Building E-commerce Applications with Ruby: Payment Gateways and Catalog Management
E-commerce has become an integral part of modern business, enabling companies to reach a global audience and provide convenient shopping experiences. Developing an e-commerce application requires careful consideration of various aspects, including payment gateways and catalog management. In this blog, we’ll delve into the world of building e-commerce applications using Ruby, focusing on the crucial elements of payment gateways and catalog management. We’ll explore the significance of these features, walk through their implementation, and provide code samples to guide you along the way.
Table of Contents
1. Understanding Payment Gateways
A payment gateway is a service that facilitates online transactions by securely processing payments between customers and merchants. It acts as a bridge between the e-commerce website and the financial institution, ensuring that sensitive payment information is encrypted and transmitted securely. Integrating a payment gateway into your e-commerce application is essential to provide customers with a seamless and secure checkout process.
2. Choosing the Right Payment Gateway
Before diving into the implementation, it’s important to choose the right payment gateway for your e-commerce application. Factors to consider include transaction fees, supported payment methods, security features, and integration complexity. Some popular payment gateways compatible with Ruby include Stripe, PayPal, and Braintree. In this tutorial, we’ll focus on integrating the Stripe payment gateway.
2.1. Integrating Stripe
Stripe is a widely-used payment gateway that offers a developer-friendly API and comprehensive documentation. To integrate Stripe into your Ruby e-commerce application, follow these steps:
2.1.1. Sign Up for Stripe:
Create an account on the Stripe website and obtain your API keys, including the publishable key and secret key.
2.1.2. Install the Stripe Gem:
In your Ruby project, use the following command to install the Stripe gem:
ruby gem install stripe
2.1.3. Configure Stripe:
In your application, configure Stripe with your API keys:
ruby require 'stripe' Stripe.api_key = 'your_secret_key'
2.1.4. Create a Payment Form:
Design a payment form where customers can enter their payment details, such as card number, expiration date, and CVC. Ensure that the form is secure and adheres to PCI DSS compliance standards.
2.1.5. Process Payment:
In your Ruby code, use the Stripe gem to process the payment:
ruby # Example code to charge a customer token = params[:stripeToken] amount = 1000 # Amount in cents begin charge = Stripe::Charge.create( amount: amount, currency: 'usd', source: token, description: 'Example Charge' ) # Handle successful payment rescue Stripe::CardError => e # Handle card error rescue Stripe::InvalidRequestError => e # Handle invalid request error rescue Stripe::AuthenticationError => e # Handle authentication error rescue Stripe::StripeError => e # Handle generic Stripe error end
2.1.6. Handle Webhooks:
Implement webhook handlers to receive and process events from Stripe, such as successful payments or refunds.
By integrating Stripe into your e-commerce application, you enable secure and hassle-free transactions for your customers, enhancing their shopping experience.
3. Efficient Catalog Management
Effective catalog management is vital for showcasing your products and enabling customers to find and purchase items with ease. A well-organized catalog improves user experience and boosts sales. Let’s explore how to implement efficient catalog management in your Ruby e-commerce application.
3.1. Define Product Model:
Create a model to represent your products. This model should include attributes such as name, description, price, and image URL.
ruby class Product < ActiveRecord::Base validates :name, presence: true validates :price, numericality: { greater_than: 0 } end
3.2. Create Product Views:
Design views to display your products, including product listings and individual product pages. Utilize HTML, CSS, and ERB (Embedded Ruby) templates to create visually appealing and responsive product displays.
3.3. Implement Product Search:
Allow customers to search for products by integrating a search functionality. You can use libraries like Ransack to simplify the search implementation.
ruby # In your controller def index @q = Product.ransack(params[:q]) @products = @q.result(distinct: true) end
3.4. Manage Categories and Tags:
Categorize your products by creating categories and assigning them to products. Implement tags to enable more granular product filtering.
ruby class Category < ActiveRecord::Base has_many :products end class Product < ActiveRecord::Base belongs_to :category has_many :product_tags has_many :tags, through: :product_tags end
3.5. Implement Cart Functionality:
Enable customers to add products to their cart and proceed to checkout. Create a cart model and associated actions to manage the cart items.
ruby class Cart < ActiveRecord::Base has_many :cart_items has_many :products, through: :cart_items end class CartItem < ActiveRecord::Base belongs_to :cart belongs_to :product end
Conclusion
Building e-commerce applications with Ruby involves integrating essential features like payment gateways and efficient catalog management. Payment gateways, such as Stripe, ensure secure and seamless transactions, enhancing the customer experience. Catalog management encompasses defining product models, creating views, implementing search and filtering, and managing categories and tags. By incorporating these elements effectively, you can develop a robust and user-friendly e-commerce application that attracts customers and drives sales. Remember to stay updated with the latest developments in Ruby and e-commerce trends to continually optimize your application for success. Happy coding!
Table of Contents
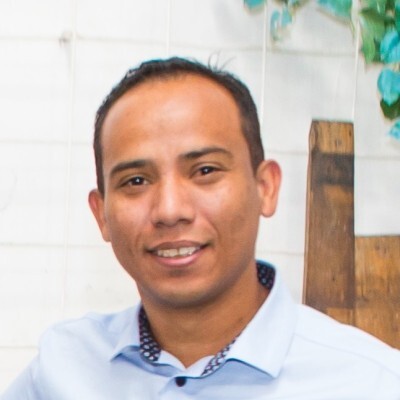
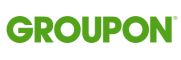