Exploring Ruby’s GUI Frameworks: Building Desktop Applications
In the realm of programming languages, Ruby has earned its reputation for simplicity and elegance. While it’s commonly associated with web development, thanks to frameworks like Ruby on Rails, it’s important not to overlook its capabilities in other domains. One such domain is desktop application development, and Ruby offers a variety of GUI (Graphical User Interface) frameworks that empower developers to craft desktop applications with ease and efficiency. In this article, we will embark on a journey to explore Ruby’s GUI frameworks, understand their unique features, and even peek into some code samples to demonstrate their power.
Table of Contents
1. Why Consider Ruby for Desktop Applications?
Before we delve into the specifics of GUI frameworks, let’s briefly discuss why Ruby can be a great choice for building desktop applications. Ruby’s syntax is known for its readability and simplicity, making it a language that’s easy to learn and work with. This characteristic extends to desktop application development, allowing developers to focus on crafting functional and aesthetically pleasing user interfaces without getting bogged down by complex code.
Furthermore, the Ruby community is known for its helpfulness and enthusiasm. This means that if you encounter challenges while developing a desktop application, you’re likely to find a supportive community ready to assist you. This kind of collaborative environment can significantly accelerate your learning and development process.
2. Introducing Ruby’s GUI Frameworks
Ruby offers several GUI frameworks, each with its own set of features and benefits. Let’s explore some of the most popular ones:
2.1. Shoes
Shoes is a lightweight GUI toolkit for Ruby that prioritizes simplicity and ease of use. It provides a simple syntax that allows developers to create windows, buttons, text inputs, and other common GUI components. Shoes’ focus on minimalism makes it a great choice for small utility applications or prototypes.
Here’s a quick example of creating a simple Shoes application:
ruby require 'shoes' Shoes.app do button "Click me!" do alert "Hello, Shoes!" end end
2.2. Tk
Tk is a graphical user interface toolkit that’s not specific to Ruby; it’s also available for other programming languages like Tcl and Python. Tk provides a wide range of GUI components and is known for its simplicity and cross-platform compatibility.
Creating a basic Tk application in Ruby is quite straightforward:
ruby require 'tk' root = TkRoot.new { title "Tkinter Example" } TkLabel.new(root) do text "Hello, Tk!" pack { padx 15 ; pady 15; side 'left' } end Tk.mainloop
2.3. GTK
GTK (GIMP Toolkit) is a popular open-source GUI framework that provides a rich set of widgets for building desktop applications. It’s widely used in Linux environments and has bindings for various programming languages, including Ruby.
Creating a GTK application involves more steps, but the flexibility it offers is rewarding:
ruby require 'gtk3' app = Gtk::Application.new('org.example.HelloWorld', :flags_none) app.signal_connect('activate') do |application| window = Gtk::ApplicationWindow.new(application) window.set_title('Hello, GTK!') window.set_default_size(200, 100) label = Gtk::Label.new(label: 'Hello, GTK!') window.add(label) window.show_all end app.run
3. Choosing the Right Framework for Your Project
Each framework has its own strengths and target use cases. When choosing a framework for your desktop application, consider the following factors:
- Complexity: If your project requires a simple interface and quick development, a lightweight framework like Shoes might be ideal. On the other hand, for more complex applications with intricate user interfaces, GTK or Tk might be better suited.
- Platform Compatibility: If your application needs to run on multiple platforms (Windows, macOS, Linux), consider frameworks that offer cross-platform support, such as Tk and GTK.
- Community and Documentation: A strong community and comprehensive documentation can be invaluable resources, especially when you’re just starting out with a framework. Shoes and Tk have relatively simpler learning curves, while GTK might require a bit more investment in learning.
- Customization: If you need extensive customization and a wide range of UI components, GTK provides a plethora of options. Shoes, while simple, might be limiting in terms of available components.
4. Building Your First Ruby Desktop Application
Let’s dive into building a simple desktop application using the Shoes framework. Our goal is to create a basic to-do list application with an intuitive user interface.
ruby require 'shoes' Shoes.app(title: "To-Do List", width: 300, height: 400) do stack(margin: 10) do para "Add a new task:" flow do @task_input = edit_line(width: 200) button "Add" do if @task_input.text != "" @tasks.prepend do para @task_input.text @task_input.text = "" end end end end end @tasks = stack(margin: 10) end
This simple application lets users input tasks and add them to the to-do list. It demonstrates the power of Shoes in creating quick and intuitive user interfaces.
Conclusion
Ruby’s GUI frameworks offer a spectrum of choices for building desktop applications. Whether you’re aiming for simplicity, cross-platform compatibility, or extensive customization, there’s a framework that fits your needs. Shoes, Tk, and GTK each have their strengths and unique features that can empower you to create visually appealing and functional desktop applications. As you explore these frameworks and delve deeper into Ruby desktop development, you’ll discover the joy of crafting applications that seamlessly integrate into users’ desktop experiences. Happy coding!
In this article, we’ve barely scratched the surface of what’s possible with Ruby’s GUI frameworks. The best way to master these tools is to roll up your sleeves, dive into the documentation and tutorials, and start experimenting with your own desktop application projects. So go ahead, embark on your journey to becoming a proficient Ruby desktop application developer!
Table of Contents
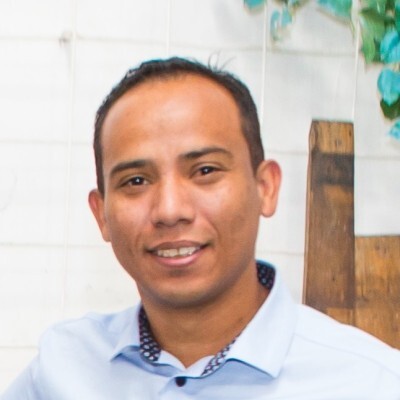
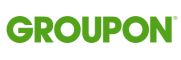