Exploring Ruby’s Functional Programming Features
In the world of programming languages, Ruby has always been celebrated for its elegant syntax and object-oriented nature. However, beneath its object-oriented foundation lies a powerful functional core. Functional programming is a programming paradigm that emphasizes the use of pure functions and immutable data. While Ruby is predominantly an object-oriented language, it offers a plethora of features that enable developers to embrace functional programming principles. In this blog post, we will delve into Ruby’s functional programming capabilities, exploring the benefits of incorporating functional techniques into your Ruby codebase. We will also provide you with practical code samples to demonstrate how to harness the power of functional programming in Ruby.
Understanding Functional Programming
Before we dive into the functional programming features in Ruby, let’s take a moment to understand the key principles of functional programming. Functional programming revolves around the idea of writing programs by composing pure functions, which do not have any side effects and always produce the same output for a given input. This purity enables developers to reason about code more easily and enhances code readability and maintainability.
Ruby’s Functional Programming Features
1. First-Class Functions and Closures
Ruby treats functions as first-class citizens, allowing you to pass functions as arguments, return functions from other functions, and assign functions to variables. This enables you to write higher-order functions, which are functions that can take other functions as arguments or return functions as results. Closures, on the other hand, allow functions to “remember” the variables in their surrounding scope, even after the outer function has finished executing. Let’s take a look at an example:
ruby def multiply_by(factor) lambda { |n| n * factor } end double = multiply_by(2) triple = multiply_by(3) puts double.call(5) # Output: 10 puts triple.call(5) # Output: 15
In this example, the multiply_by function returns a lambda function that multiplies its argument by the factor variable defined in its surrounding scope. The returned functions, double and triple, can then be invoked with arguments to perform the respective multiplications.
2. Immutability and Immutable Data Structures:
In functional programming, immutability plays a crucial role. Immutable objects cannot be modified after they are created, which eliminates the possibility of unexpected changes and makes the code more predictable. While Ruby objects are mutable by default, Ruby provides immutable data structures such as frozen strings and frozen arrays. By leveraging these data structures, you can ensure that certain parts of your code remain immutable, leading to more reliable and bug-resistant code. Let’s see an example:
ruby name = "John".freeze numbers = [1, 2, 3].freeze name.upcase! # Raises a RuntimeError since frozen strings are immutable numbers << 4 # Raises a RuntimeError since frozen arrays are immutable
In this example, we freeze the name string and the numbers array, preventing any modifications to them after they have been defined. Attempting to modify them afterward results in a RuntimeError.
3. Higher-Order Functions and Function Composition
Higher-order functions are a fundamental aspect of functional programming. Ruby allows you to create higher-order functions by passing functions as arguments or returning them from other functions. Additionally, you can compose functions together to create more complex operations by leveraging function composition. Consider the following example:
ruby def add_two(n) n + 2 end def square(n) n * n end def compose(f, g) lambda { |x| f.call(g.call(x)) } end add_two_and_square = compose(method(:square), method(:add_two)) puts add_two_and_square.call(4) # Output: 36
In this example, we define the add_two and square functions. The compose function takes two functions, f and g, and returns a new function that applies f to the result of applying g to its argument. We then use compose to create a new function called add_two_and_square, which adds two to its argument and then squares the result. When we invoke add_two_and_square with 4, we get the expected output of 36.
4. Lazy Evaluation and Infinite Sequences:
Lazy evaluation is a technique where values are computed only when needed. This can be particularly useful when dealing with large datasets or infinite sequences. Ruby provides lazy enumeration through the lazy method, which allows you to create lazy sequences. Lazy sequences are only evaluated as elements are accessed, which can significantly improve performance. Let’s take a look at an example:
ruby fibonacci = Enumerator.new do |yielder| a = 0 b = 1 loop do yielder << a a, b = b, a + b end end.lazy puts fibonacci.take(10).to_a # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
In this example, we create an Enumerator that generates Fibonacci numbers. By calling the lazy method on the enumerator, we make it lazy. When we invoke take(10) on the lazy enumerator, only the first ten Fibonacci numbers are computed, allowing us to work with infinite sequences in a memory-efficient manner.
Conclusion
In this blog post, we’ve explored the functional programming features that Ruby has to offer. We’ve seen how Ruby allows us to leverage first-class functions, closures, immutability, higher-order functions, function composition, lazy evaluation, and infinite sequences. By incorporating these functional programming techniques into our Ruby codebase, we can write cleaner, more modular, and easier-to-reason code.
Functional programming principles can enhance your Ruby programming skills and help you tackle complex problems with ease. Whether you are already familiar with functional programming or just starting to explore this paradigm, embracing functional techniques in Ruby will undoubtedly broaden your horizons as a developer. So go ahead, experiment with functional programming in Ruby, and unlock the true potential of your code. Happy coding!
Table of Contents
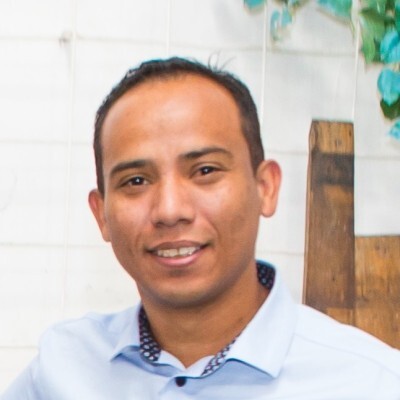
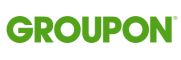