Integrating External APIs with Ruby: Simplifying Data Retrieval
In today’s interconnected world, integrating external APIs (Application Programming Interfaces) has become a fundamental aspect of modern software development. APIs enable developers to access a wide range of data and services provided by external platforms, empowering them to enrich their applications with robust features and functionality. In this comprehensive guide, we will explore how to integrate external APIs with Ruby, a powerful and versatile programming language known for its simplicity and readability.
Ruby provides an array of tools and libraries that make working with APIs a breeze. Whether you need to retrieve weather data, consume social media feeds, or access financial information, Ruby offers numerous options to simplify data retrieval and streamline the integration process. In this blog, we will walk through the steps of integrating external APIs using Ruby, along with code samples to illustrate key concepts and best practices.
Understanding APIs: A Brief Overview
Before we delve into integrating APIs with Ruby, it’s essential to understand what APIs are and how they function. An API is a set of rules and protocols that allow different software applications to communicate with each other. APIs provide a standardized way to exchange data and perform specific operations.
There are various types of APIs, such as web APIs, which are commonly used for integrating external services into web applications. Web APIs use HTTP (Hypertext Transfer Protocol) to transmit requests and responses. Most web APIs return data in widely-used formats like JSON (JavaScript Object Notation) or XML (eXtensible Markup Language).
Choosing the Right API
Selecting the right API for your project is crucial to ensure efficient data retrieval and seamless integration. Consider the following factors when choosing an API:
- Functionality: Determine the specific features and data you require from the API.
- Documentation: Look for well-documented APIs with clear instructions and examples.
- Authentication: Check if the API requires authentication and supports the required authentication methods (e.g., API keys, OAuth).
- Rate Limits: Understand the rate limits imposed by the API to ensure your application remains within the allowed usage.
- Support: Consider the availability of support channels, such as community forums or official support teams.
Once you have chosen the API that meets your requirements, you can start integrating it into your Ruby application.
Setting Up Your Ruby Development Environment
Before integrating external APIs, ensure you have Ruby and a package manager like Bundler installed on your system. Create a new Ruby project or navigate to an existing project directory.
To manage dependencies, create a Gemfile in the project directory and add the required gems. For example, to use the ‘httparty’ gem, add the following line to your Gemfile:
ruby gem 'httparty'
Making API Requests with Net::HTTP
Ruby’s standard library includes the Net::HTTP module, which provides classes and methods for making HTTP requests. To send a GET request to an API endpoint, follow these steps:
1. Require the ‘net/http’ library:
ruby require 'net/http'
2. Create a URI object representing the API endpoint:
ruby uri = URI('https://api.example.com/data')
3. Use the Net::HTTP module to make the request:
ruby response = Net::HTTP.get_response(uri)
4. Parse the response:
ruby body = response.body
5. Process the retrieved data as needed.
While Net::HTTP is a powerful tool for making API requests, it can be verbose and require additional code for tasks like error handling and authentication. To simplify API calls, we can use the HTTParty gem.
Simplifying API Calls with HTTParty
HTTParty is a popular Ruby gem that provides a clean and concise syntax for making HTTP requests and simplifies handling JSON and XML responses. To use HTTParty, add it to your Gemfile and run bundle install as mentioned earlier.
Here’s an example of using HTTParty to retrieve data from an API:
ruby require 'httparty' response = HTTParty.get('https://api.example.com/data') body = response.body # Process the retrieved data as needed.
HTTParty’s get method sends a GET request to the specified URL and returns a response object. We can access the response body using the body method. HTTParty automatically handles parsing JSON or XML responses into a Ruby hash or object, simplifying data extraction and manipulation.
Parsing JSON Responses with Ruby
When working with APIs, JSON is a widely used format for data exchange. Ruby provides built-in support for parsing and generating JSON data through the ‘json’ library.
To parse a JSON response using the ‘json’ library, follow these steps:
1. Require the ‘json’ library:
ruby require 'json'
2. Parse the JSON response:
ruby response_body = '{"name": "John", "age": 30}' parsed_response = JSON.parse(response_body)
The JSON.parse method converts the JSON response into a Ruby hash or object, allowing easy access to its properties.
Authenticating API Requests
Many APIs require authentication to ensure secure access and prevent unauthorized usage. Depending on the API, you may need to include an API key, a token, or use OAuth for authentication. Let’s explore a common approach using an API key.
- Obtain an API key from the provider.
- Include the API key in your request header or query parameters:
ruby response = HTTParty.get('https://api.example.com/data', headers: { 'API-Key' => 'YOUR_API_KEY' })
Always ensure you keep your API keys and authentication credentials secure. Consider using environment variables or configuration files to store sensitive information separately from your code.
Error Handling and Exception Management
When integrating APIs, it’s crucial to handle errors gracefully and provide meaningful feedback to the users or log errors for debugging purposes. Ruby provides a robust exception handling mechanism that allows you to catch and handle exceptions.
Here’s an example of error handling when using HTTParty:
ruby begin response = HTTParty.get('https://api.example.com/data') # Process the retrieved data as needed. rescue StandardError => e puts "An error occurred: #{e.message}" end
The begin and rescue keywords enable you to wrap the code that may raise exceptions, allowing you to handle any potential errors.
