Creating 3D Applications with Ruby: Leveraging Graphics Libraries
Ruby, a versatile and dynamic programming language, has found its place in web development, scripting, and automation tasks. However, it might not be the first choice when you think about 3D graphics and game development. But did you know that Ruby can also be used to create immersive 3D applications, thanks to powerful graphics libraries? In this guide, we’ll explore the world of 3D graphics with Ruby and delve into the fascinating realm of 3D application development.
Table of Contents
1. Introduction to 3D Graphics with Ruby
1.1. Why Ruby for 3D Graphics?
Ruby’s primary strength lies in its simplicity and readability. It’s a fantastic choice for beginners and experienced developers alike. When it comes to 3D graphics, Ruby might not be as performance-oriented as some other languages like C++ or Python, but it compensates with its ease of use and the vast collection of libraries available for 3D rendering.
1.2. Essential Graphics Libraries for Ruby
Before we dive into creating 3D applications with Ruby, let’s get acquainted with some of the essential graphics libraries that make it all possible:
1.2.1. Gosu
Gosu is a 2D and 3D game development library for Ruby. It provides a simple interface for window management, input handling, and graphics rendering. While primarily designed for 2D games, you can create 3D games and applications by leveraging its OpenGL support.
1.2.2. Rubygame
Rubygame is a cross-platform multimedia library for game development with Ruby. It offers a high-level interface for creating 2D games, but it can also be used for basic 3D graphics rendering with OpenGL support.
1.2.3. Ruby-OpenGL
Ruby-OpenGL is a Ruby binding to the OpenGL 3D graphics library. It allows you to access the full power of OpenGL within your Ruby applications, making it a crucial tool for 3D graphics development.
1.2.4. Shoes
Shoes is a graphical toolkit for creating graphical user interfaces (GUIs) with Ruby. While not designed explicitly for 3D graphics, you can use it to build interactive 3D applications by combining it with other graphics libraries like OpenGL.
These libraries provide a solid foundation for 3D graphics development in Ruby. In this guide, we’ll primarily focus on using Gosu and Ruby-OpenGL to create a simple 3D application.
2. Setting Up Your Development Environment
2.1. Installing Required Libraries
Before you start creating 3D applications, you need to set up your development environment by installing the required libraries. We’ll focus on using Gosu and Ruby-OpenGL, so let’s get them installed.
2.1.1. Install Gosu:
bash gem install gosu
Install Ruby-OpenGL:
bash gem install ruby-opengl
3. Getting Familiar with Gosu
Gosu is a fantastic library for creating 2D and 3D applications in Ruby. It abstracts many of the complexities of OpenGL and provides a simple and intuitive interface for window management and input handling.
To get started with Gosu, create a new Ruby file (e.g., my_3d_app.rb) and require Gosu:
ruby require 'gosu'
Next, define a class that inherits from Gosu::Window. This class will represent your application’s window:
ruby class My3DApp < Gosu::Window def initialize super(800, 600, false) self.caption = "My 3D Application" end end
In this code, we create a window with a width of 800 pixels and a height of 600 pixels. The false parameter indicates that the window is not fullscreen. We also set a caption for the window.
Now, let’s create an instance of our My3DApp class and start the application:
ruby app = My3DApp.new app.show
This simple code sets up a window and runs your 3D application. Of course, this is just the beginning. To make a 3D application, we need to integrate OpenGL rendering into Gosu.
4. Integrating OpenGL with Gosu
Gosu allows us to leverage OpenGL for 3D rendering while still providing a user-friendly interface for window management and input. To do this, we can use the Gosu::Image class to load textures and draw them using OpenGL.
4.1. Loading Textures
To load a texture in Gosu, create an instance of Gosu::Image and pass the path to the image file:
ruby texture = Gosu::Image.new('path_to_texture.png')
Now that you have a texture loaded, you can use it for 3D rendering.
4.2. OpenGL Integration
To integrate OpenGL with Gosu, you need to define a custom drawing method within your My3DApp class. Override the draw method and use OpenGL functions to render your 3D objects.
ruby class My3DApp < Gosu::Window def initialize super(800, 600, false) self.caption = "My 3D Application" @texture = Gosu::Image.new('path_to_texture.png') end def draw gl do # Set up your OpenGL rendering here glEnable(GL_TEXTURE_2D) glBindTexture(GL_TEXTURE_2D, @texture.gl_tex_info.tex_name) glBegin(GL_QUADS) glTexCoord2d(0, 0); glVertex2f(0, 0) glTexCoord2d(1, 0); glVertex2f(@texture.width, 0) glTexCoord2d(1, 1); glVertex2f(@texture.width, @texture.height) glTexCoord2d(0, 1); glVertex2f(0, @texture.height) glEnd end end end
In this code, we first enable 2D textures and bind our loaded texture. Then, we use OpenGL functions to draw a textured quad (rectangle) on the screen.
