Building Serverless Applications with Ruby: Deploying on Cloud Platforms
Serverless architecture has gained immense popularity in recent years for its ability to simplify application development and reduce operational overhead. One of the key benefits of serverless computing is its support for multiple programming languages, including Ruby. In this blog post, we will delve into the world of serverless applications with Ruby and explore how to deploy them on various cloud platforms.
Table of Contents
1. What is Serverless Computing?
Before we dive into building serverless applications with Ruby, let’s first understand what serverless computing is all about.
1.1. Understanding Serverless Computing
Serverless computing, often referred to as Function as a Service (FaaS), is a cloud computing model that allows developers to build and run applications without worrying about managing servers or infrastructure. In this model, developers write code in the form of functions, and cloud providers take care of the underlying infrastructure, scaling, and execution of these functions in response to events or HTTP requests.
Key characteristics of serverless computing include:
- No Server Management: Developers don’t need to provision, configure, or manage servers. The cloud provider handles all server-related tasks.
- Automatic Scaling: Serverless platforms automatically scale the number of function instances in response to the workload, ensuring optimal performance and cost-efficiency.
- Pay-as-You-Go Pricing: With serverless, you only pay for the actual execution time and resources used by your functions, making it cost-effective for both small and large applications.
- Event-Driven: Serverless functions are triggered by events, such as HTTP requests, database changes, or messages from queues, making it ideal for building event-driven applications.
Now that we have a basic understanding of serverless computing, let’s explore how to build serverless applications using Ruby.
2. Building Serverless Applications with Ruby
Ruby is a dynamic, object-oriented programming language known for its simplicity and productivity. While it’s not as commonly associated with serverless development as languages like JavaScript or Python, Ruby enthusiasts can still leverage the power of serverless computing. Let’s walk through the steps to build serverless applications with Ruby and deploy them on popular cloud platforms.
2.1. Prerequisites
Before we begin, ensure you have the following prerequisites in place:
- Ruby Installed: You should have Ruby installed on your local development environment. You can download it from the official Ruby website.
- Serverless Framework: Install the Serverless Framework, a popular open-source framework for building serverless applications. You can install it using npm with the following command:
shell npm install -g serverless
- Cloud Platform Account: Sign up for an account on the cloud platform you intend to use (e.g., AWS, Azure, Google Cloud).
3. Creating a Serverless Ruby Project
Let’s start by creating a new serverless Ruby project using the Serverless Framework. We’ll use AWS Lambda as the serverless compute service for this example, but you can adapt the approach to other cloud providers as well.
Step 1: Initialize a New Serverless Project
Open your terminal and run the following commands to create a new serverless project:
shell serverless create --template aws-ruby --path my-serverless-ruby-app cd my-serverless-ruby-app
This will create a new directory for your project and set up a basic serverless configuration file (serverless.yml) with the AWS Lambda template.
Step 2: Define Your Serverless Functions
In the serverless.yml file, you can define your serverless functions and their configurations. Here’s an example of a simple Ruby function that responds to an HTTP request:
yaml functions: hello: handler: handler.hello events: - http: path: hello method: get
In this example, we define a function called hello that responds to HTTP GET requests on the /hello path. The handler value specifies the Ruby function that will be executed when the HTTP endpoint is triggered.
Step 3: Write Your Ruby Function
Create a handler.rb file in your project directory and define the hello function:
ruby def hello(event:, context:) { statusCode: 200, body: JSON.generate('Hello, Serverless Ruby!') } end
This simple Ruby function takes two arguments, event and context, and returns a response with a status code of 200 and a JSON message.
Step 4: Deploy Your Serverless Application
Once you’ve defined your serverless function, it’s time to deploy your application to the cloud. Run the following command to deploy your Ruby function to AWS Lambda:
shell serverless deploy
This command packages and deploys your Ruby code to AWS Lambda, creating the necessary resources and setting up the HTTP endpoint.
4. Deploying Ruby Serverless Applications on Different Cloud Platforms
Now that you’ve deployed a serverless Ruby application on AWS Lambda, let’s explore how to deploy it on other popular cloud platforms.
4.1. Deploying to Azure Functions
Azure Functions is Microsoft’s serverless compute service. To deploy your Ruby serverless application on Azure Functions, follow these steps:
Step 1: Install Azure Functions Core Tools
Install the Azure Functions Core Tools, which provides a set of command-line tools for working with Azure Functions:
shell npm install -g azure-functions-core-tools@3 --unsafe-perm true
Step 2: Create an Azure Functions Project
Create a new Azure Functions project with the following command:
shell func init my-azure-ruby-app
This will initialize a new Azure Functions project in the my-azure-ruby-app directory.