Caching API Responses for Improved Performance
Caching API responses can significantly enhance the performance and reduce the load on both your application and the API provider. By storing the retrieved data locally and serving it from the cache instead of making repetitive API requests, you can reduce response times and minimize the risk of hitting rate limits.
Ruby provides various caching mechanisms, such as the ‘redis’ or ‘memcached’ gems, which allow you to store data in memory or on disk.
To cache API responses using the ‘redis’ gem, follow these steps:
- Install the ‘redis’ gem by adding it to your Gemfile and running bundle install.
2. Set up a Redis client:
ruby require 'redis' redis = Redis.new
3. Make an API request and cache the response:
ruby response = HTTParty.get('https://api.example.com/data') redis.set('api_response', response.body)
4. Retrieve the cached response:
ruby cached_response = redis.get('api_response')
By incorporating caching into your API integration, you can achieve faster response times and better overall performance.
Testing API Integrations
Testing is an essential part of any software development process. When integrating external APIs, it’s crucial to thoroughly test your code to ensure its reliability and accuracy. Ruby provides a rich ecosystem of testing frameworks and libraries that can assist you in writing comprehensive API tests.
One popular testing framework in the Ruby community is RSpec. RSpec allows you to write expressive and readable tests using a behavior-driven development (BDD) approach. By using RSpec, you can define API integration tests that validate the expected behavior of your code.
Here’s an example of an RSpec test for an API integration:
ruby require 'rspec' require 'httparty' RSpec.describe 'API Integration' do it 'retrieves data successfully' do response = HTTParty.get('https://api.example.com/data') expect(response.code).to eq(200) expect(response.body).not_to be_empty end end
This example tests whether the API integration successfully retrieves data by checking the HTTP response code and ensuring the response body is not empty. RSpec provides numerous matchers and assertions to verify various aspects of API interactions.
Popular Ruby Gems for API Integration
Ruby’s vast ecosystem offers numerous gems that simplify API integration and provide additional functionality. Here are a few popular gems to consider when working with APIs in Ruby:
- HTTParty: A gem that simplifies making HTTP requests and handling responses.
- RestClient: Another gem for sending HTTP requests and managing responses.
- Faraday: A flexible HTTP client that supports multiple adapters and middlewares.
- OAuth: A gem that simplifies OAuth authentication for API integrations.
- Twitter: A gem specifically designed for interacting with the Twitter API.
Explore these gems based on your specific needs and leverage their capabilities to streamline your API integration workflow.
Building a Practical Example: Integrating the OpenWeatherMap API
To demonstrate the integration of an external API in Ruby, let’s build a practical example using the OpenWeatherMap API. The OpenWeatherMap API provides access to weather forecasts, current weather data, and historical weather information.
Step 1: Obtain an API key from the OpenWeatherMap website.
Step 2: Set up your Ruby project as outlined in previous sections.
Step 3: Use HTTParty to retrieve weather data:
ruby require 'httparty' response = HTTParty.get("http://api.openweathermap.org/data/2.5/weather?q=London&appid=YOUR_API_KEY") body = response.body # Process and display the weather data.
This example fetches the current weather data for London using the OpenWeatherMap API. Replace YOUR_API_KEY with the actual API key obtained in Step 1.
Best Practices and Tips for API Integration
To ensure efficient and reliable API integration, consider the following best practices and tips:
- Thoroughly read the API documentation to understand the available endpoints, parameters, and data formats.
- Implement proper error handling and exception management to gracefully handle potential errors.
- Utilize caching to reduce API requests and enhance overall performance.
- Securely store and manage authentication credentials using environment variables or configuration files.
- Implement appropriate logging to capture API interactions and assist in debugging.
- Write comprehensive tests to validate the behavior and correctness of your API integrations.
- Monitor API usage and respect rate limits to ensure compliance with the API provider’s terms of service.
- Keep your API integration code modular and maintainable by adhering to best practices such as separation of concerns and code organization principles.
Conclusion
Integrating external APIs with Ruby opens up a world of possibilities for your applications. Whether you’re retrieving weather data, interacting with social media platforms, or accessing financial information, Ruby provides a robust set of tools and libraries to simplify data retrieval and streamline integration. By following the guidelines and best practices outlined in this comprehensive guide, you can confidently integrate APIs, enhance your applications, and deliver valuable experiences to your users. Happy coding!
Table of Contents
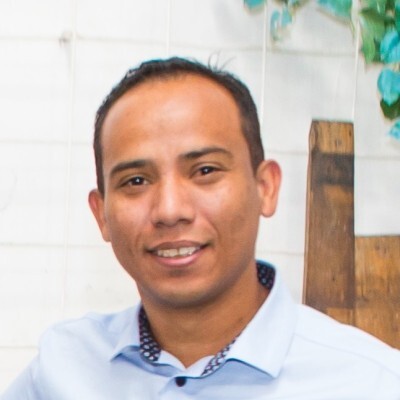
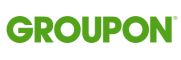