Ruby for Computer Vision: Image Processing and Object Recognition
In the realm of computer vision, the ability to interpret and manipulate images is essential. Whether you’re working on automating tasks, enhancing visual content, or building advanced AI systems, mastering image processing and object recognition can open up a world of possibilities. In this blog, we’ll explore how Ruby, a versatile and dynamic programming language, can be harnessed for these tasks.
Table of Contents
1. Why Choose Ruby for Computer Vision?
Before diving into the intricacies of image processing and object recognition with Ruby, let’s address the question of why one should consider Ruby for these tasks. Ruby is typically known for its simplicity, expressiveness, and ease of use. While it may not be the most commonly chosen language for computer vision, it still offers several compelling advantages:
1.1. Rapid Prototyping
Ruby’s clean and concise syntax allows developers to quickly prototype ideas and algorithms. This makes it an excellent choice for experimenting with computer vision concepts before committing to larger projects.
1.2. Extensive Libraries
Ruby boasts a rich ecosystem of libraries and gems that can be leveraged for computer vision tasks. The Ruby community actively contributes to these libraries, ensuring continuous improvements and updates.
1.3. Interoperability
Ruby can seamlessly integrate with other languages, such as Python and C++, which are popular choices for computer vision. This flexibility enables you to combine Ruby’s strengths with libraries and tools from other ecosystems.
Now that we’ve established the merits of Ruby for computer vision, let’s explore the practical aspects of image processing and object recognition using this versatile language.
2. Setting Up Your Environment
Before we start working on image processing and object recognition, it’s crucial to set up your development environment. Make sure you have Ruby installed on your system. You can check your Ruby version using the following command:
ruby ruby -v
If Ruby is not installed, you can download it from the official Ruby website or use a package manager like RVM for easy installation and version management.
Next, you’ll want to install essential gems that will help us with image processing and object recognition. One of the most popular libraries for this purpose is OpenCV. You can install it using the following command:
ruby gem install ruby-opencv
With Ruby and the necessary libraries in place, we can proceed with image processing and object recognition tasks.
3. Image Processing with Ruby
3.1. Loading an Image
To get started with image processing in Ruby, let’s load an image using the OpenCV library. We’ll also display it to verify that everything is set up correctly.
ruby require 'opencv' # Load an image image = OpenCV::IplImage.load('path_to_your_image.jpg') # Display the loaded image window = OpenCV::GUI::Window.new('Loaded Image') window.show(image)
Replace ‘path_to_your_image.jpg’ with the path to the image you want to load. Running this code will open a window displaying the loaded image.
3.2. Grayscale Conversion
Grayscale images are often used in computer vision tasks because they simplify processing while retaining essential information. Let’s convert our loaded image to grayscale.
ruby # Convert the image to grayscale gray_image = image.BGR2GRAY # Display the grayscale image gray_window = OpenCV::GUI::Window.new('Grayscale Image') gray_window.show(gray_image)
This code converts the loaded image to grayscale and displays it in a new window.
3.3. Applying Filters
Image processing often involves applying filters to enhance or modify images. We can use the OpenCV library to apply various filters, such as blurring and edge detection.
3.3.1. Gaussian Blur
ruby # Apply Gaussian blur with a 5x5 kernel blurred_image = gray_image.smooth(OpenCV::CV_GAUSSIAN, 5, 5) # Display the blurred image blurred_window = OpenCV::GUI::Window.new('Blurred Image') blurred_window.show(blurred_image)
3.3.2. Edge Detection (Canny)
ruby # Apply Canny edge detection canny_image = gray_image.canny(50, 150) # Display the edge-detected image canny_window = OpenCV::GUI::Window.new('Edge-Detected Image') canny_window.show(canny_image)
These examples showcase just a few of the many image processing capabilities of Ruby through the OpenCV library. Experiment with different filters and techniques to achieve the desired results for your specific task.
4. Object Recognition with Ruby
Object recognition involves identifying and locating specific objects or patterns within an image. We can achieve this using techniques like template matching and feature detection. Let’s explore template matching as an example.
4.1. Template Matching
Template matching is a straightforward yet effective technique for locating a template image within a larger image. In this example, we’ll search for a template within a larger image and highlight the match.
ruby # Load the larger image main_image = OpenCV::IplImage.load('path_to_larger_image.jpg') # Load the template image template = OpenCV::IplImage.load('path_to_template_image.jpg') # Perform template matching result = main_image.match_template(template) # Find the best match min_value, max_value, min_point, max_point = result.min_max_loc # Get the dimensions of the template image template_width = template.width template_height = template.height # Draw a rectangle around the matched area main_image.rectangle!(max_point, OpenCV::CvPoint.new(max_point.x + template_width, max_point.y + template_height), :color => OpenCV::CvColor::Red, :thickness => 2) # Display the main image with the match highlighted result_window = OpenCV::GUI::Window.new('Template Matching Result') result_window.show(main_image)
Replace ‘path_to_larger_image.jpg’ and ‘path_to_template_image.jpg’ with the paths to your larger image and the template image you want to find within it. This code will display the main image with a highlighted region where the template was found.
Conclusion
In this blog, we’ve explored the potential of Ruby for computer vision tasks, focusing on image processing and object recognition. Ruby’s simplicity and versatility, combined with libraries like OpenCV, make it a viable choice for developing computer vision applications.
As you delve deeper into the world of computer vision with Ruby, you’ll discover a vast array of techniques and possibilities. Whether you’re building a facial recognition system, automating quality control in manufacturing, or creating artistic filters for images, Ruby has the tools and libraries to support your endeavors.
Remember that computer vision is a vast and evolving field, so continuous learning and experimentation are key. As you gain experience, you’ll become proficient in harnessing the power of Ruby to solve complex visual tasks.
So, go ahead and start your journey into the exciting realm of computer vision with Ruby, and unlock the potential to create innovative and impactful solutions. Happy coding!
Table of Contents
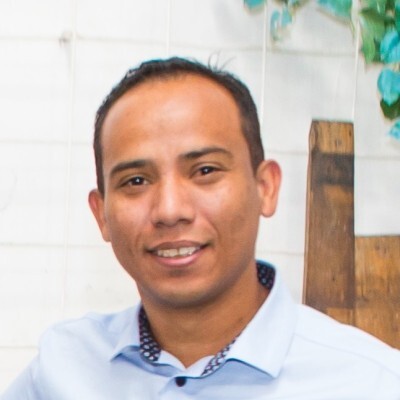
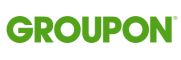