Building Voice Assistants with Ruby: Developing Conversational AI
In the age of smart homes, virtual assistants, and the ever-growing demand for seamless user experiences, voice assistants have become a prominent aspect of our daily lives. Whether it’s Siri, Google Assistant, or Alexa, voice-activated AI has revolutionized the way we interact with technology.
Table of Contents
But have you ever wondered how these voice assistants are created? How does a simple voice command trigger a series of actions? The answer lies in the world of Conversational AI, and in this blog, we’ll explore how to build your own voice assistant using Ruby.
1. Understanding Voice Assistants and Conversational AI
1.1. What is a Voice Assistant?
A voice assistant is a software application that uses speech recognition, natural language processing, and text-to-speech conversion to understand and respond to voice commands or questions from users. These assistants can perform various tasks, such as setting reminders, answering questions, controlling smart devices, and more.
Voice assistants have evolved into powerful tools that streamline our interactions with technology, making it easier to access information and perform tasks. Building your own voice assistant allows you to customize its capabilities and integrate it with your own applications.
1.2. The Role of Conversational AI
Conversational AI is the underlying technology that powers voice assistants. It focuses on enabling natural, human-like conversations between humans and machines. Key components of conversational AI include:
- Natural Language Processing (NLP): NLP techniques are used to understand and interpret human language. This involves tasks like language recognition, sentiment analysis, and intent recognition.
- Speech Recognition: Speech recognition technology converts spoken language into text, making it possible for machines to understand and process voice input.
- Text-to-Speech (TTS) Conversion: TTS technology transforms text into spoken language, allowing machines to respond to users in a human-like voice.
Now that we’ve established the fundamentals, let’s dive into how Ruby can play a pivotal role in building voice assistants.
2. Setting the Foundation with Ruby
2.1. Why Ruby?
Ruby is a dynamic, open-source programming language known for its simplicity and productivity. While it may not be the first choice for all AI development, its elegant syntax and extensive ecosystem make it a viable option for building voice assistants. Here’s why Ruby can be an excellent choice:
- Readability: Ruby’s clean and intuitive syntax is easy to read and write, making it accessible for developers of all levels.
- Community and Gems: Ruby boasts a vibrant community and a vast collection of gems (libraries) that can expedite the development process. Gems like Sinatra and Rails can help create web interfaces for your voice assistant.
- Integration: Ruby’s ability to integrate seamlessly with other technologies and APIs allows you to incorporate various functionalities into your voice assistant.
2.2. Ruby’s Role in Conversational AI
Ruby may not be as commonly associated with AI as Python, but it can serve as a strong foundation for building conversational AI. By harnessing the power of Ruby, you can create voice assistants that understand and respond to natural language, making them more user-friendly and engaging.
In the next sections, we’ll delve into the key concepts of voice assistant development and the tools and libraries available to simplify the process.
3. Key Concepts in Voice Assistant Development
Before we start writing code, it’s crucial to understand the key concepts that underpin voice assistant development. These concepts form the building blocks of a functional voice assistant:
3.1. Natural Language Processing (NLP)
NLP is at the heart of conversational AI. It enables machines to understand and interpret human language, allowing them to recognize user intent, extract information, and generate appropriate responses. NLP encompasses several subfields, including:
- Intent Recognition: Identifying the user’s intention behind a command or query.
- Named Entity Recognition: Extracting specific entities such as dates, locations, and names from text.
- Sentiment Analysis: Determining the emotional tone of a user’s message.
- In Ruby, you can leverage libraries like ‘nlp’ and ‘stanford-nlp’ to incorporate NLP capabilities into your voice assistant.
3.2. Speech Recognition
Speech recognition is the process of converting spoken language into text. It plays a crucial role in understanding user input. While there are specialized speech recognition services like Google Cloud Speech-to-Text, you can also use the ‘ruby_speech_recognition’ gem to implement this functionality in Ruby.
3.3. Text-to-Speech (TTS) Conversion
TTS technology allows your voice assistant to respond to users in a natural, human-like voice. This is achieved by converting text responses into audio. Popular TTS services, such as Amazon Polly or Google Text-to-Speech, can be integrated with your Ruby application to achieve this.
Now that we’ve covered the fundamental concepts, let’s explore the tools and libraries that Ruby developers can utilize to build voice assistants effectively.
4. Tools and Libraries
4.1. Ruby SpeechRecognition
Ruby SpeechRecognition is a gem that provides access to various speech recognition engines, making it easier to capture and process voice input. It supports multiple backends, including Google Web Speech API and pocketsphinx. Here’s how you can get started with Ruby SpeechRecognition:
ruby # Install the gem gem install ruby_speech_recognition # Sample code for speech recognition require 'ruby_speech_recognition' recognizer = RubySpeechRecognition::Recognizer.new recognizer.on(:ready_for_speech) { puts 'Ready for speech recognition' } recognizer.on(:speech) do |text| puts "You said: #{text}" # Perform actions based on the recognized speech end Recognizer.listen
This code initializes a speech recognizer, listens for speech input, and executes actions based on the recognized text.
4.2. Wit.ai
Wit.ai is a natural language processing platform owned by Facebook. It offers powerful NLP capabilities, including intent recognition and entity extraction. You can integrate Wit.ai with your Ruby voice assistant using the ‘wit-ruby’ gem:
ruby # Install the gem gem install wit-ruby # Sample code for using Wit.ai require 'wit' client = Wit.new(access_token: 'YOUR_ACCESS_TOKEN') response = client.message('Recognize this text') puts "Intent: #{response['intents'][0]['name']}"
Integrating Wit.ai into your project allows you to enhance the understanding of user commands.