This is a simple example of how you can integrate OpenGL with Gosu for 3D rendering. You can build upon this foundation to create more complex 3D scenes and animations.
5. Building a Simple 3D Scene
Now that we have a basic understanding of integrating OpenGL with Gosu, let’s take the next step and build a simple 3D scene. In this example, we’ll create a rotating 3D cube.
5.1. Creating a 3D Cube
To create a 3D cube, you need to define its vertices and faces. Here’s a simple representation of a cube’s vertices:
ruby vertices = [ [-1.0, -1.0, -1.0], [ 1.0, -1.0, -1.0], [ 1.0, 1.0, -1.0], [-1.0, 1.0, -1.0], [-1.0, -1.0, 1.0], [ 1.0, -1.0, 1.0], [ 1.0, 1.0, 1.0], [-1.0, 1.0, 1.0] ]
These coordinates represent the eight corners of the cube. Next, define the cube’s faces by specifying the vertex indices for each face:
ruby faces = [ [0, 1, 2, 3], # Front face [4, 5, 6, 7], # Back face [0, 1, 5, 4], # Bottom face [2, 3, 7, 6], # Top face [0, 3, 7, 4], # Left face [1, 2, 6, 5] # Right face ]
Now that we have the cube’s vertices and faces defined, we can start rendering it in our 3D application.
5.2. Rendering the 3D Cube
In your draw method, you can use OpenGL to render the cube. You’ll need to set up a projection matrix and a model-view matrix to position and rotate the cube.
Here’s a simplified example:
ruby class My3DApp < Gosu::Window def initialize super(800, 600, false) self.caption = "My 3D Application" @texture = Gosu::Image.new('path_to_texture.png') @rotation_angle = 0.0 end def draw gl do glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) glMatrixMode(GL_PROJECTION) glLoadIdentity gluPerspective(45, width.to_f / height, 0.1, 1000) glMatrixMode(GL_MODELVIEW) glLoadIdentity glTranslatef(0.0, 0.0, -5.0) glRotatef(@rotation_angle, 1.0, 1.0, 1.0) # Render the cube here using the defined vertices and faces # ... @rotation_angle += 1.0 end end end
In this code, we set up a perspective projection matrix and a model-view matrix to position and rotate the cube. Inside the draw method, you can use the vertices and faces defined earlier to render the cube.
As the @rotation_angle increases, the cube will continuously rotate in 3D space.
This example provides a basic understanding of how to create a 3D scene with Ruby and OpenGL. You can enhance and customize it to build more complex 3D applications and games.
Conclusion
Creating 3D applications with Ruby is an exciting endeavor, made possible by leveraging powerful graphics libraries like Gosu and Ruby-OpenGL. While Ruby may not be the first choice for high-performance 3D game development, it offers a friendly and accessible way to explore the world of 3D graphics.
In this guide, we’ve covered the essential steps to get started with 3D graphics in Ruby, from setting up your development environment to integrating OpenGL with Gosu and building a simple 3D scene. With this foundation, you can embark on your journey to create stunning 3D applications and games using Ruby.
Remember that 3D graphics is a vast and complex field, and there’s always more to learn. As you gain experience, you can explore advanced topics like shaders, physics simulations, and more to take your 3D Ruby applications to the next level. Happy coding, and may your 3D creations come to life!
Table of Contents
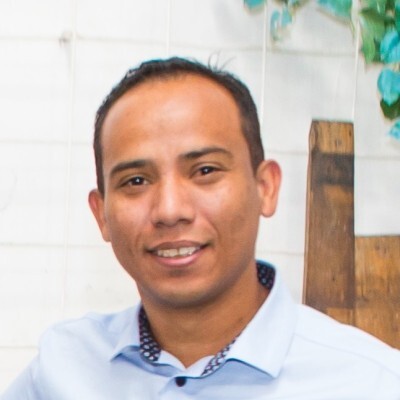
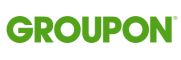