Working with Data in Ruby: Arrays, Hashes, and Beyond
Ruby, an object-oriented scripting language, provides programmers and Ruby developers with a multitude of ways to store, manipulate, and manage data. While some languages have just a few types of data structures to manage collections of data, Ruby offers numerous options, such as Arrays and Hashes, providing flexibility and efficiency in different scenarios. This makes it a popular choice for businesses looking to hire Ruby developers. However, the capabilities of Ruby extend far beyond these fundamental structures.
This blog will explore how to work with data in Ruby, providing key insights that could prove useful for those looking to hire Ruby developers or for programmers wishing to enhance their skills, focusing on arrays and hashes, then delving into more advanced options.
Arrays in Ruby
Ruby arrays are ordered, integer-indexed collections of any object. They allow you to store an ordered list of elements, which can be objects of any type or class.
```ruby # Array Initialization array = [1, 'two', 3.0, [4, 5], {six: 6}] # Accessing Array Elements puts array[1] # => 'two' puts array[3][0] # => 4 # Modifying an Array array << 'seven' # => [1, 'two', 3.0, [4, 5], {six: 6}, 'seven'] array.pop # => 'seven' array.shift # => 1
As demonstrated above, array operations in Ruby are simple and intuitive. The power of Ruby arrays is further increased by numerous array methods provided by Ruby, such as `each`, `map`, `select`, `reject`, `reduce`, and many more.
Hashes in Ruby
While arrays use an integer index, hashes in Ruby are collections of unique keys and their values, commonly referred to as key-value pairs. Keys can be any object type.
```ruby # Hash Initialization hash = {'a' => 1, 'b' => 2, 'c' => 3} # Accessing Hash Values puts hash['a'] # => 1 # Modifying a Hash hash['d'] = 4 # => {'a' => 1, 'b' => 2, 'c' => 3, 'd' => 4} hash.delete('a') # => {'b' => 2, 'c' => 3, 'd' => 4}
Ruby hashes are particularly useful when you want to associate specific values with specific keys, and when the keys are not simple integers.
Arrays and Hashes: A Powerful Combination
Both arrays and hashes are incredibly powerful in their own right, but when combined, they provide additional flexibility and functionality.
```ruby students = [ {'name' => 'Alice', 'age' => 20, 'major' => 'Computer Science'}, {'name' => 'Bob', 'age' => 21, 'major' => 'Physics'} ] puts students[0]['name'] # => 'Alice'
In the above example, we have an array of hashes, each hash representing a student. This structure allows for a complex and highly flexible representation of data.
Beyond Arrays and Hashes
Ruby provides numerous other ways to handle and manage collections of data.
Sets
A Set is similar to an Array, but it enforces uniqueness among its elements and provides efficient set operations like union, intersection, and difference.
```ruby require 'set' s1 = Set.new [1, 2, 3, 4] s2 = Set.new [3, 4, 5, 6] puts s1.union(s2) # => #<Set: {1, 2, 3, 4, 5, 6}> puts s1.intersection(s2) # => #<Set: {3, 4}>
Structs
Structs in Ruby are convenient and flexible data structures. They’re essentially small, ad-hoc bundles of attributes with their associated values.
```ruby Student = Struct.new(:name, :age, :major) s = Student.new('Alice', 20, 'Computer Science') puts s.name # => 'Alice'
OpenStructs
OpenStructs are similar to structs, but they don’t have a fixed schema. They allow you to set attributes on an instance and automatically create accessor methods for these attributes.
```ruby require 'ostruct' student = OpenStruct.new student.name = 'Alice' student.age = 20 puts student.name # => 'Alice'
Conclusion
Ruby provides an array of options (pun intended) for handling and managing data. The fundamental structures like Arrays and Hashes are incredibly powerful and flexible, and when combined, can represent complex datasets in intuitive and easy-to-manipulate ways. But the Ruby world extends far beyond these foundations, with structures like Sets, Structs, and OpenStructs providing unique functionality for different scenarios.
Working with data in Ruby is a rich and rewarding endeavor, a fact that is well-acknowledged by businesses aiming to hire Ruby developers. The variety of tools and structures available, coupled with Ruby’s inherent object-oriented nature and its commitment to programmer happiness, makes it an attractive choice. As you continue to explore and work with data in Ruby, you’ll discover that the language’s depth, power, and flexibility can handle virtually any data task you throw at it. Therefore, it’s no surprise that companies looking to leverage these strengths often opt to hire Ruby developers.
Table of Contents
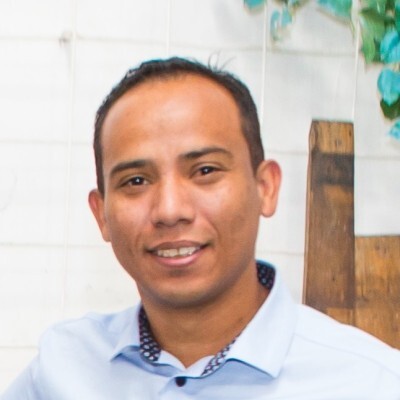
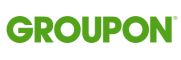