How to iterate through an array in Ruby?
Iterating through an array in Ruby is a fundamental operation that allows you to access and process each element of the array one by one. Ruby provides several methods and techniques for iteration, making it easy to work with arrays efficiently. Here are some common methods for iterating through an array in Ruby:
- Using the `each` Method: The `each` method is the most straightforward way to iterate through an array. It allows you to execute a block of code for each element in the array. Here’s an example:
```ruby my_array = [1, 2, 3, 4] my_array.each do |element| puts element end ```
In this example, the `each` method iterates through `my_array`, and the block of code inside the `do…end` is executed for each element, printing them one by one.
- Using the `map` Method: The `map` method is used when you want to transform each element of the array and create a new array with the transformed values. It returns a new array containing the results of applying the block of code to each element.
```ruby my_array = [1, 2, 3, 4] squared_array = my_array.map { |element| element * element } ```
In this example, `squared_array` will contain `[1, 4, 9, 16]`, which are the squares of the original elements.
- Using Other Iteration Methods: Ruby provides a variety of other iteration methods like `each_with_index`, `select`, `reject`, and `reduce` (or `inject`) to perform specific tasks during iteration. `each_with_index` allows you to access both the element and its index, while `select` and `reject` filter elements based on a condition. `reduce` (or `inject`) is used for accumulating values.
```ruby my_array = [1, 2, 3, 4] my_array.each_with_index do |element, index| puts "Element #{element} at index #{index}" end even_numbers = my_array.select { |element| element.even? } sum = my_array.reduce(0) { |acc, element| acc + element } ```
These methods cover a wide range of scenarios for iterating through arrays in Ruby. Depending on your specific task, you can choose the most appropriate iteration method to process the elements effectively and elegantly.
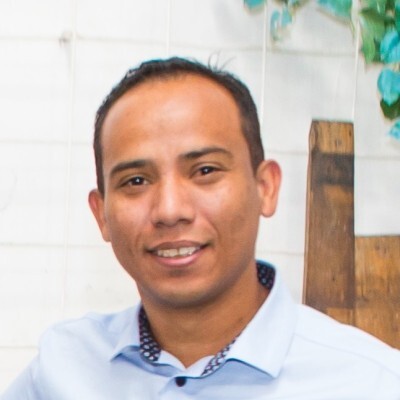
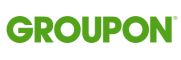