How to create a custom iterator in Ruby?
Creating a custom iterator in Ruby involves defining your own iterator method within a class. Custom iterators are useful when you want to traverse and manipulate elements in a specific way that isn’t covered by Ruby’s built-in iterators like `each` or `map`. To create a custom iterator, follow these steps:
- Define a Class: Start by defining a class that encapsulates the data structure you want to iterate over. This class should include an `initialize` method to set up the initial state and any necessary data structures.
- Define an Iterator Method: Inside your class, define a method that will serve as your custom iterator. This method should use a block to specify the actions to be performed on each element during iteration. You can yield elements to the block one by one.
- Yield Elements: Within your iterator method, use the `yield` keyword to pass each element to the block. This allows the block to process the element as desired. You can also pass additional information or modify the element before yielding it.
- Usage: Instantiate your class and call the custom iterator method, passing a block that defines the actions to be taken on each element. The block should receive the yielded element as its parameter.
Here’s a simple example of a custom iterator that iterates through an array of numbers and squares each element:
```ruby class CustomIterator def initialize(numbers) @numbers = numbers end def iterate index = 0 while index < @numbers.length yield @numbers[index] * @numbers[index] index += 1 end end end numbers = [1, 2, 3, 4, 5] iterator = CustomIterator.new(numbers) iterator.iterate do |squared| puts "Squared value: #{squared}" end ```
In this example, the `CustomIterator` class takes an array of numbers, and the `iterate` method squares each element and yields it to the provided block. When you call the `iterate` method with a block, it processes each element and prints the squared value.
Custom iterators are powerful because they allow you to tailor the iteration process to your specific requirements, making your code more expressive and flexible. You can create custom iterators for various data structures and tasks, enhancing the readability and maintainability of your Ruby code.
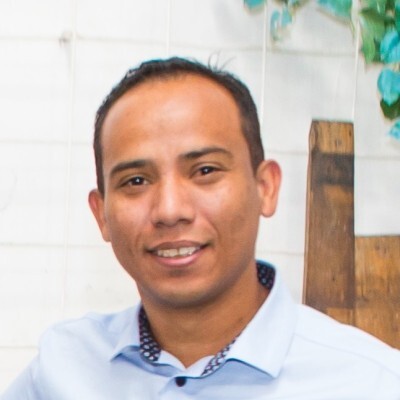
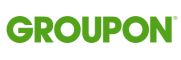