Exploring Ruby’s Networking Capabilities: Sockets and Protocols
Networking is a fundamental aspect of modern software development, enabling applications to communicate and exchange data over various networks. Ruby, a versatile and expressive programming language, offers robust networking capabilities through its support for sockets and protocols. Whether you’re building a client-server application, implementing network protocols, or simply exploring network communication, Ruby provides a rich set of tools to make your life easier.
In this blog post, we will delve into Ruby’s networking capabilities, focusing specifically on sockets and protocols. We’ll explore the basics of networking, examine how sockets work, and learn about the most common protocols used in network communication. Additionally, we’ll provide code samples and practical examples to demonstrate how to leverage these features in your Ruby applications.
Understanding Networking Basics
Before we dive into the specifics of Ruby’s networking capabilities, it’s essential to understand some networking fundamentals. At its core, networking involves establishing connections between different devices, allowing them to communicate with each other. These devices can be computers, servers, IoT devices, or any other network-enabled device.
When devices communicate over a network, they rely on protocols, which are a set of rules and guidelines that define how data is formatted, transmitted, and received. Some well-known protocols include HTTP, TCP, UDP, and FTP, among others. These protocols ensure that data is exchanged reliably and efficiently.
Ruby’s Socket Library
Ruby’s Socket library is the gateway to networking in Ruby. It provides a set of classes and methods that allow you to work with sockets, establish connections, and perform network communication. The Socket library is part of Ruby’s standard library, meaning you don’t need to install any additional gems to get started.
To begin working with sockets in Ruby, you first need to require the socket library:
ruby require 'socket'
With the Socket library loaded, you can create sockets, establish connections, and perform network communication.
TCP Socket Communication
The Transmission Control Protocol (TCP) is one of the most widely used protocols for reliable network communication. It ensures that data sent over a network arrives intact and in the correct order.
Let’s take a look at a simple example that demonstrates how to establish a TCP connection between a client and server in Ruby:
ruby # Server side server = TCPServer.new(2000) loop do client = server.accept client.puts "Hello, client!" client.close end # Client side socket = TCPSocket.new('localhost', 2000) response = socket.gets puts response socket.close
In this example, the server creates a TCP server socket and listens on port 2000. When a client connects, it sends a “Hello, client!” message and closes the connection. On the client side, a TCP client socket is created and connects to the server. The client then reads the server’s response and closes the connection.
UDP Socket Communication
Unlike TCP, the User Datagram Protocol (UDP) provides a connectionless communication model. UDP is typically used when low latency and efficiency are more important than reliability.
Here’s a simple example that demonstrates how to use UDP sockets in Ruby:
ruby # Server side socket = UDPSocket.new socket.bind('localhost', 2000) data, addr = socket.recvfrom(1024) puts "Received: #{data} from #{addr[2]}" # Client side socket = UDPSocket.new socket.send("Hello, server!", 0, 'localhost', 2000) socket.close
In this example, the server creates a UDP socket and binds it to port 2000. It waits to receive a message from a client and then prints the received data and the client’s address. On the client side, a UDP socket is created, and a message is sent to the server’s address and port.
Working with Protocols
In addition to sockets, Ruby provides built-in support for various protocols that simplify working with higher-level network communication.
For example, the Net::HTTP library allows you to perform HTTP requests and interact with web services:
ruby require 'net/http' uri = URI('https://api.example.com') response = Net::HTTP.get_response(uri) puts response.body if response.is_a?(Net::HTTPSuccess)
In this example, we require the net/http library and create a URI object representing the target URL. We then use Net::HTTP.get_response to send an HTTP GET request and obtain the response. Finally, we print the response body if the response was successful.
Conclusion
Ruby’s networking capabilities, including its support for sockets and protocols, provide developers with powerful tools for building networked applications. By understanding the basics of networking, utilizing Ruby’s Socket library, and leveraging higher-level protocols, you can create robust and efficient network communication in your Ruby applications.
In this blog post, we explored the fundamentals of networking, demonstrated how to work with TCP and UDP sockets, and showcased the usage of protocols like HTTP. Armed with this knowledge, you can confidently tackle a wide range of networking tasks and build scalable and reliable networked applications using Ruby.
Table of Contents
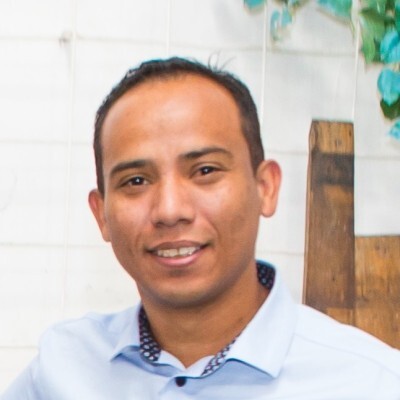
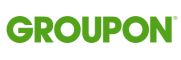