Building Recommendation Systems with Ruby: Personalized User Experiences
In today’s digital landscape, user experiences are at the forefront of every successful application. Whether it’s an e-commerce platform, a content streaming service, or a social media app, users expect tailored recommendations that cater to their unique interests and preferences. This is where recommendation systems come into play, leveraging data and algorithms to provide personalized suggestions that keep users engaged and satisfied.
Table of Contents
In this guide, we’ll dive into the world of building recommendation systems using the power of Ruby. We’ll explore various techniques and approaches that empower developers to create personalized user experiences. From collaborative filtering to content-based recommendations, we’ll cover the key concepts and provide code samples to help you get started on your journey to crafting intelligent recommendation systems.
1. Understanding Recommendation Systems
1.1. What are Recommendation Systems?
Recommendation systems are algorithms designed to suggest relevant items or content to users based on their preferences and behaviors. These systems play a crucial role in enhancing user engagement and satisfaction by providing personalized experiences. Whether it’s suggesting products that a customer might like, recommending movies based on viewing history, or proposing friends to connect with on a social platform, recommendation systems are at work behind the scenes.
1.2. Importance of Personalization
Personalization is the key to capturing user interest and retaining their attention. Generic, one-size-fits-all recommendations are no longer effective in today’s competitive digital landscape. Users are more likely to engage with platforms that understand their tastes and preferences, leading to increased user retention, higher conversion rates, and improved user satisfaction.
2. Types of Recommendation Systems
There are several approaches to building recommendation systems, each with its own strengths and limitations. Let’s explore the main types:
2.1. Collaborative Filtering
Collaborative filtering relies on user behavior and preferences to make recommendations. It assumes that users who have similar behaviors or interests in the past will continue to have similar preferences in the future. Collaborative filtering can be further divided into user-based and item-based methods.
2.2. User-Based Collaborative Filtering
In user-based collaborative filtering, recommendations are made based on the behaviors of similar users. For example, if User A and User B have both liked similar movies, the system might recommend a movie liked by User A to User B.
2.3. Item-Based Collaborative Filtering
Item-based collaborative filtering focuses on the similarity between items. If Item X is frequently purchased by users who have also purchased Item Y, the system might suggest Item Y to a user who has shown interest in Item X.
2.4. Content-Based Filtering
Content-based filtering recommends items based on their attributes and features. It creates user profiles based on the characteristics of items the user has interacted with in the past. For instance, if a user has watched and liked several action movies, the system might suggest more action-packed films.
2.5. Hybrid Methods
Hybrid recommendation systems combine multiple approaches to improve recommendation quality. By leveraging both collaborative and content-based methods, these systems can provide more accurate and diverse suggestions.
3. Getting Started with Ruby for Recommendations
To start building recommendation systems with Ruby, you’ll need to set up your development environment and gather relevant data.
3.1. Setting Up Your Environment
First, make sure you have Ruby installed on your system. You can use tools like RVM or rbenv to manage your Ruby versions. Additionally, you might want to explore RubyGems, the package manager for Ruby, to find libraries that can assist in recommendation system development.
3.2. Collecting and Preprocessing Data
Data is the foundation of recommendation systems. Collect user interactions, preferences, and item attributes to create meaningful recommendations. Preprocessing data might involve cleaning, transforming, and organizing it for effective use in your recommendation algorithms.
4. Collaborative Filtering with Ruby
Collaborative filtering techniques can be implemented using Ruby to create personalized recommendations.
4.1. User-Based Collaborative Filtering
User-based collaborative filtering involves finding similar users and recommending items that those similar users have liked. Here’s a simplified example using Ruby:
ruby def user_based_recommendations(user_id, user_data, similarity_matrix) similar_users = find_similar_users(user_id, similarity_matrix) recommendations = [] similar_users.each do |similar_user| user_data[similar_user].each do |item_id, rating| recommendations << item_id unless user_data[user_id].key?(item_id) end end recommendations end
4.2. Item-Based Collaborative Filtering
Item-based collaborative filtering focuses on item similarity to make recommendations. Here’s a basic implementation snippet:
ruby def item_based_recommendations(user_id, user_data, item_similarity_matrix) user_ratings = user_data[user_id] recommendations = {} user_ratings.each do |item_id, rating| item_similarity_matrix[item_id].each do |related_item, similarity| next if user_ratings.key?(related_item) recommendations[related_item] ||= 0 recommendations[related_item] += similarity * rating end end recommendations.sort_by { |item, score| -score }.map(&:first) end
5. Content-Based Recommendations in Ruby
Content-based filtering is another powerful technique to personalize recommendations.
