Concurrency in Ruby: Leveraging Threads and Fibers
Concurrency is a critical aspect of modern software development, enabling applications to execute multiple tasks simultaneously and improve overall performance. In Ruby, concurrency can be achieved through threads and fibers, two powerful tools that allow developers to leverage parallelism and asynchronous programming. This blog post will explore the concepts of threads and fibers in Ruby, their differences, and how they can be used to write efficient and responsive concurrent code.
Understanding Threads
Threads are lightweight execution units that enable concurrent execution within a single process. They allow multiple sections of code to execute simultaneously, taking advantage of the available CPU cores and speeding up program execution. Ruby provides excellent support for threads with its built-in Thread class.
Let’s take a look at a simple example that demonstrates the use of threads in Ruby:
ruby threads = [] # Create 5 threads 5.times do |i| threads << Thread.new do puts "Thread #{i} started" sleep(rand(1..5)) # Simulating some work puts "Thread #{i} finished" end end # Wait for all threads to complete threads.each(&:join) puts "All threads completed"
In this example, we create five threads that perform some work, simulated by a random sleep duration. Each thread outputs a message indicating its start and finish. The Thread.new block defines the code to be executed concurrently.
When running the code, you’ll observe that the threads start and finish in a non-deterministic order, as they are executed concurrently. This parallelism can significantly improve the overall performance of your application.
Thread Safety and Synchronization
While threads offer great concurrency benefits, they also introduce challenges related to thread safety and synchronization. When multiple threads access shared resources concurrently, issues like race conditions and data inconsistency can arise. Ruby provides various synchronization mechanisms to address these challenges.
One popular synchronization mechanism is the Mutex class. A Mutex, short for mutual exclusion, allows only one thread to access a resource at a time. Let’s modify our previous example to demonstrate the use of Mutex:
ruby require 'thread' mutex = Mutex.new counter = 0 # Create 5 threads 5.times do |i| Thread.new do mutex.synchronize do puts "Thread #{i} started" sleep(rand(1..5)) # Simulating some work counter += 1 puts "Thread #{i} finished (Counter: #{counter})" end end end # Wait for all threads to complete sleep(6) puts "All threads completed (Final Counter: #{counter})"
In this modified example, a Mutex is used to synchronize access to the counter variable. The mutex.synchronize block ensures that only one thread can modify the counter at a time. Running the code will demonstrate how the counter is safely incremented by each thread, preventing any race conditions.
While Mutex provides a simple and effective way to synchronize access to shared resources, it’s essential to use it judiciously to avoid potential performance bottlenecks. In some cases, other synchronization primitives like Semaphores or ConditionVariables might be more suitable depending on the specific requirements of your application.
Introducing Fibers
In addition to threads, Ruby also provides fibers, which are lightweight cooperative concurrency primitives. Unlike threads, fibers are not managed by the operating system but by the Ruby interpreter itself. Fibers allow you to achieve concurrency through cooperative multitasking, where control is explicitly transferred between fibers.
Let’s explore a simple example to understand how fibers work:
ruby fiber = Fiber.new do puts "Fiber started" Fiber.yield # Transfer control back to the caller puts "Fiber resumed" end puts "Program started" fiber.resume # Start the fiber puts "Program resumed" fiber.resume # Resume the fiber puts "Program completed"
In this example, we create a fiber using the Fiber.new constructor and define the code to be executed within the fiber using a block. The Fiber.yield statement transfers control back to the caller, allowing other parts of the program to execute. The fiber.resume method starts or resumes the execution of the fiber.
When you run this code, you’ll notice that the control flow jumps between the fiber and the program, resulting in the following output:
php Program started Fiber started Program resumed Fiber resumed Program completed
Fibers are particularly useful when you need fine-grained control over concurrency, such as implementing generators or iterators. Unlike threads, fibers do not have a built-in mechanism for parallelism, as they run within a single thread. However, they can achieve high levels of concurrency by scheduling their execution cooperatively.
Combining Threads and Fibers
Threads and fibers can be used together to harness the benefits of both parallelism and cooperative concurrency. By combining these two concurrency primitives, you can build highly responsive and efficient applications.
Consider the following example, where multiple fibers are executed within separate threads:
ruby threads = [] # Create 5 threads 5.times do |i| threads << Thread.new do fiber = Fiber.new do puts "Fiber #{i} started" Fiber.yield # Transfer control back to the caller puts "Fiber #{i} resumed" end puts "Thread #{i} started" fiber.resume puts "Thread #{i} resumed" fiber.resume puts "Thread #{i} finished" end end # Wait for all threads to complete threads.each(&:join) puts "All threads completed"
In this example, each thread contains its own fiber, and the control flow jumps between the fiber and the thread. Running the code will demonstrate the interleaved execution of fibers within separate threads, resulting in improved concurrency.
Conclusion
Concurrency is a crucial aspect of modern software development, enabling applications to leverage parallelism and enhance performance. In Ruby, threads and fibers provide powerful mechanisms for achieving concurrency. Threads offer parallel execution and are suitable for CPU-bound tasks, while fibers provide cooperative multitasking and are ideal for I/O-bound operations. By understanding the differences between threads and fibers and utilizing them effectively, you can write concurrent Ruby code that is both performant and responsive.
Table of Contents
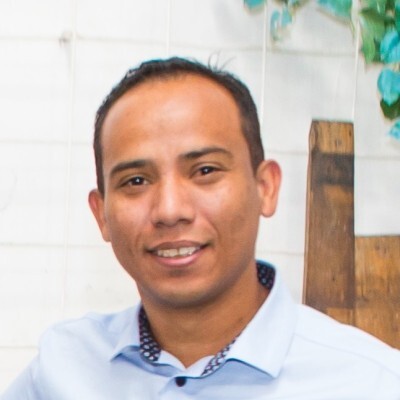
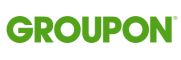