Step 3: Create a Ruby Function
Inside your Azure Functions project directory, create a new Ruby function by running:
shell func new --language Ruby --name hello
This command will create a new Ruby function named hello in the project.
Step 4: Deploy to Azure Functions
Deploy your Ruby function to Azure Functions using the following command:
shell func azure functionapp publish my-azure-ruby-app
This command will package and deploy your Ruby code to Azure Functions, making it accessible via HTTP endpoints.
4.2. Deploying to Google Cloud Functions
Google Cloud Functions is Google’s serverless compute service. To deploy your Ruby serverless application on Google Cloud Functions, follow these steps:
Step 1: Install Google Cloud SDK
Install the Google Cloud SDK, which provides the tools needed to deploy and manage Google Cloud resources:
shell curl -O https://dl.google.com/dl/cloudsdk/channels/rapid/downloads/google-cloud-sdk-345.0.0-linux-x86_64.tar.gz tar -xzf google-cloud-sdk-345.0.0-linux-x86_64.tar.gz ./google-cloud-sdk/install.sh
Step 2: Authenticate with Google Cloud
Authenticate with your Google Cloud account by running:
shell gcloud auth login
Follow the prompts to log in to your Google Cloud account.
Step 3: Create a Ruby Function
Create a new Ruby function in your Google Cloud Functions project by running:
shell gcloud functions deploy hello --runtime ruby27 --trigger-http
This command deploys your Ruby code to Google Cloud Functions and sets up an HTTP trigger.
5. Best Practices for Serverless Ruby Development
While deploying serverless Ruby applications on various cloud platforms is a great way to leverage the benefits of serverless computing, there are some best practices you should follow to ensure efficient development and maintenance of your applications.
5.1. Keep Functions Small and Focused
Break down your application into small, focused functions. Each function should do one thing and do it well. This approach makes your code more maintainable and easier to test.
5.2. Use External Libraries Sparingly
Serverless platforms may have limitations on the size of deployment packages. Be mindful of the size of your deployment package, and only include the external libraries that are essential for your functions.
5.3. Optimize Cold Starts
Cold starts can impact the latency of your serverless functions. To minimize cold start times, consider using provisioned concurrency (if available on your cloud platform) and optimizing your code for faster initialization.
5.4. Implement Error Handling
Implement robust error handling in your functions. Log errors and use appropriate error responses to provide meaningful feedback to clients.
5.5. Monitor and Debug
Use cloud platform monitoring and debugging tools to gain insights into the performance and behavior of your serverless functions. This can help you identify and address issues proactively.
5.6. Secure Your Functions
Ensure that your serverless functions are secure by following best practices for authentication, authorization, and data protection. Use environment variables for sensitive configuration values.
5.7. Testing and CI/CD
Implement automated testing for your serverless functions and set up a continuous integration and continuous deployment (CI/CD) pipeline to streamline development and deployment processes.
Conclusion
Building serverless applications with Ruby opens up a world of possibilities for Ruby developers. With the right tools and best practices in place, you can create efficient and scalable serverless applications that run on various cloud platforms, such as AWS Lambda, Azure Functions, and Google Cloud Functions.
Remember that serverless computing is not a one-size-fits-all solution, and the choice of cloud platform depends on your specific requirements and preferences. Whether you’re building web APIs, processing data, or creating event-driven applications, serverless Ruby can be a valuable addition to your development toolkit.
So, roll up your sleeves, start experimenting, and unleash the power of serverless Ruby to build the next generation of cloud-native applications.
In this blog post, we’ve explored the world of serverless computing with Ruby, including how to create serverless Ruby applications and deploy them on popular cloud platforms. We’ve also discussed best practices for efficient serverless Ruby development. Now, it’s time for you to dive in, experiment, and start building your own serverless Ruby applications. Happy coding!
Table of Contents
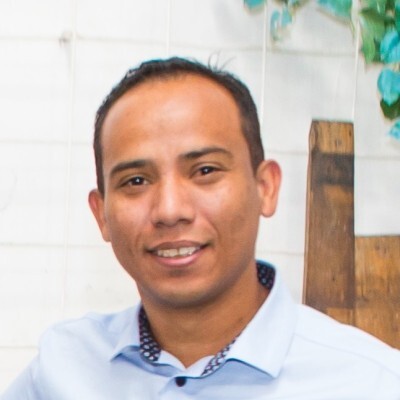
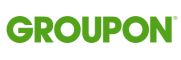