Exploring Ruby’s Closures: Blocks, Procs, and Lambdas
In the world of programming languages, closures are a powerful concept that can greatly enhance the flexibility and expressiveness of code. Ruby, a dynamic and object-oriented language, offers various mechanisms to work with closures, including blocks, Procs, and Lambdas. These constructs allow developers to encapsulate code and pass it around as objects, enabling concise and reusable code patterns.
In this blog, we will take a deep dive into Ruby’s closures and explore how blocks, Procs, and Lambdas work, their similarities, and their differences. We will also examine practical use cases and provide code samples to demonstrate their capabilities. So, let’s get started!
Understanding Blocks
Blocks are Ruby’s simplest form of closures. They are chunks of code that can be passed to methods for execution. Blocks are enclosed within curly braces ({}) or between the keywords do and end. They are often used in conjunction with iterators and method invocations to customize behavior or perform specific actions within a given context.
Here’s an example of a block being used with the each method to iterate over an array:
ruby numbers = [1, 2, 3, 4, 5] numbers.each do |number| puts number * 2 end
In this code snippet, the block do |number| … end is passed to the each method, which invokes the block for each element of the numbers array. The block multiplies each element by 2 and prints the result. Blocks are a great way to encapsulate behavior and make code more modular and reusable.
Understanding Procs
Procs, short for procedures, are objects that encapsulate blocks of code. Unlike blocks, Procs can be assigned to variables, stored in data structures, and passed around as arguments to methods. This makes Procs highly flexible and allows for the creation of reusable code snippets.
To create a Proc, we use the Proc.new or proc methods and pass in the block of code we want to encapsulate. Here’s an example:
ruby hello_proc = Proc.new do puts "Hello, World!" end hello_proc.call
In this example, we create a Proc named hello_proc that encapsulates the block do … end. We can invoke the block of code by calling the call method on the Proc object. This allows us to treat the block as an object and execute it wherever and whenever we need it.
One of the advantages of Procs is their ability to accept arguments. Let’s modify the previous example to include an argument:
ruby greeting_proc = Proc.new do |name| puts "Hello, #{name}!" end greeting_proc.call("Alice") greeting_proc.call("Bob")
In this updated example, the Proc accepts an argument name and interpolates it within the greeting message. We can then call the Proc multiple times with different arguments, resulting in personalized greetings.
Understanding Lambdas
Lambdas are similar to Procs in that they also encapsulate blocks of code. However, there are subtle differences between the two. Lambdas enforce strict argument arity, meaning they expect a specific number of arguments when called. Procs, on the other hand, are more lenient and can handle a variable number of arguments.
To create a Lambda in Ruby, we use the -> or lambda keyword followed by the block of code. Here’s an example:
ruby hello_lambda = -> do puts "Hello, World!" end hello_lambda.call
In this example, we create a Lambda named hello_lambda that encapsulates the block do … end. We invoke the Lambda using the call method, just like with Procs.
Now, let’s modify the previous example to include an argument:
ruby greeting_lambda = ->(name) do puts "Hello, #{name}!" end greeting_lambda.call("Alice") greeting_lambda.call("Bob")
Similar to Procs, Lambdas can accept arguments. In this case, we define the argument name within parentheses after the -> symbol. We can then call the Lambda and provide the necessary arguments.
The difference between Lambdas and Procs becomes more apparent when it comes to argument handling. Lambdas strictly enforce the number of arguments passed, whereas Procs do not. Let’s illustrate this with an example:
ruby def demo_proc proc = Proc.new { |x, y| puts "#{x}, #{y}" } proc.call(1) end def demo_lambda lambda = ->(x, y) { puts "#{x}, #{y}" } lambda.call(1) end demo_proc # Outputs: "1, " demo_lambda # Raises an ArgumentError
In this example, the demo_proc method demonstrates that Procs can handle missing arguments gracefully. When we call the Proc with only one argument, the missing argument y is assigned nil. On the other hand, the demo_lambda method, using a Lambda, raises an ArgumentError since the expected number of arguments is not met.
Conclusion
Ruby’s closures, including blocks, Procs, and Lambdas, provide a powerful set of tools for flexible and expressive programming. Blocks allow us to encapsulate behavior within methods, Procs enable the creation of reusable code snippets, and Lambdas enforce strict argument arity. By understanding and utilizing these closure mechanisms, Ruby developers can write more modular, maintainable, and elegant code.
In this blog, we explored the fundamentals of blocks, Procs, and Lambdas, highlighting their similarities and differences. We provided code samples to demonstrate their usage and showcased practical scenarios where each closure type shines. By harnessing the power of closures, Ruby developers can unlock a world of possibilities and take their programming skills to the next level.
So go ahead, embrace the power of closures in Ruby, and elevate your code to new heights! Happy coding!
Table of Contents
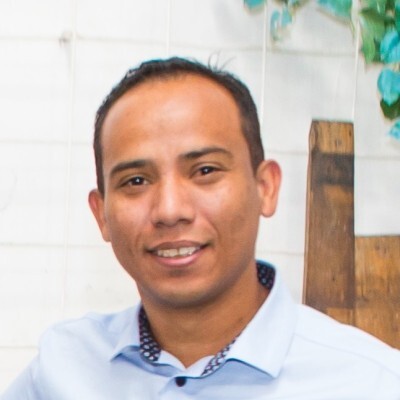
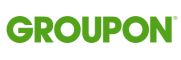