Exploring Ruby’s Core Features: From Strings to Symbols
Ruby, an elegant and robust programming language, is a favorite among developers for its simplicity and expressiveness. Its unique design philosophy, focusing on programmer happiness, has made it particularly appealing for businesses seeking to hire Ruby developers. This attention to programmer satisfaction has allowed Ruby to find a home in a wide variety of applications, from web development to data analysis and beyond.
Whether you’re a business aiming to hire Ruby developers or a budding programmer exploring new languages, understanding the core features of the language is crucial. In this blog post, we’ll be diving into some of Ruby’s core features, specifically focusing on strings and symbols. These are among the fundamental concepts that potential Ruby developers should master, and that businesses should be aware of when they hire Ruby developers.
Ruby Strings: A Flexible and Powerful Data Type
In Ruby, strings are mutable sequences of characters, enclosed within single (‘ ‘) or double (” “) quotes. Single-quoted strings are simple, allowing only for basic string content. Double-quoted strings, on the other hand, can interpolate variables and execute escape sequences.
String Basics
Ruby strings are incredibly flexible. They can be concatenated using the ‘+’ operator, repeated with the ‘*’ operator, and accessed like arrays for individual characters or slices. For example:
```ruby str1 = 'Hello' str2 = ' World' puts str1 + str2 # Output: Hello World str = 'Ho! ' puts str * 3 # Output: Ho! Ho! Ho! greeting = 'Hello, world!' puts greeting[0..4] # Output: Hello
String Interpolation
One of the standout features of Ruby’s strings is string interpolation. Interpolation allows the insertion of any Ruby expression within a string using #{}, which will be evaluated and converted into a string.
```ruby name = 'John' puts "Hello, #{name}!" # Output: Hello, John!
Note that interpolation only works in double-quoted strings.
String Methods
Ruby strings come with a plethora of built-in methods. You can transform them (`upcase`, `downcase`, `swapcase`, `capitalize`), strip whitespace (`strip`, `lstrip`, `rstrip`), replace portions (`gsub`), and much more.
```ruby str = " hello, world! " puts str.strip.capitalize # Output: Hello, world!
Ruby Symbols: Lightweight and Immutable
Symbols are one of Ruby’s unique features that sometimes puzzle newcomers. A symbol in Ruby is a lightweight, immutable object used as an identifier within code. They are defined using a colon (:), followed by the name of the symbol.
Symbol Basics
Here’s a quick demonstration of a symbol in action:
```ruby :symbol_example
Symbols are often used as keys in hashes because they’re more memory-efficient than strings. This is because each unique symbol is only stored once in memory, regardless of how many times it’s used.
```ruby hash = {apple: 1, banana: 2, orange: 3} puts hash[:apple] # Output: 1
String vs Symbol
While symbols may look like strings, they serve a different purpose and have distinct characteristics:
1. mmutability: Unlike strings, symbols are immutable. They can’t be altered once they’re created. This makes them ideal for identifiers or keys.
2. Singleton Behavior: Each symbol only exists once in memory. Two symbols with the same name are the same object. In contrast, two identical strings are different objects.
```ruby puts "string" == "string" # Output: true puts "string".object_id == "string".object_id # Output: false puts :symbol == :symbol # Output: true puts :symbol.object_id == :symbol.object_id # Output: true
Symbol Methods
Despite their simplicity, symbols support various useful methods similar to strings, such as `to_s` (convert to string), `to_sym` (convert string to symbol), and others. However, unlike strings, symbols can’t be mutated.
```ruby puts :symbol_example.to_s # Output: "symbol_example" puts "symbol_example".to_sym # Output: :symbol_example
Conclusion
Both strings and symbols are integral parts of Ruby’s core, each serving a unique purpose. This is one of the many reasons companies hire Ruby developers, to leverage the unique strengths of these features. Strings provide a mutable and flexible way to handle text data, while symbols act as immutable, efficient identifiers. Understanding these features is essential not only for those looking to write efficient and idiomatic Ruby code but also for those who hire Ruby developers, as it enhances communication and understanding of the development process.
Ruby’s emphasis on simplicity and expressiveness shines through its string and symbol handling, making it a joy to use for beginners and seasoned programmers alike. This ease and joy of use is why many companies choose to hire Ruby developers for their projects. Whether you’re crafting a quick script, developing a complex web application, or seeking to hire Ruby developers for your team, mastering these core features will enable you to leverage the power and elegance of Ruby to the fullest. Happy coding!
Table of Contents
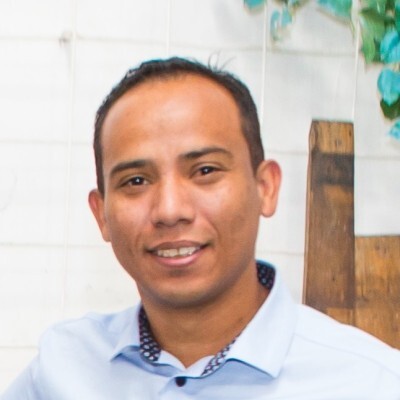
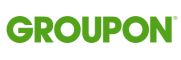