method to display localized text:
```ruby <%= t('hello') %>
Rails will automatically use the correct locale based on the user’s settings.
Conclusion
The Rails view layer and templates are a powerful way to generate dynamic HTML. By understanding the basics of views, partials, layouts, helpers, and localization, you can create rich, interactive user interfaces for your Rails applications. Happy coding!
Table of Contents
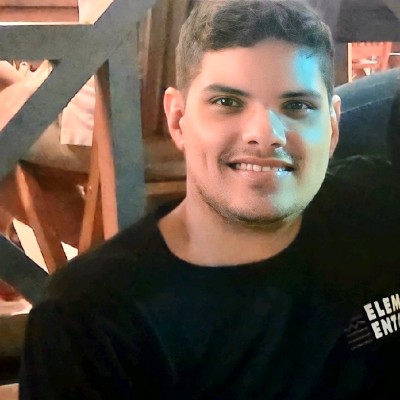
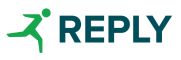