Ruby Q & A
What are some best practices for writing clean and readable Ruby code?
Writing clean and readable Ruby code is crucial for ensuring that your codebase is maintainable, understandable, and less prone to bugs. Here are some best practices to follow:
- Consistent Indentation: Use a consistent indentation style, whether it’s spaces or tabs. Most Ruby developers prefer two spaces for each level of indentation.
- Descriptive Variable and Method Names: Choose meaningful and descriptive names for variables, methods, and classes. This makes your code self-documenting and easier for others to understand.
- Comments and Documentation: Add comments to clarify complex or non-obvious parts of your code. Document your classes and methods using tools like RDoc or YARD. Well-documented code is more accessible and maintainable.
- DRY (Don’t Repeat Yourself): Follow the DRY principle by avoiding code duplication. Extract repeated code into methods or modules to promote reusability and maintainability.
- Use Ruby Conventions: Stick to Ruby’s naming conventions, such as using snake_case for variables and methods and CamelCase for class names. This helps maintain consistency across your codebase.
- Avoid Global Variables: Limit the use of global variables, as they can lead to unexpected side effects and make it challenging to understand and debug code.
- Limit Line Length: Keep lines of code reasonably short, typically under 80 characters, to enhance readability. Break long lines into multiple lines when necessary.
- Follow Ruby Style Guide: Familiarize yourself with and adhere to the Ruby style guide, which provides a set of conventions and best practices for writing clean Ruby code.
- Testing and Test-Driven Development (TDD): Write tests for your code using a testing framework like RSpec. Embrace TDD by writing tests before implementing features. This ensures that your code works as expected and remains reliable during updates.
- Version Control: Use version control systems like Git to track changes to your code. Commit frequently with meaningful commit messages to keep a clear history of your project’s development.
- Modularize Code: Break down complex logic into smaller, manageable pieces by using classes, modules, and methods. This promotes code organization and makes it easier to understand.
- Error Handling: Implement proper error handling by using exceptions and providing meaningful error messages. This helps in diagnosing and resolving issues efficiently.
- Performance Optimization: Optimize your code for performance when necessary, but prioritize readability and maintainability. Profile your code to identify bottlenecks and address them selectively.
- Code Reviews: Encourage code reviews within your team. Multiple perspectives can help catch issues and improve code quality.
- Stay Informed: Stay up to date with the Ruby community, best practices, and evolving language features. Ruby is continuously evolving, and staying informed helps you leverage the latest improvements.
By following these best practices, you can write clean and readable Ruby code that is not only easier to maintain but also contributes to a more efficient and collaborative development process.
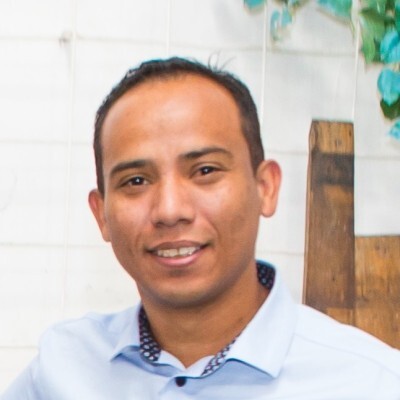
Previously at
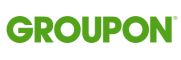
Experienced software professional with a strong focus on Ruby. Over 10 years in software development, including B2B SaaS platforms and geolocation-based apps.