Mastering Ruby: A Comprehensive Guide to the Language’s Key Functions
In the ever-evolving field of programming, Ruby holds a special place. With a clear syntax, flexibility, and the philosophy of “Matz is nice, and so we are nice,” named after its creator Yukihiro “Matz” Matsumoto, Ruby offers an enjoyable and practical programming experience. This has resulted in a steady demand to hire Ruby developers who can leverage the power of this dynamic language. This article will delve into the key features of the Ruby programming language, allowing both beginners and intermediate developers to further their understanding and mastery, thus preparing them for opportunities where businesses are looking to hire Ruby developers.
A Quick Overview of Ruby
Ruby is a high-level, dynamic, and interpreted programming language. It’s designed for simplicity and productivity with an elegant syntax that’s natural to read and easy to write. Being an object-oriented language, Ruby views everything as an object, with properties (attributes) and actions (methods). This object-oriented structure and its elegant simplicity are part of why many companies choose to hire Ruby developers. It’s also a flexible language, allowing its users to freely alter its parts. Structurally, Ruby features exception handling, garbage collection, and dynamic typing, making it a robust choice for businesses looking to hire Ruby developers for a diverse range of projects.
Ruby Syntax and Basic Data Types
One of Ruby’s standout features is its simplicity. Here’s how you might define a “Hello, World!” program:
```ruby puts "Hello, World!"
As seen above, no semicolons or main methods are needed to print text on the screen.
Ruby has several basic data types: Numbers, Strings, Symbols, Hashes, and Arrays.
```ruby # Numbers num = 42 # Strings str = "Hello, Ruby!" # Symbols (immutable strings) sym = :symbol # Arrays arr = [1, 2, 3, 4, 5] # Hashes (similar to dictionaries in other languages) hash = { "name" => "Ruby", "version" => "3.0.1" }
Understanding Ruby’s Key Functions
1. Control Structures
Control structures are at the core of every programming language, and Ruby is no different. These structures control the flow of your code, using conditional and looping constructs like if, else, while, and for.
```ruby # If-else age = 21 if age >= 18 puts "You are an adult." else puts "You are a minor." end # While loop i = 0 while i < 5 puts i i += 1 end # For loop for i in 0..5 puts i end
2. Object-Oriented Programming (OOP)
Ruby is a fully object-oriented language. This means that every value is an object, including classes and types that many other languages designate as primitives (like integers, booleans, and “null”). Here’s how you might define a class and create an object in Ruby:
```ruby # Define a class class Dog def bark puts "Woof!" end end # Create an object fido = Dog.new fido.bark # Outputs: Woof!
3. Blocks, Procs, and Lambdas
Ruby includes a few features that aren’t found in many languages — blocks, procs, and lambdas. These powerful tools make Ruby exceptionally good at handling tasks that might be cumbersome in other languages.
Blocks are anonymous pieces of code that can accept input and are used throughout Ruby’s standard library, particularly for iteration:
```ruby # Blocks [1, 2, 3].each do |num| puts num end
Procs (short for procedures) are saved blocks, which can be reused multiple times. Lambdas are similar to procs but behave more like methods, particularly with how they handle arguments and return statements:
```ruby # Procs doubler = Proc.new { |num| puts num * 2 } [1, 2, 3].each(&doubler) # Lambdas greeter = ->(name) { puts "Hello, #{name}!" } greeter.call("Ruby")
4. Modules and Mixins
Modules in Ruby are collections of methods and constants. They cannot generate instances. They’re used to bundle reusable code in one place, and they can be mixed into classes using the `include` keyword:
```ruby module Walkable def walk puts "I'm walking." end end class Person include Walkable end p = Person.new p.walk # Outputs: I'm walking.
5. Metaprogramming
Ruby’s metaprogramming is part of what makes the language so flexible. Metaprogramming is the practice of writing code that writes code. This allows for dynamic method creation:
```ruby class Book def initialize(title) @title = title end def self.create_accessors_for(*attributes) attributes.each do |attribute| define_method(attribute) do instance_variable_get("@#{attribute}") end define_method("#{attribute}=") do |value| instance_variable_set("@#{attribute}", value) end end end create_accessors_for :title end b = Book.new("Mastering Ruby") b.title # Outputs: "Mastering Ruby"
In this example, `create_accessors_for` is a class method that dynamically creates getter and setter methods for the specified attribute.
Conclusion
Ruby is a powerful, flexible, and enjoyable programming language. Its straightforward syntax, object-oriented nature, and metaprogramming capabilities make it a go-to choice for both newcomers and seasoned developers. This combination of power and flexibility is why many organizations opt to hire Ruby developers for a range of projects. Whether you’re building a quick script, a complex web application with Ruby on Rails, or anything in between, mastering Ruby’s key functions will provide a strong foundation for effective and efficient programming. It also adds significant weight to your profile should you aspire to be among those who are being sought to hire as Ruby developers.
Remember, the best way to master Ruby, like any other language, is through regular practice. Happy coding!
Table of Contents
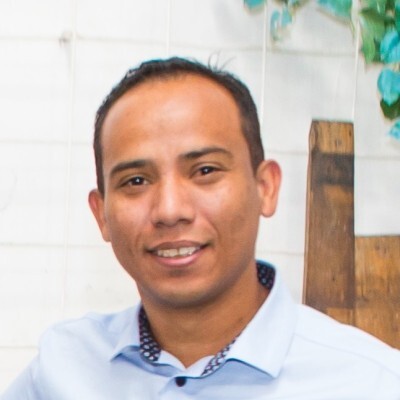
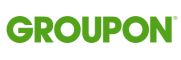