Ruby Q & A
How to declare variables in Ruby?
In Ruby, declaring variables is a fundamental concept that involves naming and assigning values to identifiers. Unlike some other languages, Ruby is dynamically typed, which means you don’t need to specify the data type of a variable explicitly; it is determined at runtime based on the assigned value. Here’s how you declare variables in Ruby:
- Variable Naming: Variable names in Ruby start with a lowercase letter or an underscore (_) followed by letters, digits, or underscores. They should be descriptive and meaningful to make your code more readable. Ruby conventionally uses snake_case for variable names, where words are separated by underscores (e.g., `my_variable`, `user_name`).
- Assignment: You can assign values to variables using the assignment operator `=`. For example, to declare and assign a variable `x` with the value `10`, you would write `x = 10`. The data type of `x` is determined automatically based on the value `10`, which is an integer in this case.
- Reassignment: Ruby allows you to change the value of a variable after it’s declared. For instance, you can update the value of `x` with `x = 20`, and now `x` will hold the value `20`.
- Variable Scope: Ruby has different types of variable scope, including local variables, instance variables, class variables, and global variables. Local variables are confined to the current scope or block and have limited visibility. Instance variables start with `@` and are accessible within an object instance. Class variables start with `@@` and are shared among all instances of a class. Global variables start with `$` and are accessible from anywhere in the program.
Here’s an example:
```ruby
# Declaring and assigning a local variable
age = 30
# Reassigning the variable
age = 35
# Declaring and using an instance variable within a class
class Person
def initialize(name)
@name = name
end
end
# Creating an object and accessing the instance variable
person = Person.new("Alice")
puts person.instance_variable_get(:@name) # Outputs "Alice"
```
```ruby
# Declaring and assigning a local variable
age = 30
# Reassigning the variable
age = 35
# Declaring and using an instance variable within a class
class Person
def initialize(name)
@name = name
end
end
# Creating an object and accessing the instance variable
person = Person.new("Alice")
puts person.instance_variable_get(:@name) # Outputs "Alice"
```
```ruby # Declaring and assigning a local variable age = 30 # Reassigning the variable age = 35 # Declaring and using an instance variable within a class class Person def initialize(name) @name = name end end # Creating an object and accessing the instance variable person = Person.new("Alice") puts person.instance_variable_get(:@name) # Outputs "Alice" ```
Declaring variables in Ruby is straightforward, and you can use descriptive names to make your code more readable. Ruby’s dynamic typing allows for flexibility in variable assignment, and variables’ scope determines their visibility and accessibility within the code.
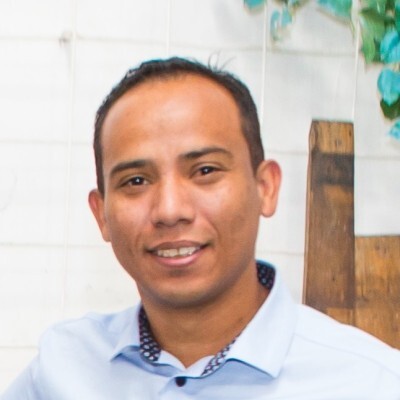
Previously at
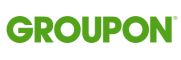
Experienced software professional with a strong focus on Ruby. Over 10 years in software development, including B2B SaaS platforms and geolocation-based apps.