What is the difference between ‘map’ and ‘each’ in Ruby?
In Ruby, both the `map` and `each` methods are used for iterating over collections, such as arrays and hashes, and performing actions on their elements. However, they serve different purposes and have distinct behaviors:
- `each` Method:
– Purpose: The primary purpose of the `each` method is to iterate over a collection and perform an action on each element. It is used for its side effects, meaning it doesn’t change the original collection but instead executes a block of code for each element.
– Return Value: The `each` method always returns the original collection itself, whether it’s an array or a hash. It doesn’t modify the collection or create a new one.
Example:
```ruby numbers = [1, 2, 3, 4, 5] numbers.each { |num| puts num * 2 } ```
In this example, `each` iterates over the `numbers` array, doubling each element, but it doesn’t change the array itself.
- `map` Method:
– Purpose: The primary purpose of the `map` method is to iterate over a collection and transform each element based on the provided block of code. It is used when you want to create a new array with the modified elements from the original collection.
– Return Value: The `map` method returns a new array containing the results of applying the block of code to each element of the original collection, effectively creating a new collection.
Example:
```ruby numbers = [1, 2, 3, 4, 5] doubled_numbers = numbers.map { |num| num * 2 } ```
In this example, `map` iterates over the `numbers` array, doubling each element, and stores the results in a new array called `doubled_numbers`.
The main difference between `each` and `map` is in their purpose and return values. `each` is primarily for iteration and side effects, while `map` is used for transformation and creating a new collection. Depending on your specific task, you would choose one over the other to achieve the desired result.
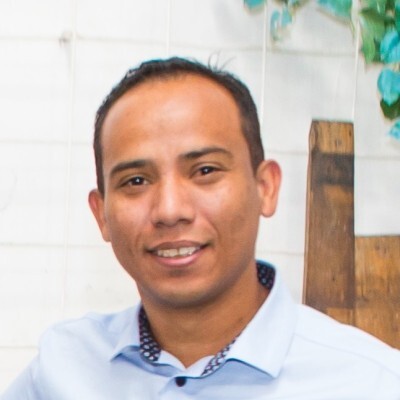
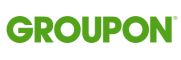