Ruby on Rails Q & A
How to write tests for a Rails application?
Testing is an integral part of the Rails framework, ensuring the reliability and stability of applications. Rails provides built-in support for unit tests, integration tests, and system tests, employing the Minitest framework by default.
- Setting up: Rails automatically sets up the testing environment for you when you create a new application. Inside the `test` directory, you’ll find subdirectories like `models`, `controllers`, `system`, etc., which hint at where different test types should reside.
- Unit Tests: These are for your models. They help verify that individual parts of your application function as intended. For example, you might have a `User` model and you want to test a method that checks if a user’s email is valid. You’d write this in the `test/models/user_test.rb` file.
```ruby test "should not save user without email" do user = User.new assert_not user.save, "Saved the user without an email" end ```
- Controller Tests: These are for your controllers and test how data flows through them. They can test things like ensuring the correct instance variables are set, redirects occur as intended, or certain templates are rendered.
- System Tests: Introduced in Rails 5.1, system tests allow you to test user interactions within your application’s interface. They use Capybara under the hood and can drive a real browser session.
- Fixtures: Located in `test/fixtures`, these are sample data that your tests run against. They allow you to have a known state for objects before tests are run.
- Running Tests: Simply use the command `rails test` in the terminal. Rails will execute all the test files and display the results.
- Factories and Mocks: While fixtures are the default, many developers opt to use libraries like FactoryBot and RSpec for a more flexible and dynamic testing approach. These tools, though external, integrate seamlessly into the Rails environment.
Rails offers a comprehensive testing setup, ensuring developers can maintain high-quality, bug-free applications. Familiarizing yourself with its testing conventions and tools can significantly enhance your application’s reliability and your efficiency as a developer.
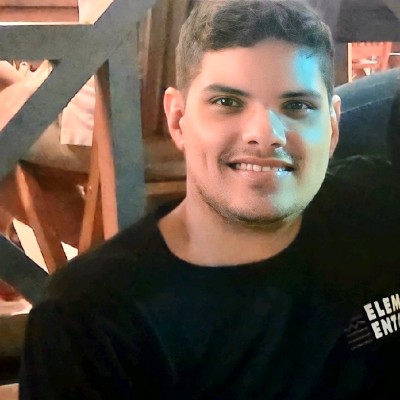
Previously at
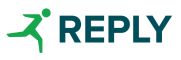
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.