Building Micro Frontends with Ruby: Modular UI Architectures
In today’s rapidly evolving web development landscape, building efficient and scalable frontend applications is a constant challenge. The traditional monolithic approach to frontend development often results in codebases that are difficult to manage, prone to bottlenecks, and challenging to scale. This is where micro frontends come into play, offering a solution that promotes modularization, reusability, and maintainability. In this blog post, we’ll delve into the world of micro frontends and explore how Ruby can be a powerful ally in crafting modular UI architectures that make development and maintenance a breeze.
Table of Contents
1. Understanding Micro Frontends
Micro frontends are an architectural pattern that mirrors the principles of microservices in the frontend world. Just as microservices involve breaking down complex backend systems into smaller, manageable services, micro frontends apply the same concept to the frontend layer. The idea is to split a frontend application into smaller, self-contained units, each responsible for a specific part of the user interface. This enables teams to work independently on different parts of the application, using different technologies if necessary.
2. Benefits of Micro Frontends
2.1. Modularity and Reusability
Micro frontends emphasize modularity, allowing developers to build UI components that can be reused across different parts of the application or even in different projects. This modular approach simplifies maintenance, as changes to one component don’t necessarily affect others.
2.2. Team Scalability
Large frontend monoliths can become a bottleneck for development teams. Micro frontends enable multiple teams to work concurrently on different parts of the application, reducing merge conflicts and promoting parallel development.
2.3. Technology Diversity
Different parts of an application might require different technologies for optimal performance. Micro frontends allow developers to choose the best tools for each specific use case, resulting in a more efficient and performant application.
2.4. Independent Deployment
Micro frontends can be independently deployed, enabling teams to release updates or bug fixes for a specific part of the application without affecting the entire system. This promotes faster iteration and reduces the risk associated with large deployments.
3. Implementing Micro Frontends with Ruby
Ruby, a dynamic and expressive programming language, can be an excellent choice for building micro frontends due to its flexibility and developer-friendly syntax. Let’s explore how Ruby can be leveraged to implement micro frontends effectively.
3.1. Component-based Architecture with Ruby Gems
Ruby Gems provide a convenient way to package and distribute Ruby code. By creating custom gems for UI components, you can encapsulate the functionality and styling of a component into a reusable package. This gem can then be easily integrated into different micro frontend projects, maintaining consistency across applications.
Here’s a simplified example of creating and using a UI component gem:
ruby # Creating a gem for a Button component # button_component.gemspec Gem::Specification.new do |spec| spec.name = 'button_component' spec.version = '1.0.0' spec.summary = 'Reusable Button Component' spec.authors = ['Your Name'] spec.email = ['your@email.com'] spec.files = Dir.glob('lib/**/*') end # Defining the Button component # lib/button_component.rb module ButtonComponent class Button def render(text) "<button class='button'>#{text}</button>" end end end ruby # Using the Button component gem in a micro frontend project # main_app.rb require 'button_component' button = ButtonComponent::Button.new button_markup = button.render('Click me') puts button_markup
3.2. Ruby on Rails for Micro Frontends
Ruby on Rails, a popular web application framework, can also be adapted for micro frontend architectures. Each micro frontend can be developed as a separate Rails application, providing isolation and autonomy to different parts of the UI.
In this approach, a “shell” application acts as the entry point for users, while the individual micro frontends are mounted as Rails engines within the shell. This separation enables independent development, deployment, and scaling of micro frontends.
Here’s a high-level overview of setting up micro frontends with Ruby on Rails:
3.2.1. Create Shell Application
Create a new Rails application that serves as the shell for the micro frontends. This application will handle routing and serve as the entry point.
3.2.2. Develop Micro Frontends
For each micro frontend, create a separate Rails engine. These engines encapsulate the UI components and logic specific to that frontend.
3.2.3. Mount Micro Frontends
In the shell application’s routes.rb file, mount the micro frontends as Rails engines. This enables the shell to delegate requests to the appropriate micro frontend engine.
3.2.4. Shared Components
To maintain consistency across micro frontends, create a shared Ruby Gem that contains common UI components, styles, and utilities. This gem can be imported and used across different micro frontend projects.
3.3. Communication Between Micro Frontends
While micro frontends offer numerous advantages, they also introduce challenges related to communication and coordination between different parts of the application. Here are a couple of strategies to facilitate communication between micro frontends:
3.3.1. Cross-Micro Frontend Events
Implement a cross-micro frontend event bus using a library like PUB/SUB to facilitate communication between different parts of the application. When a user interacts with one micro frontend, events can be published to the event bus, which other micro frontends can subscribe to and react accordingly.
3.3.2. Shared API Contracts
Define and maintain a clear API contract that outlines the communication interfaces between micro frontends. This contract can be shared as a document or defined using tools like Swagger. By adhering to a shared API contract, micro frontends can interact seamlessly regardless of the technology stack used.
Conclusion
The era of monolithic frontend applications is gradually giving way to the micro frontend approach, which offers improved scalability, modularity, and reusability. Ruby, with its versatility and developer-friendly syntax, can be a powerful tool for building micro frontends that enhance development efficiency and maintainability.
By adopting a component-based architecture using Ruby Gems, leveraging Ruby on Rails for building separate micro frontend applications, and implementing effective communication strategies, developers can harness the full potential of micro frontend architectures. Embracing these principles can lead to a more agile development process, faster deployment cycles, and a smoother user experience overall.
In the ever-evolving landscape of web development, embracing innovative approaches like micro frontends is key to staying ahead of the curve. So, why not explore the possibilities of building modular UI architectures with Ruby and unlock a new realm of frontend development possibilities? Your users and development team will thank you for it.
Table of Contents
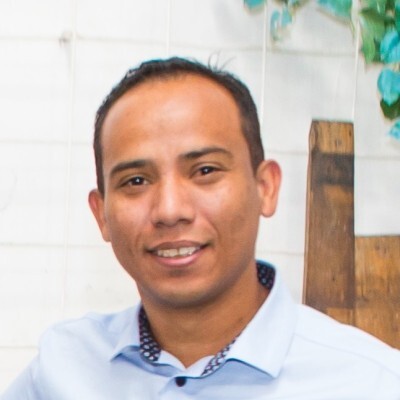
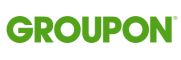