4.3. Google Cloud Speech-to-Text
Google Cloud offers a comprehensive suite of AI and machine learning services, including speech recognition through the Speech-to-Text API. You can use the ‘google-cloud-speech’ gem to access this service from your Ruby application:
ruby # Install the gem gem install google-cloud-speech # Sample code for Google Cloud Speech-to-Text require "google/cloud/speech" speech = Google::Cloud::Speech.new audio = speech.audio "path/to/audio/file.wav", language: "en-US" results = audio.recognize results.each do |result| puts "Transcript: #{result.transcript}" end
Google Cloud Speech-to-Text is a powerful tool for accurate speech recognition and transcription.
5. Building a Simple Voice Assistant
Now that we’ve covered the essentials, let’s embark on the journey of building a simple voice assistant in Ruby. This assistant will respond to basic voice commands. Here’s a step-by-step guide:
Step 1: Installing Dependencies
To begin, make sure you have the necessary gems installed:
- ruby_speech_recognition: For capturing voice input.
- google-cloud-speech: For speech recognition.
- google-cloud-text_to_speech: For generating voice output.
bash gem install ruby_speech_recognition google-cloud-speech google-cloud-text_to_speech
Step 2: Capturing Voice Input
Create a Ruby script to capture voice input using the Ruby SpeechRecognition gem:
ruby require 'ruby_speech_recognition' recognizer = RubySpeechRecognition::Recognizer.new recognizer.on(:speech) do |text| puts "You said: #{text}" # Add logic to process the command end recognizer.listen
This code initializes the recognizer and listens for voice input.
Step 3: Processing Voice Input
Next, enhance your voice assistant by adding logic to process voice input. You can use conditional statements to recognize specific commands:
ruby require 'ruby_speech_recognition' recognizer = RubySpeechRecognition::Recognizer.new recognizer.on(:speech) do |text| case text when 'What's the weather today?' # Fetch weather information when 'Tell me a joke.' # Deliver a humorous response else puts "I didn't understand that command." end end recognizer.listen
This code recognizes and responds to commands like asking for the weather or requesting a joke.
Step 4: Generating Voice Output
To make your voice assistant truly conversational, add text-to-speech (TTS) functionality. You can use the Google Cloud Text-to-Speech API to convert text responses into audio:
ruby require "google/cloud/text_to_speech" def text_to_speech(text) text_to_speech = Google::Cloud::TextToSpeech.new synthesis_input = { text: text } voice = { language_code: "en-US", name: "en-US-Wavenet-D" } audio_config = { audio_encoding: :MP3 } response = text_to_speech.synthesize_speech( input: synthesis_input, voice: voice, audio_config: audio_config ) File.open("response.mp3", "wb") do |file| file.write(response.audio_content) end system("mpg123 response.mp3") end
This code defines a text_to_speech function that generates audio from text and plays it using mpg123.
With these steps, you’ve created a simple voice assistant in Ruby. You can expand upon this foundation by adding more commands, integrating NLP for better understanding, and connecting with external services.
6. Enhancing Your Voice Assistant
Building a basic voice assistant is just the beginning. To create a powerful and user-friendly voice assistant, consider implementing the following enhancements:
6.1. Adding Dialog Flow
Implementing a dialog flow allows your voice assistant to engage in more natural conversations. Tools like Dialogflow by Google can be integrated into your Ruby application to handle multi-turn conversations and complex interactions.
6.2. Integration with Web Services
Connect your voice assistant to web services and APIs to perform actions such as checking the weather, controlling smart home devices, or retrieving information from the internet. Ruby’s versatility makes it easy to make HTTP requests and process JSON responses.
6.3. Handling Multiple Languages
Extend the language capabilities of your voice assistant to reach a broader audience. Implement multilingual support by using translation services like Google Translate and language-specific NLP models.
7. Challenges and Considerations
While building voice assistants can be exciting, there are several challenges and considerations to keep in mind:
7.1. Privacy and Security
Voice assistants often process sensitive information. Ensure that you prioritize user privacy and data security by implementing robust encryption, access controls, and data anonymization practices.
7.2. Continuous Learning and Improving
To stay competitive, voice assistants should continually learn and improve. Implement machine learning models to adapt to user preferences and evolving language patterns.
Conclusion
Building voice assistants with Ruby is an engaging and rewarding endeavor. By combining Ruby’s simplicity with the power of NLP and speech recognition, you can create voice assistants that are both functional and user-friendly. Whether you’re developing a personal voice assistant or integrating one into your application, Ruby provides a strong foundation to get started.
As technology continues to advance, voice assistants will play an increasingly vital role in how we interact with the digital world. Embrace the possibilities of Conversational AI and voice assistants by diving into the world of Ruby development.
With the tools, libraries, and concepts explored in this blog, you have the knowledge to embark on your journey to build voice assistants and shape the future of user interaction. Happy coding!
Table of Contents
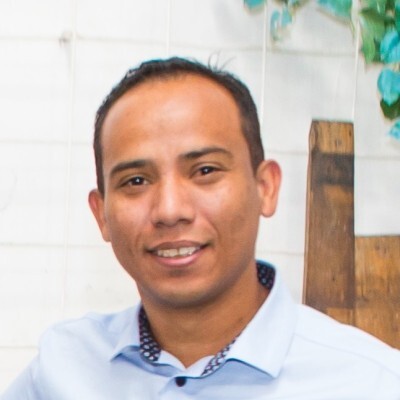
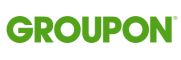