Ruby for Natural Language Generation: Generating Text and Content
In the realm of technology, the ability to generate human-like text and content programmatically has become increasingly important. Whether it’s for chatbots, content creation, or data analysis reports, Natural Language Generation (NLG) plays a pivotal role. One language that has garnered attention for its simplicity and versatility in NLG is Ruby. In this blog, we’ll delve into how Ruby can be used for NLG, exploring techniques, tools, and providing code samples to demonstrate its capabilities.
Table of Contents
1. Understanding Natural Language Generation (NLG)
Before we dive into the specifics of using Ruby for NLG, let’s briefly understand what NLG is all about. NLG is a subfield of artificial intelligence (AI) and computational linguistics that focuses on generating human-like language from structured data. This technology enables computers to transform data into coherent, contextually relevant narratives.
NLG finds applications in various domains, such as:
- Content Creation: Automating the generation of articles, product descriptions, and other written content.
- Data Analysis: Creating textual summaries and explanations of complex data sets.
- Chatbots and Virtual Assistants: Producing responses that feel natural and human-like.
- Personalization: Tailoring messages and recommendations to individual users.
2. Why Ruby for NLG?
Ruby is a dynamic, object-oriented programming language known for its elegant syntax and developer-friendly environment. It offers a wide range of libraries and gems that facilitate various tasks, including NLG. The simplicity and expressiveness of Ruby make it an excellent choice for rapid development and experimentation.
3. NLG Techniques and Approaches
3.1. Template-Based NLG
Template-based NLG is one of the simplest approaches to generating text. It involves creating templates that contain placeholders for dynamic content. These placeholders are then replaced with specific data to generate the final text. Ruby’s string interpolation and formatting capabilities make it well-suited for this approach.
ruby # Template-based NLG example in Ruby product_name = "Smartphone" price = "$499" description_template = "Introducing our latest #{product_name} for just #{price}!" puts description_template
3.2. Markov Chains
Markov chains are a probabilistic model that can be used for text generation. They analyze a given input text and create a model based on the probabilities of transitioning from one word to another. This approach can produce text that resembles the input data in terms of style and vocabulary. Ruby’s ease of handling text manipulation and probability calculations makes it a good fit for implementing Markov chains.
ruby # Markov chain text generation example in Ruby def generate_text(markov_model, length) current_word = markov_model.keys.sample generated_text = [current_word] (length - 1).times do next_word = markov_model[current_word].sample generated_text << next_word current_word = next_word end generated_text.join(" ") end # Example usage markov_model = { "I" => ["am", "have", "like"], "am" => ["happy", "excited"], "have" => ["a", "an"], # ... } generated_text = generate_text(markov_model, 10) puts generated_text
3.3. Neural Networks
Neural networks, particularly Recurrent Neural Networks (RNNs) and Long Short-Term Memory networks (LSTMs), have demonstrated remarkable capabilities in text generation. These networks learn patterns from large text corpora and can generate text that flows naturally. While implementing neural networks for NLG can be more complex, Ruby offers libraries like TensorFlow.rb and Keras.rb that allow integration with neural network models.
ruby # Using TensorFlow.rb for text generation require 'tensor_stream' require 'tensor_stream/tensorflow' model = TensorStream::Layers::Sequential.new model.add(TensorStream::Layers::Embedding.new(input_dim: vocab_size, output_dim: embedding_dim, input_length: sequence_length)) model.add(TensorStream::Layers::LSTM.new(units: 256, return_sequences: true)) model.add(TensorStream::Layers::Dense.new(units: vocab_size)) # ... # Training the model and generating text
4. NLG Tools and Libraries in Ruby
Ruby’s open-source community has contributed various tools and libraries that simplify NLG tasks. Some noteworthy ones include:
4.1. Faker
Faker is a gem that generates fake data, including names, addresses, and text. While it’s primarily used for testing and development, it can also be employed creatively for NLG purposes. For instance, you can use it to simulate user-generated content or generate placeholder text.
ruby # Using Faker for generating fake text require 'faker' 10.times do puts Faker::Lorem.sentence end
4.2. Sentence Generator
SentenceGenerator is a gem specifically designed for generating sentences. It allows you to create grammatically correct sentences by combining different sentence parts.
ruby # Using SentenceGenerator to create sentences require 'sentence_generator' generator = SentenceGenerator::Generator.new generator.subject = ['I', 'You', 'He'] generator.verb = ['like', 'enjoy', 'dislike'] generator.object = ['apples', 'bananas', 'oranges'] 5.times do puts generator.generate end
5. Putting It All Together
Ruby’s versatility and the available tools make it an excellent choice for NLG. Whether you’re using template-based approaches, Markov chains, or diving into neural networks, Ruby provides the flexibility and ease of use necessary for text generation tasks. By harnessing the power of Ruby and its NLG capabilities, you can automate content creation, enhance user interactions, and explore creative possibilities.
In this blog, we’ve only scratched the surface of what Ruby can offer in the realm of NLG. As you delve deeper into the world of text generation, you’ll uncover more techniques, tools, and possibilities that Ruby brings to the table. So go ahead, experiment, and let Ruby empower you to craft compelling narratives through code.
Conclusion
Natural Language Generation is a captivating field that blends linguistics and technology, enabling computers to generate human-like text and content. Ruby’s simplicity and expressive syntax make it an excellent companion for NLG tasks. Whether you’re a content creator, a data scientist, or a developer, exploring Ruby’s NLG capabilities can open up new avenues for efficiency and creativity. So why not dive in and start crafting your own AI-generated masterpieces with Ruby?
Table of Contents
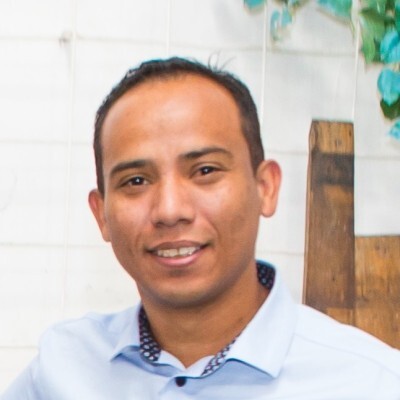
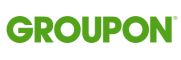