Mastering Error Handling in Ruby: Exception Handling 101
When diving into the vast world of Ruby, a crucial topic you’ll undoubtedly encounter is error handling. Effective error handling in any programming language is a fundamental skill every developer should possess. When it comes to Ruby, the language offers a solid error-handling mechanism through its system of exceptions.
This article will delve into the principles of exception handling in Ruby, including its structure, types of exceptions, how to handle them, and best practices.
Understanding Exceptions in Ruby
Exceptions are Ruby’s way of managing errors or unusual situations that may arise during the execution of a program. Think of an exception as an instance of the Exception class or any of its subclasses. When an error occurs, an exception is raised (or ‘thrown’). If the raised exception is not rescued (or ‘caught’), it may result in the termination of the program.
Ruby’s Exception Hierarchy
Understanding the hierarchy of exceptions in Ruby is vital to handling them effectively, whether you’re working alone or collaborating with a team of expert Ruby developers. The Exception class, the root of Ruby’s exception hierarchy, is the progenitor for all other exception classes, including system exit and signal exceptions. Most of the exceptions you’ll grapple with, especially when working on complex projects that often require you to hire Ruby developers, are subclasses of the StandardError class, a direct subclass of Exception. These encompass common exceptions like NoMethodError, ArgumentError, and RuntimeError.
Here’s a simplified version of the hierarchy:
Exception
– NoMemoryError
– ScriptError
– StandardError
– ArgumentError
– IOError
– NameError
– RuntimeError
etc.
Basic Exception Handling
Ruby uses the `begin`, `rescue`, and `end` keywords for basic exception handling. In a `begin` block, you place the code that might raise an exception. If an exception is raised, the flow of control goes to the `rescue` block, where you handle the exception.
Let’s look at a simple example:
```ruby begin # risky code 1/0 rescue puts "An error occurred!" end
When you run this, instead of the program crashing due to a division by zero error, it moves to the `rescue` block and prints “An error occurred!” to the console.
Specifying Exception Types
In most cases, you’ll want to specify the type of exceptions your `rescue` block should handle. This is done by appending the `rescue` keyword with the name of the exception class. Multiple classes can be specified, separated by commas.
```ruby begin # risky code 1/0 rescue ZeroDivisionError puts "Tried to divide by zero!" end
In this code, the `rescue` block only rescues `ZeroDivisionError` exceptions.
Multiple Rescue Blocks
Different exceptions may require different treatment. You can cater to this by having multiple `rescue` blocks in your exception handling structure. Ruby will attempt to match the raised exception with the exceptions in your `rescue` blocks in the order they’re defined.
```ruby begin # risky code 1/0 rescue ZeroDivisionError puts "Tried to divide by zero!" rescue StandardError puts "Some other error occurred!" end
## Using else and ensure
Ruby provides two additional keywords for exception handling: `else` and `ensure`. Code in the `else` block is executed if the `begin` block didn’t raise any exceptions. The `ensure` block contains code that will be executed regardless of whether an exception was raised.
# code to run in all cases end
Raising Exceptions
Sometimes, you may want to raise your own exceptions. You can do this using the `raise` keyword followed by an optional string which will become the error message.
```ruby begin raise "An error occurred!" rescue RuntimeError => e puts e.message end
The `raise` method, when used without any arguments within a `rescue` block, re-raises the exception being handled.
Exception Handling Best Practices
- Don’t Overuse Exceptions: Exceptions are meant to handle exceptional situations, not for regular flow control. Using exceptions for common situations can make your code harder to read and debug.
- Be Specific with Rescue: Try to avoid rescuing `StandardError` or `Exception` without a good reason. Being specific about what you’re rescuing allows your program to respond correctly to different types of errors.
- Keep Rescue Blocks Small: The larger the block, the more potential there is for an unexpected error. Keeping `rescue` blocks small helps isolate where errors occur and makes the code easier to maintain.
- Include Error Information: When raising your own exceptions, include relevant information in the error message to help with debugging.
Conclusion
Understanding and mastering exception handling is an essential aspect of Ruby programming. It not only helps prevent unexpected program terminations but also improves code readability and maintainability. This guide provided a thorough introduction to error handling in Ruby, covering topics from the exception hierarchy to raising and handling exceptions effectively.
However, there’s still much more to learn and implement when it comes to creating robust and resilient Ruby applications. If you are in need of professional help, hiring Ruby developers can be a great solution. Skilled Ruby developers bring extensive experience and can help ensure your applications are not only error-free but also optimized and efficient. Happy coding, and remember, Ruby exceptions are your friends, not foes!
Table of Contents
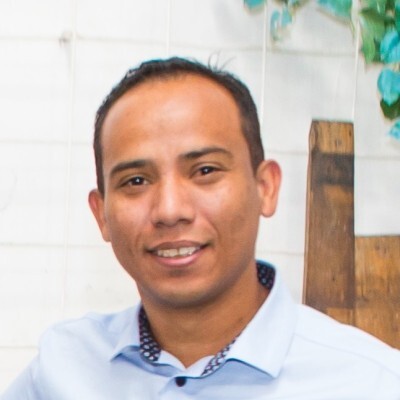
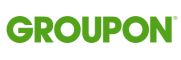