Ruby for Data Engineering: Processing and Transforming Large Datasets
In the realm of data engineering, the ability to process and transform large datasets efficiently is paramount. With the exponential growth of data in various industries, having tools that can handle this data deluge is essential. While languages like Python, Java, and Scala are often considered the go-to options for data engineering tasks, Ruby, with its elegant syntax and powerful libraries, can also play a significant role in this space. In this blog post, we’ll delve into the world of Ruby for data engineering, exploring how it can be leveraged for processing and transforming large datasets.
Table of Contents
1. Introduction to Ruby in Data Engineering
1.1. Why Ruby?
Ruby is a versatile programming language known for its elegant syntax and developer-friendly design principles. While it might not be the first language that comes to mind for data engineering tasks, its unique features make it a strong contender. Ruby’s expressiveness and readability can lead to more maintainable code, making it easier to collaborate on complex data engineering projects.
1.2. Ruby’s Role in Data Engineering
Data engineering involves tasks such as data extraction, transformation, loading, and processing. Ruby’s strengths lie in its ability to manipulate and transform data structures efficiently. With libraries and frameworks tailored to data manipulation, Ruby can handle data engineering tasks with finesse.
2. Handling Large Datasets in Ruby
2.1. Lazy Evaluation
One of Ruby’s advantages in data engineering is its support for lazy evaluation through enumerators. Lazy evaluation allows processing elements one by one, without loading the entire dataset into memory. This feature is particularly beneficial when dealing with vast datasets that might not fit in memory all at once.
ruby # Lazy enumeration example data_stream = large_dataset.lazy.map { |item| transform(item) } data_stream.each { |processed_item| do_something(processed_item) }
2.2. Memory Efficiency
Ruby’s memory management plays a crucial role in handling large datasets. By using techniques like lazy evaluation and efficient data structures, you can minimize memory usage. Additionally, Ruby’s garbage collector helps free up memory occupied by objects that are no longer needed, preventing memory leaks in long-running data processes.
3. Data Processing with Ruby
3.1. Reading and Writing Files
Ruby offers built-in methods for reading and writing files, making it easy to interact with various data formats. Whether you’re dealing with text, CSV, JSON, or other file types, Ruby provides intuitive ways to read and manipulate the data.
ruby # Reading a CSV file require 'csv' CSV.foreach('data.csv', headers: true) do |row| puts row['column_name'] end # Writing to a text file File.open('output.txt', 'w') do |file| file.puts("Hello, world!") end
3.2. Working with CSV and JSON
Ruby’s CSV and JSON libraries simplify working with these common data formats. These libraries offer robust features for parsing and generating data, allowing you to focus on the data transformation logic.
ruby require 'csv' require 'json' # Parsing CSV CSV.foreach('data.csv', headers: true) do |row| # Data transformation logic end # Parsing JSON json_data = File.read('data.json') data = JSON.parse(json_data)
3.3. Parallel Processing
Parallelism can significantly speed up data processing tasks. Ruby’s Parallelism gem provides an easy way to execute code in parallel, distributing the workload across multiple CPU cores.
ruby require 'parallelism' Parallelism.map(large_dataset, in_processes: 4) do |item| transform(item) end
4. Data Transformation Techniques
4.1. Filtering and Mapping
Data transformation often involves filtering out irrelevant data and mapping values to a different format. Ruby’s Enumerable methods like select, map, and reduce provide elegant ways to perform these transformations.
ruby # Filtering using select filtered_data = data.select { |item| item[:value] > 100 } # Mapping using map mapped_data = data.map { |item| item[:value] * 2 }
4.2. Aggregation
Aggregating data to obtain summary statistics is a common data engineering task. Ruby’s Enumerable methods can be combined with the reduce method to perform aggregation operations.
ruby # Aggregating using reduce total_value = data.reduce(0) { |sum, item| sum + item[:value] }
4.3. Joining and Combining
Combining data from multiple sources often requires joining datasets based on common keys. Ruby’s Hash data structure and enumerable methods can simplify these operations.
ruby # Joining data based on a common key result = data1.group_by { |item| item[:key] } .merge(data2.group_by { |item| item[:key] }) { |key, val1, val2| val1 + val2 }
5. Real-world Examples
5.1. Log File Analysis
Ruby can be a valuable tool for analyzing log files to extract insights. You can parse log files, extract relevant information, and generate reports using Ruby’s data manipulation capabilities.
5.2. ETL (Extract, Transform, Load) Processes
Ruby can streamline ETL processes by handling data extraction from various sources, transforming the data, and loading it into the target system.
5.3. Web Scraping and Data Cleaning
Using libraries like Nokogiri, Ruby can scrape data from websites and then clean and transform the extracted data for further analysis.
6. Libraries for Enhanced Data Engineering
6.1. CSV and JSON Libraries
Ruby’s built-in libraries for handling CSV and JSON data formats simplify parsing and generating structured data.
6.2. Parallel Processing with Parallelism
The Parallelism gem enhances Ruby’s parallel processing capabilities, enabling faster execution of data transformation tasks.
6.3. Working with Database Libraries
Ruby offers libraries like ActiveRecord for interacting with databases, making it seamless to integrate data engineering processes with database operations.
7. Best Practices for Efficient Data Engineering in Ruby
7.1. Optimize Memory Usage
Utilize lazy evaluation and efficient data structures to minimize memory consumption, especially when dealing with large datasets.
7.2. Utilize Parallelism Judiciously
While parallel processing can speed up tasks, it’s essential to balance it with the overhead of thread management and potential bottlenecks.
7.3. Profile and Benchmark Your Code
Regularly profile and benchmark your Ruby code to identify performance bottlenecks and areas for optimization.
8. Future Trends and Considerations
8.1. Ruby’s Growing Ecosystem for Data Engineering
The Ruby ecosystem for data engineering is evolving, with new libraries and tools being developed to cater to specific data processing needs.
8.2. Integrating Ruby with Big Data Technologies
As big data technologies continue to expand, integrating Ruby with platforms like Apache Spark or Hadoop might become more relevant for large-scale data processing.
Conclusion
In conclusion, Ruby might not be the most conventional choice for data engineering tasks, but its expressive syntax, elegant design, and powerful libraries make it a viable option. Whether you’re processing log files, performing ETL operations, or scraping data from the web, Ruby’s capabilities can simplify and streamline your data engineering workflow. By harnessing its features, optimizing memory usage, and embracing parallelism, you can effectively process and transform large datasets using Ruby. So, don’t overlook Ruby’s potential in the world of data engineering—give it a try and discover the efficiency and elegance it can bring to your data projects.
Table of Contents
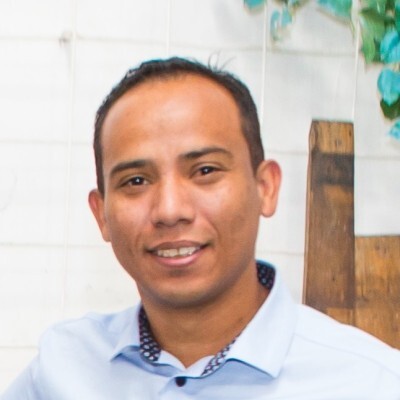
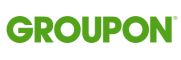