Creating Command-Line Tools with Ruby: From Scripting to Executables
Command-line tools play a vital role in automating tasks, performing system operations, and facilitating efficient workflows. Ruby, with its expressive syntax and extensive library support, is a powerful language for building such tools. In this blog, we’ll explore the process of creating command-line tools using Ruby, starting from simple scripts and advancing to the creation of standalone executables.
Scripting Basics
To begin our journey, let’s create a basic Ruby script that can be executed from the command line. Open your favorite text editor and create a new file called my_script.rb. Here’s an example script that greets the user:
ruby #!/usr/bin/env ruby puts "Hello, World!"
Save the file and open your terminal. Navigate to the directory where you saved my_script.rb and execute the following command:
ruby my_script.rb
You should see the output Hello, World! displayed in the terminal. Congratulations! You’ve just created and executed your first Ruby script.
Accepting Command-Line Arguments
Now, let’s enhance our script to accept command-line arguments. Command-line arguments allow users to provide inputs or parameters to customize the behavior of the script. We can access these arguments using the ARGV constant, which is an array that contains the arguments passed to the script.
Update your my_script.rb file with the following code:
ruby #!/usr/bin/env ruby puts "Hello, #{ARGV[0]}!"
Save the changes and execute the script with an argument:
ruby my_script.rb John
The output will be Hello, John!. In this example, we accessed the first command-line argument provided (ARGV[0]) and used it to personalize the greeting.
Parsing Command-Line Options
While command-line arguments are useful, they have limitations when it comes to handling complex options or flags. To overcome this, we can leverage the power of Ruby gems like OptionParser to parse command-line options effortlessly.
Let’s modify our script to use OptionParser for handling options. Update your my_script.rb file with the following code:
ruby #!/usr/bin/env ruby require 'optparse' options = {} OptionParser.new do |opts| opts.banner = "Usage: my_script.rb [options]" opts.on("-n", "--name NAME", "Specify a name") do |name| options[:name] = name end opts.on("-h", "--help", "Prints help") do puts opts exit end end.parse! name = options[:name] || "World" puts "Hello, #{name}!"
Save the changes and execute the script:
css ruby my_script.rb --name John
The output will be Hello, John!. You can also provide the -h or –help option to see the available options and usage instructions.
Interacting with Users
In some cases, you may need to gather information from the user during script execution. Ruby provides several methods to interact with the user, such as gets, print, and puts. Let’s modify our script to prompt the user for input:
ruby #!/usr/bin/env ruby print "Enter your name: " name = gets.chomp puts "Hello, #{name}!"
Save the changes and execute the script:
ruby my_script.rb
The script will prompt you to enter your name, and after you provide the input, it will greet you accordingly.
External Dependencies and Gems
As your command-line tool grows in complexity, you may need to leverage external dependencies or Ruby gems to enhance its functionality. Bundler, a popular Ruby gem, helps manage gem dependencies for your project. Let’s explore how to set up and use Bundler.
First, install Bundler by executing the following command:
gem install bundler
Next, create a new file called Gemfile in the same directory as your script. Add the following content to specify your gem dependencies:
ruby source 'https://rubygems.org' gem 'my_gem'
Replace ‘my_gem’ with the name of the gem you want to use. Save the Gemfile.
Run the following command to install the gem and its dependencies:
bundle install
Now, you can require the gem in your script:
ruby #!/usr/bin/env ruby require 'my_gem' # Rest of your code
Ensure that the gem is installed and available on the system where you intend to run the script.
Testing Command-Line Tools
Testing is crucial to ensure the correctness and reliability of your command-line tools. Ruby provides various testing frameworks like RSpec and Minitest for this purpose. Let’s use RSpec to create a basic test suite for our command-line tool.
First, install the RSpec gem by running:
gem install rspec
Next, create a new directory called spec in the same directory as your script. Inside the spec directory, create a new file called my_script_spec.rb. Here’s an example test for our script:
ruby require 'rspec' require_relative '../my_script' RSpec.describe 'MyScript' do it 'greets the user' do expect { MyScript.run }.to output("Hello, World!\n").to_stdout end end
Save the file and run the tests using the following command:
bash rspec spec/my_script_spec.rb
You should see the test pass, indicating that your script is functioning correctly.
Packaging as Executables
To distribute your command-line tool more conveniently, you can package it as a standalone executable that users can run without having Ruby or any dependencies installed. Two popular Ruby gems, RubyGems and rake-compiler, facilitate this process.
First, ensure that the rubygems gem is installed by executing:
gem install rubygems
Next, install the rake-compiler gem by running:
gem install rake-compiler
Create a new file called Rakefile in your project directory with the following content:
ruby require 'rake/extensiontask' Rake::ExtensionTask.new('my_script') do |ext| ext.lib_dir = 'lib/my_script' ext.source_pattern = 'lib/my_script/**/*.{rb,c}' end
Replace ‘my_script’ with the name of your script. Save the Rakefile.
Run the following command to compile your script into an executable:
python rake compile
After successful compilation, you’ll find the executable file in the pkg directory. Users can now run your command-line tool without needing Ruby installed:
bash ./my_script
Conclusion
In this blog post, we explored the process of creating command-line tools with Ruby. We started with the basics of scripting and gradually progressed to handling command-line arguments, parsing options, interacting with users, managing external dependencies, testing, and packaging as standalone executables. Armed with this knowledge, you can now leverage Ruby’s power and flexibility to create efficient and versatile command-line tools tailored to your specific needs.
Remember, the journey doesn’t end here. Dive deeper into Ruby’s ecosystem, explore more gems, and continue honing your skills to create even more powerful command-line tools. Happy scripting!
Table of Contents
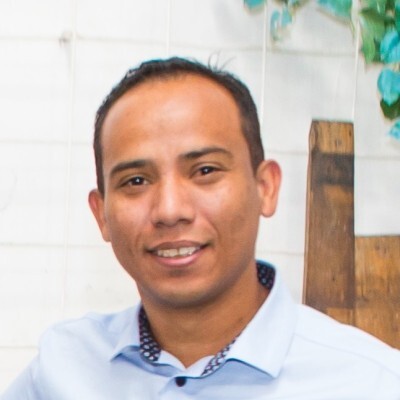
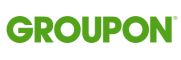