5.1. Building User Profiles
Create user profiles based on the content attributes of items they have interacted with. For example, if users have rated movies, their profiles might include genres, directors, and actors they prefer.
5.2. Calculating Content Similarity
To make content-based recommendations, calculate the similarity between items based on their attributes. Here’s a simplified code snippet:
ruby def calculate_content_similarity(item1, item2) # Calculate similarity between item1 and item2 based on attributes end
6. Hybrid Recommendation Systems
Hybrid methods combine collaborative and content-based approaches for improved recommendations.
6.1. Combining Collaborative and Content-Based Approaches
A common approach is to assign weights to recommendations from different methods and combine them:
ruby def hybrid_recommendations(user_id, collaborative_recommendations, content_based_recommendations, weights) hybrid_recommendations = {} collaborative_recommendations.each do |item| hybrid_recommendations[item] ||= 0 hybrid_recommendations[item] += weights[:collaborative] end content_based_recommendations.each do |item| hybrid_recommendations[item] ||= 0 hybrid_recommendations[item] += weights[:content_based] end hybrid_recommendations.sort_by { |item, score| -score }.map(&:first) end
7. Evaluating and Optimizing Recommendation Systems
To ensure your recommendation system is effective, you need to evaluate and optimize its performance.
7.1. Metrics for Performance Evaluation
Common metrics include precision, recall, and Mean Average Precision (MAP). These metrics help you measure the accuracy and relevance of your recommendations.
7.2. A/B Testing and Continuous Improvement
Implement A/B testing to compare different recommendation algorithms and fine-tune your system. Regularly update your algorithms based on user feedback and changing preferences.
8. Implementing Real-Time Recommendations
Real-time recommendations involve adjusting suggestions based on user interactions as they happen.
8.1. Incorporating User Interaction in Real Time
Update user profiles and recommendation models in real time as users interact with your platform. This ensures that recommendations remain relevant and up to date.
8.2. Scalability and Performance Considerations
As your user base grows, consider the scalability of your recommendation system. Distributed computing and caching mechanisms can help maintain system performance.
9. Ethical Considerations in Recommendations
Building recommendation systems comes with ethical responsibilities.
9.1. Privacy and Data Security
Safeguard user data and ensure compliance with data protection regulations. Implement anonymization techniques and provide transparent privacy policies.
9.2. Avoiding Bias and Filter Bubbles
Mitigate bias by diversifying recommendations and exposing users to a variety of content. Prevent filter bubbles by occasionally introducing novel items to users.
10. Future Trends in Recommendation Systems
Recommendation systems continue to evolve with advancements in machine learning and AI.
10.1. Machine Learning and AI Advancements
Deep learning techniques, such as neural networks, are enhancing recommendation accuracy. Reinforcement learning and natural language processing are also contributing to more sophisticated recommendations.
10.2. Contextual and Location-Based Recommendations
As technology improves, recommendations will become more context-aware, considering factors like user location, time of day, and social context.
Conclusion
In conclusion, recommendation systems are pivotal in creating personalized user experiences that drive engagement and satisfaction. By harnessing the capabilities of Ruby and understanding various recommendation techniques, developers can craft intelligent systems that cater to users’ unique preferences. Whether you’re building an e-commerce platform, a music streaming service, or a news aggregator, the principles and code examples covered in this guide provide a solid foundation for building recommendation systems that enhance user interactions and keep them coming back for more.
Table of Contents
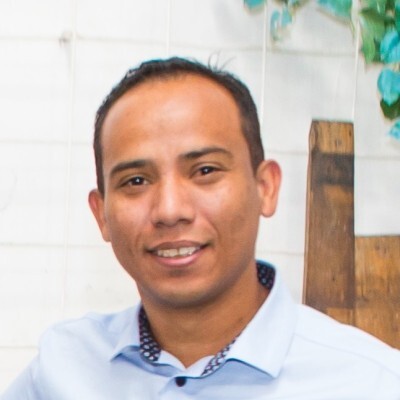
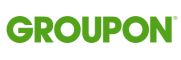