Demystifying Ruby’s Enumerable Module: Harnessing Its Power
In the world of Ruby programming, the Enumerable module is undoubtedly one of the most powerful and flexible tools available. It’s chock-full of methods that make data manipulation not just possible, but simple, clean, and efficient. Such complexity often highlights the importance of opting to hire Ruby developers to navigate the nuances effectively.
The beauty of Ruby’s Enumerable module lies in its capacity to handle a plethora of data types and structures: arrays, hashes, sets, and more. However, with this flexibility and versatility comes a certain level of complexity. This is where the skills and expertise of hired Ruby developers come into play, as they can proficiently unravel such complexity.
In this blog post, we aim to demystify Ruby’s Enumerable module and explore how to harness its full potential. Whether you’re a seasoned Ruby programmer or an entrepreneur looking to hire Ruby developers, understanding the power and application of the Enumerable module is crucial to fully utilize Ruby’s robustness.
What is Ruby’s Enumerable Module?
The Enumerable module is a collection of methods that get mixed into Ruby’s collection classes like Array, Hash, and Range. It leverages the power of Ruby’s mixins, which allow classes to utilize methods defined elsewhere. This promotes code reuse and helps keep the Ruby code DRY (Don’t Repeat Yourself).
The Enumerable methods mainly focus on iterating over collections, hence the name Enumerable. They offer functionalities for traversing, searching, sorting, manipulating, and summarizing data.
Key Methods in the Enumerable Module
Let’s take a closer look at some of the key methods provided by the Enumerable module, and how they can supercharge your Ruby code.
- `each` and `map`
`each` and `map` are perhaps the most frequently used methods in Enumerable. They are both used to traverse collections, but there’s a fundamental difference between the two.
`each` iterates over each element of a collection, executing the block of code supplied to it. However, it doesn’t modify the original collection or create a new one. It simply returns the original collection after executing the block.
```ruby [1, 2, 3].each { |n| puts n * 2 } # Output: 2 # Output: 4 # Output: 6
On the other hand, `map` creates a new array containing the results of the block’s execution for each element in the original collection.
```ruby new_array = [1, 2, 3].map { |n| n * 2 } # new_array is now: [2, 4, 6]
- `select` and `reject`
These are filter methods that help you select or reject elements from a collection based on the condition specified in the block.
`select` returns a new collection containing all elements for which the block’s condition is true.
```ruby new_array = [1, 2, 3, 4, 5].select { |n| n.even? } # new_array is now: [2, 4]
`reject` does the opposite – it returns a new collection with all elements for which the block’s condition is false.
```ruby new_array = [1, 2, 3, 4, 5].reject { |n| n.even? } # new_array is now: [1, 3, 5]
- `reduce` (also known as `inject`)
`reduce` is a powerful method that can be used to process a collection of data and combine it into a single value. It takes an initial value and a binary operation (specified in the block) and applies this operation cumulatively to the elements of the collection.
```ruby sum = [1, 2, 3, 4, 5].reduce(0) { |acc, n| acc + n } # sum is now: 15
- `sort` and `sort_by`
`sort` and `sort_by` are used to arrange the elements of a collection in a certain order. `sort` uses the spaceship operator (`<=>`) to compare elements, while `sort_by` allows you to specify the attribute for sorting.
```ruby sorted_array = [5, 3, 2, 1, 4].sort # sorted_array is now: [1, 2, 3, 4, 5] words = %w[apple zebra banana mango] sorted_words = words.sort_by { |word| word.length } # sorted_words is now: ["apple", "mango", "zebra", "banana"]
Harnessing the Power of Enumerable
When working with collections of data, the Enumerable module is your best friend. It provides methods that can simplify complex operations, reduce the amount of code you need to write, and make your code cleaner and more efficient.
The real power of Enumerable becomes evident when you combine its methods. For instance, you can use `select` and `map` together to filter a collection and then transform it:
```ruby numbers = [1, 2, 3, 4, 5, 6] even_squares = numbers.select { |n| n.even? }.map { |n| n * n } # even_squares is now: [4, 16, 36]
Here, `select` filters out the odd numbers, and then `map` squares each of the remaining even numbers.
Conclusion
The Enumerable module is a cornerstone of Ruby programming. It’s powerful, versatile, and intuitive. Mastering its use is a significant step towards becoming a proficient Ruby programmer, and a core reason why many businesses decide to hire Ruby developers.
Whether you’re processing arrays of data, manipulating hashes, or even working with your custom classes, the Enumerable module has a tool for every job. Remember, practice is key when it comes to harnessing the power of Enumerable. This is especially important for those looking to hire Ruby developers, as their efficiency will be greatly enhanced by a deep understanding of this module.
Keep coding, keep iterating, and keep improving. With the Enumerable module, Ruby truly becomes a joy to work with, allowing you to express complex ideas with simple, elegant code. This is precisely why so many companies choose to hire Ruby developers – they appreciate the ability to convert complex processes into clear, elegant solutions.
So go ahead and explore, experiment, and make the most of this powerful module at your disposal. Whether you’re a developer seeking to level up your skills or a business leader planning to hire Ruby developers, a solid grasp of the Enumerable module will prove invaluable. Happy coding!
Table of Contents
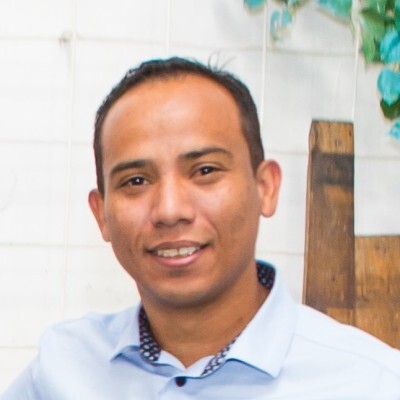
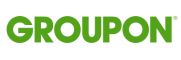